Update on this, I've found this helpful article:
https://www.mql5.com/en/articles/488
To keep it short, it seems the threshold must be 100
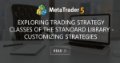
Exploring Trading Strategy Classes of the Standard Library - Customizing Strategies
- www.mql5.com
In this article we are going to show how to explore the Standard Library of Trading Strategy Classes and how to add Custom Strategies and Filters/Signals using the Patterns-and-Models logic of the MQL5 Wizard. In the end you will be able easily add your own strategies using MetaTrader 5 standard indicators, and MQL5 Wizard will create a clean and powerful code and fully functional Expert Advisor.
Actually this is a better post for future people who would like to find out how to find the SIGNIFICANCES
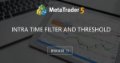
Intra time filter and threshold
- 2019.07.06
- pbzf
- www.mql5.com
hello, I am working on a EA by using mql5 wizard generator. I did not really understand how the signal mechanism module and intra time filter works...

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
I just made a self generated EA code for testing. Even after reading the documentation, I am confused how it works, especially on where the value "significance" is based.
Anyway, I just want to ask if this code opens order only if all of the conditions are met, or it opens order even only one condition is met? I tried testing it but I can't seem to view exactly so I want to verify by code.
If it does open order even one condition is met, how do I make it open an order only if all conditions are met.