A batch file is a text file, not an executable. You can't run it.
Try file="cmd.exe" and parameters="/c "+batchfile.
I couldnt run batch file.
returned error code is 2. Probably it coudnt find script file but it is in Files folder and it was working when I run it on comand
So I dont know how it works on MT5
To run a batch file in MQL5, you can use the WinExec() function, which is available in MetaTrader 5's MQL5 language. This function allows you to execute external applications, including batch files. Here's a simple example:
//+------------------------------------------------------------------+ //| RunBatch.mq5| //| Copyright 2024, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property strict //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { string batchFilePath = "C:\\path\\to\\your\\batchfile.bat"; int result = WinExec(batchFilePath, SW_HIDE); // SW_HIDE to hide the command prompt window, SW_SHOW to show it if(result <= 31) { Print("Error executing batch file! Error code: ", GetLastError()); } else { Print("Batch file executed successfully!"); } return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { } //+------------------------------------------------------------------+
Replace "C:\\path\\to\\your\\batchfile.bat" with the actual path to your batch file. You can change SW_HIDE to SW_SHOW if you want to display the command prompt window while running the batch file.
Keep in mind that running external applications from within your trading platform can pose security risks, so make sure you trust the batch file you are executing and the source of the file.
And thats how you do it..
To run a batch file in MQL5, you can use the WinExec() function, which is available in MetaTrader 5's MQL5 language. This function allows you to execute external applications, including batch files. Here's a simple example:
Replace "C:\\path\\to\\your\\batchfile.bat" with the actual path to your batch file. You can change SW_HIDE to SW_SHOW if you want to display the command prompt window while running the batch file.
Keep in mind that running external applications from within your trading platform can pose security risks, so make sure you trust the batch file you are executing and the source of the file.
And thats how you do it..
And I got error code
#import "Shell32.dll" int WinExec( string CmdLine, int nCmdShow); #import int OnInit() { string file = TerminalInfoString(TERMINAL_DATA_PATH)+"MQL5\\Files\\batchfile.bat"; int result = WinExec(file,0); return(INIT_SUCCEEDED); }
How can I run batch files?
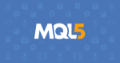
- www.mql5.com
WinExec is not MQL5 function. That is external function.
And I got error code
How can I run batch files?
Use the WinExec in the windows API.
Try this for Mql:
//+------------------------------------------------------------------+ //| RunBatch.mq5 | //| Copyright 2024, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property strict // Include necessary WinAPI declarations #import "shell32.dll" int ShellExecuteW(int hwnd, string lpOperation, string lpFile, string lpParameters, string lpDirectory, int nShowCmd); #import //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { // Specify the path to your batch file string batchFilePath = "C:\\Path\\To\\Your\\BatchFile.bat"; // Execute the batch file int result = ShellExecuteW(0, "open", batchFilePath, "", "", 1); if(result > 32) { Print("Batch file executed successfully."); } else { Print("Error executing batch file. Error code: ", result); } return INIT_SUCCEEDED; } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { // Place your deinitialization code here } //+------------------------------------------------------------------+
Replace "C:\\Path\\To\\Your\\BatchFile.bat" with the actual path to your batch file. This script will attempt to execute the specified batch file using ShellExecuteW() . If successful, it will print "Batch file executed successfully." to the Experts tab in MetaTrader's Terminal. If there's an error, it will print the error code.
Remember that when running external files or programs, especially batch files, ensure that they are safe and do not pose any security risks to your system.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I couldnt run batch file.
returned error code is 2. Probably it coudnt find script file but it is in Files folder and it was working when I run it on comand
So I dont know how it works on MT5