The problem is not with Point(), but with how you compare these values
if(close[i-1]==(open[i]+Point())) if(close[i-1]==(open[i])-Point())
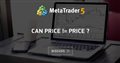
- 2011.12.08
- www.mql5.com
Just typed (didn't compile or run):
for(int i=limit; i<rates_total; i++) { BUYBuffer[i]=0.0; SELLBuffer[i]=0.0; double closeNorm = NormalizeDouble(close[i-1], Digits()); if(closeNorm==NormalizeDouble(open[i]+Point(), Digits())) BUYBuffer[i]=high[i]; //--- Bearish if(closeNorm==NormalizeDouble(open[i]-Point(), Digits()))//left SELLBuffer[i]=low[i]; }
This is the most primitive way. And this method is not the fastest.
You can Google something like "How to compare two float or double numbers".
I don't know why you didn't like this thread.
There's a ton of information in this post (and it's just one of 85 posts in this thread):
Forum on trading, automated trading systems and testing trading strategies
William Roeder, 2023.06.16 14:05
NormalizeDouble, It's use is usually wrong.
-
Floating point has an infinite number of decimals, it's you were not understanding floating point and that some numbers can't be represented exactly. (like 1/10.)
Double-precision floating-point format - Wikipedia, the free encyclopediaSee also The == operand. - MQL4 programming forum (2013)
-
Print out your values to the precision you want with DoubleToString - Conversion Functions - MQL4 Reference.
-
SL/TP (stops) need to be normalized to tick size (not Point) — code fails on non-currencies.
On 5Digit Broker Stops are only allowed to be placed on full pip values. How to find out in mql? - MQL4 programming forum #10 (2011)And abide by the limits Requirements and Limitations in Making Trades - Appendixes - MQL4 Tutorial and that requires understanding floating point equality Can price != price ? - MQL4 programming forum (2012)
-
Open price for pending orders need to be adjusted. On Currencies, Point == TickSize, so you will get the same answer, but it won't work on non-currencies. So do it right.
Trailing Bar Entry EA - MQL4 programming forum (2013)
Bid/Ask: (No Need) to use NormalizeDouble in OrderSend - MQL4 programming forum (2012) -
Lot size must also be adjusted to a multiple of LotStep and check against min and max. If that is not a power of 1/10 then NormalizeDouble is wrong. Do it right.
(MT4 2013)) (MT5 2022)) -
MathRound() and NormalizeDouble() are rounding in a different way. Make it explicit.
MT4:NormalizeDouble - MQL5 programming forum (2017)
How to Normalize - Expert Advisors and Automated Trading - MQL5 programming forum (2017) -
Prices you get from the terminal are already correct (normalized).
-
PIP, Point, or Tick are all different in general.
What is a TICK? - MQL4 programming forum (2014)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello,
I have a strange problem when I add Point() in code it causes buffers to disappear from candles for some candlesticks. When i remove point it works fine on all bar. How can i fix this?
When Point is added on code
When Point is removed from code