Guys, I have to use some functions of an native DLL.
It is implemented like this in C, I think. Notice the inner structs.
Here is the exposed struct;
In C it is called like this:
But I could not make it work in MQL5. Is it possible?
In MQL5 I could typdef the and expose just the libnative_ExportedSymbols structure only, for inner ones I could not find the syntax. Is it possible?
I am posting it again @Dominik Egert:
This is how it it's making it available in the build DLL:
Notice how the inner structs are referenced in C, is it possible to do it in Mql5? I tried guessing how it works in many ways, and I read lots of documentation about the #import clause but I could not make it work.
Thanks.
I am posting it again @Dominik Egert:
This is how it it's making it available in the build DLL:
Notice how the inner structs are referenced in C, is it possible to do it in Mql5? I tried guessing how it works in many ways, and I read lots of documentation about the #import clause but I could not make it work.
Thanks.
EDIT:
I have thought about this, and I think you need a helper DLL to actually convert the struct pointer to a native MQL struct...
Something along the lines of this (only typed, not tested):
typedef void (*funcptr_forIntegers)(char, short, int, long) typedef void (*funcptr_forFloats)(float, double); struct _libnative_ExportedSymbols { struct _root { struct _example { funcptr_forIntegers integer_func; funcptr_forFloats floats_func; } example; } root; } libnative_ExportedSymbols; #import "my_nasty_dll" ulong libnative_symbols(void); #import #import "helper_dll" void convert_structptr_to_struct(_libnative_ExportedSymbols&, ulong) #import void OnStart() { _libnative_ExportedSymbols mql_struct(); convert_structptr_to_struct(mql_struct, libnative_symbols()); };
EDIT:
I have thought about this, and I think you need a helper DLL to actually convert the struct pointer to a native MQL struct...
Something along the lines of this (only typed, not tested):
I understood. I was trying to avoid to have a extra dll in between, but it seems there is no other way.
If I cannot escape of having an extra DLL, I think it would be easier if in the helper one I just expose my methods without a nested structure. What do you think? Does it sound easier?
I understood. I was trying to avoid to have a extra dll in between, but it seems there is no other way.
If I cannot escape of having an extra DLL, I think it would be easier if in the helper one I just expose my methods without a nested structure. What do you think? Does it sound easier?
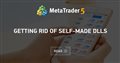
- www.mql5.com
The exposed ones will not change much. I will keep some sort of well defined "interfaces". Damn, I really need to solve it...

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Guys, I have to use some functions of an native DLL.
It is implemented like this in C, I think. Notice the inner structs.
Here is the exposed struct;
In C it is called like this:
But I could not make it work in MQL5. Is it possible?
In MQL5 I could typdef the and expose just the libnative_ExportedSymbols structure only, for inner ones I could not find the syntax. Is it possible?