Try this .
Theres the argument that "you removed the update charts 2nd check" but you also now know the ea closed the chart with that specific id.
What is the ea doing by the way ? You could use custom chart events to facilitate intrachart communication if they are running your tools .
#property strict long chartids[]; string syms[]={"USDJPY","CADJPY","GBPNZD"}; void OnInit(){ string txt=""; //======Open 3 charts============== for(int i=0;i<ArraySize(syms);i++){ long id=ChartOpen(syms[i],_Period); ObjectCreate(id,"mychart",OBJ_TEXT,0,0,0); } //======Update list of open charts= chartsupdate(); txt="After opening charts\n" + chartlist() +"\n"; //======Close 3 charts============== int from=ArraySize(chartids)-1; for(int i=from;i>=0;i--){ if(ChartClose(chartids[i])){ remove(i,chartids); } } //Sleep(500); //======Update list of open charts== //chartsupdate(); txt=txt + "After closing charts\n" + chartlist() +"\n"; Comment(txt); } void chartsupdate(){ long thisid=0; int x=0; ArrayResize(chartids,0); do{ thisid=ChartNext(thisid); if(thisid==-1)break; if(thisid==ChartID())continue; if(ObjectFind(thisid,"mychart")<0)continue; ArrayResize(chartids,x+1); chartids[x]=thisid; x++; if(x>99)break; } while(true); } string chartlist(){ string txt=""; for(int i=0;i<ArraySize(chartids);i++) txt=txt + (string)chartids[i] + " " +ChartSymbol(chartids[i]) +"\n"; return txt; } int find_id(long id,long &array[]){ for(int i=0;i<ArraySize(array);i++){ if(id==array[i]){ return(i); } } return(-1); } void remove(int i,long &array[]){ if(i<ArraySize(array)&&i>=0&&ArraySize(array)>0){ array[i]=array[ArraySize(array)-1]; ArrayResize(array,ArraySize(array)-1,0); } }
Try this .
void remove(int i,long &array[]){ if(i<ArraySize(array)&&i>=0&&ArraySize(array)>0){ array[i]=array[ArraySize(array)-1]; ArrayResize(array,ArraySize(array)-1,0); } }
Theres the argument that "you removed the update charts 2nd check" but you also now know the ea closed the chart with that specific id.
What is the ea doing by the way ? You could use custom chart events to facilitate intrachart communication if they are running your tools .
Thanks, it makes sense to do that.
I've been trying to write my EAs so they are not so dependent on processes within the EA, in this case updating the array from the terminal, rather than EA actions.
With my EA, I'm scanning symbols and periods with an indicator, and then opening a new chart with another EA to manage the trade, then closing it when in profit etc. But I'm thinking now, the new chart can close itself when required.
Cheers
Thanks, it makes sense to do that.
I've been trying to write my EAs so they are not so dependent on processes within the EA, in this case updating the array from the terminal, rather than EA actions.
With my EA, I'm scanning symbols and periods with an indicator, and then opening a new chart with another EA to manage the trade, then closing it when in profit etc. But I'm thinking now, the new chart can close itself when required.
Cheers
I see , you are trying to delegate tasks . You can study custom chart events in that case , it can be internal communication between charts .
Are you an android developer by the way?
My mql4 EA opens charts and closes them under various scenarios.
The problem I have is once a chart is closed, it is still showing as an open chart via ChartNext(..) etc. even though the chart is obviously closed.
For testing, the code below opens, then closes 3 charts, and lists the resulting charts.
Only if I include a Sleep(..) function - as below - does the code work ok.
WITHOUT Sleep command (wrong)
WITH Sleep command. (correct)
This is missing from the documentation: "The command is added to chart message queue and executed only after all previous commands have been processed."
It is there in MQL4 ChartOpen but missing from ChartClose documentation.
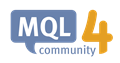
- docs.mql4.com
I see , you are trying to delegate tasks . You can study custom chart events in that case , it can be internal communication between charts .
Are you an android developer by the way?
Yes, I will look into custom charts; no I'm not an android developer, just a long time amateur mql4 programmer...;)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
My mql4 EA opens charts and closes them under various scenarios.
The problem I have is once a chart is closed, it is still showing as an open chart via ChartNext(..) etc. even though the chart is obviously closed.
For testing, the code below opens, then closes 3 charts, and lists the resulting charts.
Only if I include a Sleep(..) function - as below - does the code work ok.
WITHOUT Sleep command (wrong)
WITH Sleep command. (correct)