Here is an example: https://www.mql5.com/en/code/23886
It's for mq4 but just study the calculation.
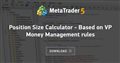
Position Size Calculator - Based on VP Money Management rules
- www.mql5.com
This indicator is based on the Money Management approach used by VP from nononsenseforex.com It uses ATR to calculate Stop Loss, Take Profit and Volume you should enter into a trade based on the percentage of your Equity you want to risk.
aliasgari524:
Hello Guys , i want to Create a personal panel for trading ,Everything is fine
Except that the calculation of lot size according to ATR does not work correctly.
Lot size calculations should be according to account balance and atr...
Hello , why are you multiplying by the tick size here ?
double atrValue = atr * tickSize; //
You are looking for "how many points the atr is" there right ?
If yes then divide by tickSize.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hello Guys , i want to Create a personal panel for trading ,Everything is fine
Except that the calculation of lot size according to ATR does not work correctly.
Lot size calculations should be according to account balance and atr...