So you are already doing it. What is the problem ?
Scott David Maclean #:
This code doesn't work for LR Correlation. It just returns a value of 0 even if it's greater than 0.
Is this related to this library from the Codebase ?
Then ask to the author.
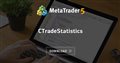
CTradeStatistics
- www.mql5.com
Class for the calculation of the ENUM_STATISTICS enumeration parameters

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hey guys, wondering if anyone has any clue how to integrate the LR Correlation statistic into the ontester using CTradeStatistics. Here's my code: