Please don't post randomly in any section. Your question is not related to the section you posted.
MT4/mql4 has it's own section on the forum.
Your topic has been moved to correct section, please don't create another duplicate topic.
Please don't post randomly in any section. Your question is not related to the section you posted.
MT4/mql4 has it's own section on the forum.
Your topic has been moved to correct section, please don't create another duplicate topic.
My Excuses!
ExtAoBuffer[i]=iMA(NULL,60,InpFastSMA,0,MODE_SMA,PRICE_CLOSE,i*24-8)-iMA(NULL,60,InpSlowSMA,0,MODE_SMA,PRICE_CLOSE,i*24-8);
-
Do not hard code numbers. Use the proper enumerations.
-
i*24-8 A) assumes your chart's period (D1) vs PERIOD_H1. Don't assume. B) Assumes that all bars exist. They don't. C) trying to read eight (8) hours into the future. Use iBarShift to get the shift of other timeframes.
-
On MT4: Unless the current chart is that specific symbol(s)/TF(s) referenced, you must handle 4066/4073 errors before accessing candle/indicator values.
Download history in MQL4 EA - MQL4 programming forum - Page 3 #26.4 (2019)
-
Do not hard code numbers. Use the proper enumerations.
-
i*24-8 A) assumes your chart's period (D1) vs PERIOD_H1. Don't assume. B) Assumes that all bars exist. They don't. C) trying to read eight (8) hours into the future. Use iBarShift to get the shift of other timeframes.
-
On MT4: Unless the current chart is that specific symbol(s)/TF(s) referenced, you must handle 4066/4073 errors before accessing candle/indicator values.
Download history in MQL4 EA - MQL4 programming forum - Page 3 #26.4 (2019)
Thank you William for your reply!
I have the following code, which doesn't seem to work. I have made a couple of mistakes I suppose...
for(i=0; i<limit; i++) { // Thank you mr William for your help so far! int shift = iBarShift(_Symbol,PERIOD_M5,iTime(_Symbol,PERIOD_M30,i),false); Comment("Shift: " + shift); // Shift seems to give the right shift value // Fill the buffer with the right value ExtAoBuffer[i]=iMA(NULL,PERIOD_M5,InpFastSMA,0,MODE_SMA,PRICE_CLOSE,i-shift)- iMA(NULL,PERIOD_M5,InpSlowSMA,0,MODE_SMA,PRICE_CLOSE,i-shift); }
I seem to get the right shift, not completely sure, but it seems like it. I get the right value of the AO on (for example) the 30 minute timeframe, from the last bar of the 5 minute timeframe. But this only works on the last bar, see attached pictures to see the results.
I've not tried to implement the error handling yet, because I am still struggling with the basics. I will try to implement this when the basic indicator is working.
I hope you can help me with this problem. I've always been struggling with indicators because of problems (lack of knowledge) like this.
Thanks in advance!
Daan
Is there anyone who might be able to help me further?
I am only trading EURUSD, so does that mean I won't have to handle these 4066/4073 errors?
Thanks in advance!
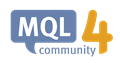
- docs.mql4.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi!
I am struggling to make a simple indicator, probably by the lack of coding experience. My excuses for my silly mistakes!
I am trying to get a indicator, similar to the AO, to show a bar of a different timeframe. So for example: I have the chart on a daily timeframe. But I want the indicator to show the 8th bar of the hourly timeframe on the daily timeframe.
I've tried the following code:
It kinda works, or atleast shows the right thing sometimes... See the pictures below:
It works at times, but it wont work in a backtest for example. Or whenever you zoom out too much...
What the indicator should do:
Show x bar of a lower timeframe on a higher timeframe. So for example the 8th bar of the hourly timeframe within the 24 bars that are in one daily bar. This means that there is a quite choppy indicator that on the daily timeframe.
Thanks in advance!
Daan