- Optimization on the mt4 platform, need help running it.
- How to make All "Open" buy trades with same tp
- trouble with OrderClose()
Hello! Trying to make a trade panel, started to read the documentation and already figured out ( kind of) how to make buttons and basicaly objects with code. One thing I cant find.. there is this box where you enter different values, and from where order function will take the data for SL, TP, SIZE or any other ... kindly point me to the correct direction. Thanks
If you started your journey in the Panels and Dialogs library , the object you are seeking is this :
Otherwise if you started in the objects section the object you are seeking is this :
☕️
(if you want to venture into no man's land though , you can take a peek at this CCanvas - Custom Graphics - Standard Library - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5)
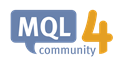
- docs.mql4.com
If you started your journey in the Panels and Dialogs library , the object you are seeking is this :
Otherwise if you started in the objects section the object you are seeking is this :
☕️
(if you want to venture into no man's land though , you can take a peek at this CCanvas - Custom Graphics - Standard Library - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5)
Thanks! Another question. If I use ObjectCreate() function and all the other parameters for creating the button, button can be pressed and depressed by click. If I create the button via classes, the button which is created, once is pressed, is returning to the original state, it does not remain pressed. What property should I use to maintain the button state once is clicked ?
Thanks! Another question. If I use ObjectCreate() function and all the other parameters for creating the button, button can be pressed and depressed by click. If I create the button via classes, the button which is created, once is pressed, is returning to the original state, it does not remain pressed. What property should I use to maintain the button state once is clicked ?
OBJPROP_STATE is the property , false means not clicked if recall .
Cant make it stay pressed or depressed. Keeps changeing back.
This is my attempt
#include <Controls\Dialog.mqh> #include <Controls\Button.mqh> #include <Controls\Label.mqh> #include <Controls\Edit.mqh> //+------------------------------------------------------------------+ //| CLASSES | //+------------------------------------------------------------------+ CAppDialog MyDialog; CButton BuyButton,SellButton,CloseAllButton,PartialCloseButton,AutoLotSizeButton; CEdit SetStopLossButton,LotSizeButton; //+------------------------------------------------------------------+ //| INPUTS | //+------------------------------------------------------------------+ string InpPanelName ="Trade Panel"; string InpFont ="Arial Bold"; int InpFontSize = 9; string InpSellButton ="SellButton"; string InpBuyButton ="BuyButton"; int panelwidth =50,panelheight=45; int AppDialogTop=50,AppDialogLeft=30,AppDialogWidth=410,AppDialogHeight=350; int BuyButtonTop=0,BuyButtonLeft=0,BuyButtonWidth=80,BuyButtonHeight=100; //+------------------------------------------------------------------+ //| VARIABLES | //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- MyDialog.Create(0,InpPanelName,0,AppDialogTop,AppDialogLeft,AppDialogWidth+AppDialogLeft,AppDialogTop+AppDialogHeight); BuyButton.Create(0,InpBuyButton,0,BuyButtonLeft,BuyButtonTop,BuyButtonLeft+BuyButtonWidth,BuyButtonTop+BuyButtonHeight); MyDialog.Add(BuyButton); BuyButton.ColorBackground(clrYellowGreen); BuyButton.Text("BUY"); SellButton.Create(0,InpSellButton,0,BuyButtonLeft+300,BuyButtonTop,BuyButtonLeft+BuyButtonWidth+300,BuyButtonTop+BuyButtonHeight); MyDialog.Add(SellButton); SellButton.ColorBackground(clrRed); SellButton.Text("SELL"); ObjectSetInteger(0,InpSellButton,OBJPROP_STATE,false); MyDialog.Run(); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- MyDialog.Destroy(reason); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- } //+------------------------------------------------------------------+ //| ChartEvent function | //+------------------------------------------------------------------+ void OnChartEvent(const int id, const long &lparam, const double &dparam, const string &sparam) { //--- MyDialog.ChartEvent(id,lparam,dparam,sparam); // ObjectSetInteger(0,InpSellButton,OBJPROP_STATE,0); if(ObjectGetInteger(0,InpSellButton,OBJPROP_STATE)==true) SellButton.ColorBackground(clrYellow); else if(ObjectGetInteger(0,InpSellButton,OBJPROP_STATE)==false) SellButton.ColorBackground(clrRed); }
Ow i answered the other way around sorry
The property is
SellButton.Pressed();
But it won't do anything , you need a switch which controls the state like this :
#property strict #include <Controls\Dialog.mqh> #include <Controls\Button.mqh> #include <Controls\Label.mqh> #include <Controls\Edit.mqh> bool SELL_SWITCH=false; //+------------------------------------------------------------------+ //| CLASSES | //+------------------------------------------------------------------+ CAppDialog MyDialog; CButton BuyButton,SellButton,CloseAllButton,PartialCloseButton,AutoLotSizeButton; CEdit SetStopLossButton,LotSizeButton; //+------------------------------------------------------------------+ //| INPUTS | //+------------------------------------------------------------------+ string InpPanelName ="Trade Panel"; string InpFont ="Arial Bold"; int InpFontSize = 9; string InpSellButton ="SellButton"; string InpBuyButton ="BuyButton"; int panelwidth =50,panelheight=45; int AppDialogTop=50,AppDialogLeft=30,AppDialogWidth=410,AppDialogHeight=350; int BuyButtonTop=0,BuyButtonLeft=0,BuyButtonWidth=80,BuyButtonHeight=100; //+------------------------------------------------------------------+ //| VARIABLES | //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- MyDialog.Create(0,InpPanelName,0,AppDialogTop,AppDialogLeft,AppDialogWidth+AppDialogLeft,AppDialogTop+AppDialogHeight); BuyButton.Create(0,InpBuyButton,0,BuyButtonLeft,BuyButtonTop,BuyButtonLeft+BuyButtonWidth,BuyButtonTop+BuyButtonHeight); MyDialog.Add(BuyButton); BuyButton.ColorBackground(clrYellowGreen); BuyButton.Text("BUY"); SellButton.Create(0,InpSellButton,0,BuyButtonLeft+300,BuyButtonTop,BuyButtonLeft+BuyButtonWidth+300,BuyButtonTop+BuyButtonHeight); MyDialog.Add(SellButton); SellButton.ColorBackground(clrRed); SellButton.Text("SELL"); MyDialog.Run(); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- MyDialog.Destroy(reason); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- } //+------------------------------------------------------------------+ //| ChartEvent function | //+------------------------------------------------------------------+ void OnChartEvent(const int id, const long &lparam, const double &dparam, const string &sparam) { //--- MyDialog.ChartEvent(id,lparam,dparam,sparam); //when the button is pressed you flip the switch if(SellButton.Pressed()){ SELL_SWITCH=!SELL_SWITCH; //and based on that change the color if(SELL_SWITCH){SellButton.ColorBackground(clrYellow);} else{SellButton.ColorBackground(clrRed);} } }
Ow i answered the other way around sorry
The property is
But it won't do anything , you need a switch which controls the state like this :
OK! Thanks! I ll try like that. This is more like I use the button to turn On/Off a switch , and the position of the switch will trigger other actions. There is no other way right? Cause if I create the Button with ObjectCreate() , as you said, with OPJPROP_STATE I can use position of the button to trigger other actions
Yeah the difference between the Dialogs Classes and the ObjectCreate() is :
In the classes the Pressed() indicates that it was pressed and you can do stuff in the event handeler , it fires once.
In the ObjectCreate it indicates its state changed and you have to handle it , and the state , the state stays after click.
Yeah the difference between the Dialogs Classes and the ObjectCreate() is :
In the classes the Pressed() indicates that it was pressed and you can do stuff in the event handeler , it fires once.
In the ObjectCreate it indicates its state changed and you have to handle it , and the state , the state stays after click.
Hi guys! I hav' another question:
I have a button on a dialog box, and I want to Use Button.Pressed() to detect when this button is pressed, and this will trigger an action. The thing is that CHARTEVENT_CLICK is also triggered, so another action is also triggered in the same time. How can I press that button without CHARTEVENTCLICK to be triggered? So just to understand better, I have seen this ideea in one trading assitant which I want to use. So I have this button which I press to allow trades, and then when I click somewhere on the chart, the order should open. And that last click will be actually the SL price also. Presently when I press the button, the order is also opened, so CHARTEVENT_CLICK is also triggered. This I want to avoid. Thanks
This is what I have know. I managed to make it work, with that _count param:
void OnChartEvent(const int id, const long &lparam, const double &dparam, const string &sparam) { if(TradeAllow.Pressed()) tradeallow=true; if(tradeallow ) { TradeAllow.ColorBackground(clrMagenta); TradeAllow.Text(" OPENING "); //--- MarketOrder if(id==CHARTEVENT_CLICK && _count>=1 ) { if(GetMousePosition(lparam,dparam,sparam)) trade=true; create_hLine("SL",mouseprice,clrAqua); } if(id==CHARTEVENT_CLICK) _count++; } }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use