As far as I know there is no "Set" variation for the indexing [] operator overloading, only for "Get".
In fact I remember reading a forum post of a work around several years back. I will see if can find it and reference it here.
I found one, but I'm not sure if this is the one read back then.
Forum on trading, automated trading systems and testing trading strategies
Custom indexing of an array / subscript operation overloading
nicholish en, 2018.06.23 20:01
You can't do assignment like that since you're returning an int, which is the equivalent to 5 = 7;
In order to implement assignment in the way you're attempting you'll need to return a reference to an object whose assignment operator has also been overloaded. ...which might look something like this.
#include <Arrays\List.mqh> class IntWrapper : public CObject { public: int custom_index; int num; void operator = (int n) { num = n; } }; class MyIntList : public CList { public: IntWrapper *operator[](int i) { IntWrapper *iw = this.GetFirstNode(); for(; CheckPointer(iw); iw=iw.Next()) if(iw.custom_index == i) return iw; iw = new IntWrapper(); this.Add(iw); iw.custom_index = i; return iw; } }; void OnStart() { MyIntList list; list[900] = 25; list[5] = 1900; Print(list[900].num); }

- 2016.09.17
- www.mql5.com
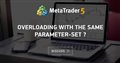
- 2016.04.04
- www.mql5.com
Fernando beat me to it as usual . There is no "void" operator that can be overloaded so you lose the get shortcut with this
template <typename X> class xElement{ public: X data; X get(){return(data);} void operator=(X value){data=value;} }; class CIntArray { //int array[]; public: xElement<int> array[]; public: public: void Set(int index, int value) { array[index]=value; } public: int Get(int index) { return array[index].get(); } public: xElement<int>* operator [] (int index) // Works { if(index>=0&&index<ArraySize(array)){ return(GetPointer(array[index]));} else{return(NULL);} } }; int OnInit() { //--- create timer CIntArray myarray; ArrayResize(myarray.array,1,0); myarray[0]=100; // Implementation missing //int retriever=myarray[0]; Print("myarray.array[0].data="+myarray.array[0].data); //--- return(INIT_SUCCEEDED); }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi all,
the example is generic, since I have no idea how to overload the indexed assignment ... does anyone know how to do?