Personally, I use the Init() function on objects in such cases.
First, an array is created, and then Init() function is called for all objects in the array, where all parameters can be set.
Initialization with separate function allows to re-initialize objects, and if you initialize in constructor, you can't re-initialize the object.
Personally, I use the Init() function on objects in such cases.
First, an array is created, and then Init() function is called for all objects in the array, where all parameters can be set.
The initialization with a separate function allows to re-initialize objects, and if you initialize it in the constructor, it's impossible to re-initialize the object.
Well, that's how I imagined it. Thanks !
One more point. It's better to create arrays of objects by means of a pointer. Otherwise you will get an array in stack memory, which is very small:
Strategy2 *pZ[]; if (ArrayResize(pZ, 10) != 10) { Alert("Memory allocation error"); return; } for (int i = 0; i < 10; ++i) { pZ[i] = new Strategy2("EURUSD"); if (CheckPointer(pZ) == POINTER_INVALID) { Alert("Class instantiation error"); return; } }
It's not a problem, and it's certainly not a potential problem. It's just the peculiarities of memory handling in MT. Here is a static array:
#define ARRAY_SIZE int(60000000) class Test { public: int nA; double fB; datetime dtC; Test(void) : nA(0) , fB(1.0) , dtC(__DATETIME__) { }; }; Test classTest[ARRAY_SIZE]; // 'classTest' - global variables section is too large void OnStart() { }
And here is a dynamic array:
#define ARRAY_SIZE int(60000000) class Test { public: int nA; double fB; datetime dtC; Test(void) : nA(0) , fB(1.0) , dtC(__DATETIME__) { }; }; Test *pClassTest[]; void OnStart() { if (ArrayResize(pClassTest, ARRAY_SIZE) != ARRAY_SIZE) { Alert("Not enought memory"); return; } for (int i = 0; i < ARRAY_SIZE; ++i) { pClassTest[i] = new Test(); if (CheckPointer(pClassTest[i]) == POINTER_INVALID) { Alert("Class instantiation error"); return; } } for (int i = 0; i < ARRAY_SIZE; ++i) delete pClassTest[i]; }
In this case, everything compiles and works.
It's not a problem, much less a potential problem. It's just the peculiarities of memory handling in MT. Here is a static array:
And here is a dynamic array:
In this case everything compiles and works.
What's the stack got to do with it? In the first case, you have tried to statically (at the compilation stage) allocate a large block of memory in a heap and the compiler justly kicked you in the forehead because it is not quite clear in reality whether you can allocate that much memory or not.
In the second case you are allocating a large chunk of memory already in runtime. And it is immediately clear whether you can allocate it or not, because the program is already working with a specific resource of the machine (memory).
And what do pointers have to do with it? Arrays in mql are of two types, predefined at compile time and dynamic. Not only pointers *, but usual class fields can point to dynamic arrays as well. So using pointers here is absolutely not justified.
s.e. Strange impression of the code, like pointers, classes, macros - with complete lack of understanding of what's going on.
It's not a problem, much less a potential problem. It's just the peculiarities of memory handling in MT. Here is a static array:
And here is a dynamic array:
In this case, everything compiles and works.
Wrong example, it's just a limitation of the compiler that doesn't say anything, the developers just decided so.
If there were two versions - one of them has a large static array and the other one has a small one and, lo and behold, when the program works, problems occur in one case, for instance, when recursively calling a function, while in the other case, under the same conditions, they don't. That's when you could draw a conclusion ... about the harm of freshly squeezed juice)))
What does the stack have to do with it? In the first case, you tried to statically (at the compilation stage) allocate a large block of memory in the heap and the compiler deservedly kicked you in the head because it is not at all clear in the real world whether you can allocate so much memory or not.
In the second case you are allocating a large chunk of memory already in runtime. And it is immediately clear whether you can allocate it or not, because the program is already working with a specific resource of the machine (memory).
And what do pointers have to do with it? Arrays in mql are of two types, predefined at compile time and dynamic. Not only pointers *, but usual class fields can point to dynamic arrays as well. So using pointers here is absolutely not justified.
s.e. Strange impression of the code, like pointers, classes, macros - with complete lack of understanding of what's going on.
If we alter the example a bit, declare it locally and put a not so ugly number, the compiler tells us directly what the problem is:
#property strict #define ARRAY_SIZE int(140000) class Test { public: int nA; double fB; datetime dtC; Test(void) : nA(0) , fB(1.0) , dtC(__DATETIME__) { }; }; void OnStart() { Test classTest[ARRAY_SIZE]; // the size of local variables is too large }If you ever want to argue with Renate, you're welcome to do so.
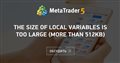
- 2014.02.08
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I'm making a class like this.
Now I want to call an object array:
How then to quickly create an array of objects if the constructor has parameters at global level?
For example? create objects first by changing the constructor and then how do I replace the objects in OnInit with symbols?
Maybe there is an easier solution ?