When using the Standard Libary to create ChartObjects, the objects are deleted upon calling the CChartObject deconstructor. Normally, this isn't a bad thing with indicators/experts/panels, or can be worked around if you call the Save/Load functions, but scripts are a run-once ordeal, so this won't work.
To get around this issue, I modified the CChartObject deconstructor as shown below, but I'm not a fan of modifying files in the Standard Library, so I was wondering if someone figured out another method of keeping the object on the chart when the script is finished running.
I thought about creating a duplicate of the object but, since there's no built-in function to create a copy of an object, I'd have to write the code myself, and that'd be re-inventing the wheel and defeats the purpose of using the Standard Library.
Thanks.
You can try to use dynamic pointers instead of automatic (using *)
CChartObject *line=new CChartObjectTrend();
And not call the destructor
You can try to use dynamic pointers instead of automatic (using *)
And not call the destructor
Thanks for the response, Amir.
Unless I'm missing something, the thing is that it will leak memory if you don't use the delete operator on the pointer when the script is finished, and using delete results in the destructor being called.
https://docs.mql4.com/basis/operators/deleteoperator
The delete operator deletes an object created by the new operator, calls the corresponding class destructor and frees up memory occupied by the object.
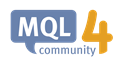
- docs.mql4.com
Thanks for the response, Amir.
Unless I'm missing something, the thing is that it will leak memory if you don't use the delete operator on the pointer when the script is finished, and using delete results in the destructor being called.
In my opinion it is released anyway at the end of the script, so if you can stand the message in the log and the leak is just because of those objects than in my view it's no harm apart from that.
I personally have a different standard lib of my own so that to me is the best solution but if you insist of not changing that lib..
* You can also try setting the m_chart_id to -1 before calling the destructor by using Detach().. That way the ObjectDelete(m_chart_id,name) won't work..
In my opinion it is released anyway at the end of the script, so if you can stand the message in the log and the leak is just because of those objects than in my view it's no harm apart from that.
I personally have a different standard lib of my own so that to me is the best solution but if you insist of not changing that lib..
* You can also try setting the m_chart_id to -1 before calling the destructor by using Detach().. That way the ObjectDelete(m_chart_id,name) won't work..
It does seem like creating a copy of the library and fixing all the issues is the best way to go. I've done that quite a bit for Panel GUI Objects but I figured that there may be another method that others were using.
As far as the m_chart_id solution, it's a private member variable with no setter, unfortunately.
Thanks again, Amir, and take care.
As far as the m_chart_id solution, it's a private member variable with no setter, unfortunately.
Thanks again, Amir, and take care.
No problem, Im not by my computer but as I recall there are methods that change it, one is Detach
*-Actually now checked it should work as mentioned above
You just need to call obj.Detach() for each object before calling it's destructor
No problem, Im not by my computer but as I recall there are methods that change it, one is Detach
*-Actually now checked it should work as mentioned above
You just need to call obj.Detach() for each object before calling it's destructor
I see what you're saying now. I'm guessing this is how they intended for developers to keep objects on the chart once the program was deinitialized. I appreciate the help!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
When using the Standard Libary to create ChartObjects, the objects are deleted upon calling the CChartObject deconstructor. Normally, this isn't a bad thing with indicators/experts/panels, or can be worked around if you call the Save/Load functions, but scripts are a run-once ordeal, so this won't work.
To get around this issue, I modified the CChartObject deconstructor as shown below, but I'm not a fan of modifying files in the Standard Library, so I was wondering if someone figured out another method of keeping the object on the chart when the script is finished running.
I thought about creating a duplicate of the object but, since there's no built-in function to create a copy of an object, I'd have to write the code myself, and that'd be re-inventing the wheel and defeats the purpose of using the Standard Library.
Thanks.