Is it possible to call one derived class into another derived class from the same Base/Parent Class ? if yes how to instantiate the 1st class into 2nd class ?
Thanks a lot Dominik.
This is really a simplest explanation of Object Class. I really appreciate your time to explain that here.
I will definitely try to work on 'is an' and 'is has' concept in my programming.
Due to tremendous pressure to complete my EA with at least one strategy and go online, I have cut of the middle layer and remained to StrategyBASE (holding variables, indicator class instantiation and common methods e.g. TrailSL ) and BreakoutCLASS (to filter range, and methods to open breakout position). So I have shifted Trend (in this case Range) calculation within BreakoutClass. The idea is to have breakout calculations into breakoutClass and trend calculations in TrendClass (with position opening methods).
What I also feel that my four major inputs for all these class declaration are Symbol, 3 Time Frames and Risk Per Trade rate. I can try to instantiate them into BASE class only by calling it from EA. Then I can use default constructor (as against parametric) for class declaration in Sub Classes.
I will find some time to implement your method into CLASS created for TESTING Purpose, and if successful will shift it to my EA project.
Once again thanks a lot for giving me direction.
Regards and wish you nice weekend.
Hi Dominik
May I request a simple example for me to understand your concept ?
thanks
If you search here for OOP and select articles you'll find lots of them about OOP and here is one for you: https://www.mql5.com/en/articles/351
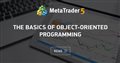
- www.mql5.com
If you search here for OOP and select articles you'll find lots of them about OOP and here is one for you: https://www.mql5.com/en/articles/351
Hi Carl
thanks for your response, and I really articles about OOP. Being a non professional programmer, I have come a long way from procedural to OOP. Now I have stabilized using OOP and going to stick to it. I have seen many useful ways I could use it. The challenges comes in when I have a new idea to implement OOP and could not find article or some other material on forum or the net.
the article you have mentioned was one of the article I have gone through on OOP before moving to it.
As @Dominik Egert has mentioned "MQL does not allow deriving from more than one class at the same time." it seems my idea of working with layers will not work.
Regards

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi Friend
I am trying to get Trend calculations in a derived class CTrend.mqh.
I want to use method of the above class to use into another derived class CBreakOut.mqh.
Both the derived classes are coming from same CStrategyBase.mqh class.
below I have tried to give some idea of what I am planning to do in terms of MQL Code.
The challenge I am facing is how to call/instantiate the CTrend into CBreakOut !!!
Your help will be highly appreciated.
Regards,