- Mql4 Trailing stop problem
- trailing stop not working
- issue with mu trailing stop loss
Only post the relevant parts of your code.
I've done it for you here, but please do it yourself in the future:
extern int WhenToTrail= 100; extern int TrailAmount= 50; int OnInit() { //--- double ticksize= MarketInfo(Symbol(),MODE_TICKSIZE); if( ticksize==0.00001 || ticksize==0.001) pips= ticksize*10; else pips=ticksize; //--- return(INIT_SUCCEEDED); } void AdjustTrail(){ for(int b=OrdersTotal()-1;b>=0;b--) { if(OrderSelect(b,SELECT_BY_POS,MODE_TRADES)) if(OrderMagicNumber()==InpMagicNumber) if(OrderSymbol()==Symbol()) if(OrderType()==OP_BUY) if(Bid-OrderOpenPrice()>WhenToTrail*pips) if(OrderStopLoss()<Bid-TrailAmount*pips) OrderModify(OrderTicket(),OrderOpenPrice(),Bid-(pips*TrailAmount),OrderTakeProfit(),0,CLR_NONE); } for(int s=OrdersTotal()-1;s>=0;s--) { if(OrderSelect(s,SELECT_BY_POS,MODE_TRADES)) if(OrderMagicNumber()==InpMagicNumber) if(OrderSymbol()==Symbol()) if(OrderType()==OP_SELL) if(OrderOpenPrice()-Ask>WhenToTrail*pips) if(OrderStopLoss()>Ask+TrailAmount*pips) OrderModify(OrderTicket(),OrderOpenPrice(),Ask+(pips*TrailAmount),OrderTakeProfit(),0,CLR_NONE); } }
The logic of your code seems right.
Be sure to check the return value of OrderModify.
Returned value
If the function succeeds, it returns true, otherwise false. To get the detailed error information, call the GetLastError() function.
It's also recommended that your Normalize the price of the stoploss since you performed arithmetic on it.
Side notes:
I also recommend you refactor (clean up and optimize) your code.
For example, you have two for-loops to distinguish between Buy orders and Sell orders, when you only need one for-loop and then run a check on OrderType to distinguish between OP_BUY and OP_SELL.
Another thing you can do is store the boolean values from the if-statements into variables with descriptive names so it's easier to read, debug, and maintain your code.
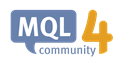
- docs.mql4.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use