I want to detect the current order was closed and total order is decreased.
But this code sometimes does not work.
It did not detect the N of order is decreased.
Can you point out the problem?
Your if Statement will never be true:
- Previous Order Count should be saved (static), not the current count.
- You are Copying Previous and Current Count before comparing them.
Here:
void OnTick() { //- Previous Count Memory & New Count static int OrdersTotalPrevious = 0; int OrdersTotalCurrent = OrdersTotal(); //- Send Test Order if(OrdersTotalCurrent == 0){ if(!OrderSend(_Symbol,OP_BUY,0.02,Ask,30,Ask - 0.0005, Ask + 0.0005,"TEST",0,0,clrNONE)) { Print("Could not send Order."); } } //- Compare Previous Count to New Count if(OrdersTotalCurrent < OrdersTotalPrevious) { Print("Now it Works."); ExpertRemove(); } //- Save Count for Next Event OrdersTotalPrevious = OrdersTotalCurrent; }
I prefer to look for OrderCloseTime() to see if my positions are still Open, there are probably other ways to do it too.
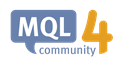
- docs.mql4.com
Your if Statement will never be true:
- Previous Order Count should be saved (static), not the current count.
- You are Copying Previous and Current Count before comparing them.
Here:
I prefer to look for OrderCloseTime() to see if my positions are still Open, there are probably other ways to do it too.
Jeremie san
Now it worked!
Maybe I needed this?
//- Save Count for Next Event
OrdersTotalPrevious = OrdersTotalCurrent;
Neither remembering the count
static int OTcurr=0; OTprev = OTcurr; OTcurr = OrdersTotal();or monitoring the time (remembering the ticket) are a good idea.
EAs must be coded to recover. If the power fails, OS crashes, terminal or chart is accidentally closed, on the next tick, any static/global ticket variables will have been lost. You will have an open order but don't know it, so the EA will never try to close it, trail SL, etc. How are you going to recover?
Use a OrderSelect / Position select loop to recover, or persistent storage (GV+flush or files) of ticket numbers required.
On a network disconnection you might get ERR_NO_RESULT or ERR_TRADE_TIMEOUT. There is nothing to be done, but log the error, return and wait for a reconnection and a new tick. Then reevaluate. Was an order opened or is the condition still valid.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I want to detect the current order was closed and total order is decreased.
But this code sometimes does not work.
It did not detect the N of order is decreased.
Can you point out the problem?