Hello,
As I understand from the article, the function "setBokerOffset ()" should work in the strategy tester as well, but it doesn't work.
void OnTick() { //--- bool isTimeSet = setBokerOffset(); if(!isTimeSet) { Alert("setBokerOffset failed"); return; } }
array out of range in 'DealingWithTime.mqh' (201,21)
Does the "The Alternative using it via Input Variables" the only way to get correct times in the strategy tester?
Hello,
As I understand from the article, the function "setBokerOffset ()" should work in the strategy tester as well, but it doesn't work.
Does the "The Alternative using it via Input Variables" the only way to get correct times in the strategy tester?
Good article. However, one problem arises: how to determine that a broker/dealer is switching from winter/summer without contacting its technical support?
- Why not ask?
- The software knows when summer/winter time is in the US and EU and uses that to calculate the broker's offset.
Good article. However, one problem arises: how to determine that a broker/dealer is switching from winter/summer without contacting its technical support?
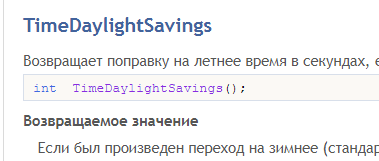
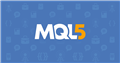
- www.mql5.com
- Why not ask?
- The software knows when summer/winter time is in the US and EU and uses that to calculate the broker offset.
1. Because support doesn't always give correct information. You yourself pointed this out about the Alpari dealer. + it is overhead: to find out from each dealer the transition. Because then you can't create a good solution, I don't know who the end user is discussing with.
2. Well, sort of, yes, but if the dealer does not transition from winter to summer and back, then you get some strange thing in the calculations.
I tried to modify your library a bit, but apparently something went wrong. I thought that the code should lead to the fact that the Expert Advisor automatically detects GMT time and trades according to GMT, not according to the broker's server. I am not sure if the code is optimal, but the solution seems to work. However, in those dealers that do not change the time - there are some incorrect calculations.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
New article Dealing with Time (Part 2): The Functions has been published:
Determing the broker offset and GMT automatically. Instead of asking the support of your broker, from whom you will probably receive an insufficient answer (who would be willing to explain a missing hour), we simply look ourselves how they time their prices in the weeks of the time changes — but not cumbersome by hand, we let a program do it — why do we have a PC after all.
Before the functions in the include file DealingWithTime.mqh (and after the macro substitution) the required variables are declared as global variables:
These variables DST_USD, DST_EUR,.. will have the actual time shift of the USA, the EU,.. They will be updated and set by our functions. In the winter time which is the normal time they are zero: the time is not shifted at that period.
After that we have the variables with the next time the time changeover will take place. They are is mainly needed to know when a new calculation is required in order to save the CPU resources:
We will consider the Russian situation later in this article.
This structure and its global variable is the heart of all. :)
We will assign the broker offsets for the three relevant periods and duration of the forex market is open in these periods, for both the actual value and for an easy check set if the values has been assigned. The global variable is named OffsetBroker, we will meet it several times.
Author: Carl Schreiber