Millard Melnyk:
whose timeseries flag==true, I must make sure that the target array's timeseries flag==false, copy the source array to the target array at the last index+1, then reset the timeseries flag to its original value in case it was set ==true.
whose timeseries flag==true, I must make sure that the target array's timeseries flag==false, copy the source array to the target array at the last index+1, then reset the timeseries flag to its original value in case it was set ==true.
Is all this necessary?
yes,
this influence either to the right or to the left tail of arrayBuffer you add elements: if to the index+1 (it is to the left side)
because the newest bar from right-side has index 0) - Timeseries - general use
ArraySetAsSeries(Buffer,false); ArrayResize(Buffer,Bars); // and make your copy ArraySetAsSeries(Buffer,true);
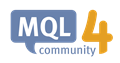
Timeseries and Indicators Access - MQL4 Reference
- docs.mql4.com
Timeseries and Indicators Access - MQL4 Reference
JeeyCi #:
yes,
this influence either to the right or to the left tail of arrayBuffer you add elements: if to the index+1 (it is to the left side)
because the newest bar from right-side has index 0) - Timeseries - general use
Thank you!

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Again, cloned from https://www.mql5.com/en/docs/indicators/ima, modified because I'm going to try doing calculations on the series of iMA values, so I need to save new data as they develop every tick and I'd rather not copy the entire timeseries once for every tick (which could be some incredible overhead when ticks are flying in fast and furious, lol.)
The whole code is here, but the code I'm asking about is lines 147-152 (set off with white space).
(EDIT: no idea why the spacing turned out like it did, I fixed it. Would be nice if the input code feature had an option so that it showed line numbers. I had to put them in elsewhere and paste the results.)
Since the logical compliment to ArrayCopy(pdData,dCpyBfr,ArraySize(pdData)+1,0,iCopyCNT); (i.e., ArrayCopy(pdData,dCpyBfr,-1,0,iCopyCNT);) does not work with arrays whose timeseries flag==true, I must make sure that the target array's timeseries flag==false, copy the source array to the target array at the last index+1, then reset the timeseries flag to its original value in case it was set ==true.
Is all this necessary? Or is there a cleaner way to append new data to my array of moving average values, since CopyBuffer doesn't seem to give the option of appending new data directly?