I follow this guide to create classes loaded like usual variables, ie "my_class class1(Param1, Param2,...);" with ''parametric constructor".
chapter "Passing Parameters to Constructor"
https://www.mql5.com/en/articles/351
my problem is that when i create a class3 of a class2 of a class1, I can't give a default value of class2, in the constructor of class3, because class2 is ''passed as reference''.
Here is my situation:
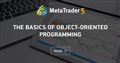
The Basics of Object-Oriented Programming
- www.mql5.com
You don't need to know what are polymorphism, encapsulation, etc. all about in to use object-oriented programming (OOP)... you may simply use these features. This article covers the basics of OOP with hands-on examples.
- Passing a dynamic object array via reference
- "declaration without type" Why does this error? Communication between classes
- Errors, bugs, questions
class class2 { public : class1 m_Klass1; int m_num2; //--- class2( void ) {} class2(class1&c_Klass1, int c_num2= 74 ) { init_2(c_Klass1,c_num2); } void init_2(class1&i_Klass1, int i_num2) { m_Klass1=i_Klass1; m_num2=i_num2; } //class2(void); ~class2( void ); }; //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ class2 number2A(number1, 10); class2 number2B(number1, 32); //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ //class2 c_Klass2(number1,73); //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ class class3 { public : class2 m_Klass2A; class2 m_Klass2B; int m_num3; //--- class3(class2&c_Klass2A,class2&c_Klass2B, int c_num3= 30 ) { init_3(c_Klass2A,c_Klass2B,c_num3); } void init_3(class2&i_Klass2A,class2&i_Klass2B, int i_num3) { m_Klass2A=i_Klass2A; m_Klass2B=i_Klass2B; m_num3=i_num3; } //class3(void); ~class3( void ); }; class3 TmpClass3(number2A, number2B, 83);
fxsaber:
Your code works. Now If I UNcomment the default constructor of class1, I get the error :
'class1' - ambiguous call to overloaded function with the same parameters
could be one of 2 function(s)
class1::class1()
class1::class1(int)
'class1' - ambiguous call to overloaded function with the same parameters
could be one of 2 function(s)
class1::class1()
class1::class1(int)
2 errors, 0 warnings
How do I choose the constructor of class1, between
class1::class1()
and
class1::class1(int) ??
THis is the code which produces the error
class class1 { public: int m_num1; //--- class1(int c_num1=99) { init_1(c_num1); } void init_1(int i_num1) { m_num1=i_num1; } class1(void) {};////<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<< Now UN commented ~class1(void) {}; }; //--- class1 number1(30); //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ class class2 { public: class1 m_Klass1; int m_num2; //--- class2(class1&c_Klass1,int c_num2=74)////<<<<<<<<<<<<<<<<<<problem here { init_2(c_Klass1,c_num2); } void init_2(class1&i_Klass1,int i_num2) { m_Klass1=i_Klass1; m_num2=i_num2; } class2(void) {}; ~class2(void) {}; }; //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ class2 number2A(number1,10); class2 number2B(number1,32); //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ class class3 { public: class2 m_Klass2A; class2 m_Klass2B; int m_num3; //--- class3(class2&c_Klass2A,class2&c_Klass2B,int c_num3=30) { init_3(c_Klass2A,c_Klass2B,c_num3); } void init_3(class2&i_Klass2A,class2&i_Klass2B,int i_num3) { m_Klass2A=i_Klass2A; m_Klass2B=i_Klass2B; m_num3=i_num3; } class3(void) {}; ~class3(void) {}; }; //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ class3 temp3(number2A,number2B,83);
Enau:
Your code works. Now If I UNcomment the default constructor of class1, I get the error :
Then you yourself.
You need your special constructor not to have a default input assigned to the input parameter.
If you need a default constructor, go like this:
class1() : c_num(99) {};
This is a default constructor with initialization.
The other taking input parameters are not default constructors. Do not use presets in them.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register