Javascript has libraries to create hmac sha256 and base64 encoding while mql5 is missing the hmac within the CryptEncode function. Thankfully there is a library for hmac sha here https://www.mql5.com/en/code/21065
Below is the output from the javascript code.
Now I am trying to duplicate the output within mql5... HMAC sha256 to string is simple thanks to the library.
This will output: MQL HMACsha256=3b3803c66fe6688cc3ddcc02ca7fa6fd940a326cefbd1e3606c42eccbb21bdba
Output: MQL BASE64=M2IzODAzYzY2ZmU2Njg4Y2MzZGRjYzAyY2E3ZmE2ZmQ5NDBhMzI2Y2VmYmQxZTM2MDZjNDJlY2NiYjIxYmRiYQ==
Well that's not right. What could have been the issue? If you take the HMAC sha256 string into any online base64 encoder you will have this output because we're taking a string of the hmac sha256 and base64 encoding it.
I have tried to verify your issue, but when using this encoder/decoder, everything seems to line up correctly.
Please verify the output from javascript, as it seems to be an encoding issue somewhere with the charset in use.
- www.base64decode.net
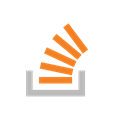
- 2015.08.24
- Bobface Bobface 2,402 4 4 gold badges 17 17 silver badges 52 52 bronze badges
- stackoverflow.com
https://stackoverflow.com/questions/32188149/difference-between-cryptojs-enc-base64-stringify-and-normal-base64-encryption
Thank you for pointing this out.
https://stackoverflow.com/questions/32188149/difference-between-cryptojs-enc-base64-stringify-and-normal-base64-encryption
sorry to bring up a 2 years old topic again. I didn't understand at last what was the point? is the mql crypt encode function working correctly ? and the result differences are not important what we see at print logs? please explain more. thanks in advanced.
int res = CryptEncode(CRYPT_BASE64,str,key,dst);
edit 1:
thanks to that post in stackoverflow , after multiple hours of trial and reading it over and over again. I finally understood what he wrote.
when we use that sha256 library , the returned value is in hex format not decimal or utf normal strings.
so I wrote a function for finding hex characters and then when I had an array with hex data, I used it on cryptencode as src array, and an empty array as key, and another array for base64encoded results.
and finally it works :D

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Javascript has libraries to create hmac sha256 and base64 encoding while mql5 is missing the hmac within the CryptEncode function. Thankfully there is a library for hmac sha here https://www.mql5.com/en/code/21065
Below is the output from the javascript code.
3b3803c66fe6688cc3ddcc02ca7fa6fd940a326cefbd1e3606c42eccbb21bdba (string hmac sha256) OzgDxm/maIzD3cwCyn+m/ZQKMmzvvR42BsQuzLshvbo= (string base64)
Now I am trying to duplicate the output within mql5... HMAC sha256 to string is simple thanks to the library.
This will output: MQL HMACsha256=3b3803c66fe6688cc3ddcc02ca7fa6fd940a326cefbd1e3606c42eccbb21bdba
Output: MQL BASE64=M2IzODAzYzY2ZmU2Njg4Y2MzZGRjYzAyY2E3ZmE2ZmQ5NDBhMzI2Y2VmYmQxZTM2MDZjNDJlY2NiYjIxYmRiYQ==
Well that's not right. What could have been the issue? If you take the HMAC sha256 string into any online base64 encoder you will have this output because we're taking a string of the hmac sha256 and base64 encoding it.