ok I think I totally misunderstood how this works
so apparently it's not possible to create a custom symbol in the strategy tester
so my question is : how do you programatically fill the values of the custom symbol you wanna work on ?
and how do you choose which data it's coming from ? (ticks, ticks based on history,...etc)
this would be so much easier if you could create custom symbols in the strategy tester, then you can create any data you need from selected data type
Jeff
EDIT : ok so apparently you can create custom symbols using scripts
but how can you select which data it uses when using Tick Copy functions ? (real, real ticks based on history, etc...) ?
https://www.mql5.com/en/articles/3540
Jeff
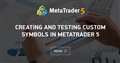
- www.mql5.com
finally found a code that works.
I Adapted it to my liking
here it is if anybody interested
//+------------------------------------------------------------------+ //| GetCandle2.mq5 | //| Aleksey Zinovik | //| | //+------------------------------------------------------------------+ #property copyright "Aleksey Zinovik" #property link "" #property version "1.00" #property script_show_inputs //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ /*input params*/ input string SName="ExampleCurrency10"; input datetime TBegin=D'2021.03.07 00:00:00'; // Start time for bar generation input int bars=100; // Number of bars /*----input params----*/ int i_bar=0; // counter of minute bars MqlRates MRatesMin[]; // array for storing bars as bars int ReplaceBar=0; // number of replaced bars //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void OnStart() { datetime TCurrent=TBegin; if(!SymbolInfoInteger(SName,SYMBOL_CUSTOM))// if the symbol exists { Print("Symbol ",SName," does not exist. Trying to create it"); CustomSymbolCreate(SName); } ArrayResize(MRatesMin,bars); int idxStart = iBarShift(NULL,0,TBegin,false); i_bar = 0; while(i_bar < bars) { int i = bars-1-i_bar + idxStart; double ask = iClose(NULL,0,i); double bid = iClose(NULL,0,i); MRatesMin[i_bar].open=iOpen(NULL,0,i); MRatesMin[i_bar].close=iClose(NULL,0,i); MRatesMin[i_bar].high=iHigh(NULL,0,i); MRatesMin[i_bar].low=iLow(NULL,0,i); // generate volume, spread MRatesMin[i_bar].real_volume = iVolume(NULL,0,i); MRatesMin[i_bar].tick_volume = iTickVolume(NULL,0,i); MRatesMin[i_bar].spread = (ask-bid)/(10.0*Point()); // store the time MRatesMin[i_bar].time=iTime(NULL,0,i); i_bar++; } if(i_bar>0) { i_bar--; ReplaceHistory(MRatesMin[0].time,MRatesMin[i_bar].time); } } //+------------------------------------------------------------------+ void ReplaceHistory(datetime DBegin,datetime DEnd) { ReplaceBar=CustomRatesReplace(SName,DBegin,DEnd,MRatesMin); if(ReplaceBar<0) Print("Error replacing bars. Error code: ",GetLastError()); else PrintFormat("Price history for period: %s to %s generated successfully. Created %i bars, added (replaced) %i bars",TimeToString(DBegin),TimeToString(DEnd),i_bar+1,ReplaceBar); } //+------------------------------------------------------------------+ //+------------------------------------------------------------------+
I will try to make it work in EAs if possible. let's see
because my initial conclusion was wrong (I wasn't able to make it work in Scripts either)
Hello Jean .
What is the purpose of the synthetic ,what will its use be ?

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi
let's say that I want to make my own OHLC symbol from ticks based on history.
i'm not sure how to do so, because to set the close value, you need to set it one tick before the new bar, but how do you know it's the last tick ?
if you set it after the new bar, then it's data based on the new bar, and it becomes the open of the new bar, but you cannot set the close of the previous bar anymore
Am I missing something ?
thanks
Jeff