PositionSelect comes in handy.
The code also assumes that you have an array of symbols array_symbols (this array contains symbols by which you check signals).
int total=ArraySize(array_symbols); for(int i=0; i<total; i++) { if(PositionSelect(array_symbols[i])) { Print("This ",array_symbols[i]," has a position already"); continue; } else { //--- you code open position } }
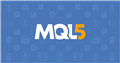
- www.mql5.com
PositionSelect comes in handy.
The code also assumes that you have an array of symbols array_symbols (this array contains symbols by which you check signals).
Thank you very much. This is a new perspective I am learning. I have added it to my code and I am getting the following on the compiler:
'array_symbols' - undeclared identifier
'array_symbols' - variable expected.
Given that the symbols will be open randomly, please guide me on how to declare them and have them as variables.
Thank you very much. This is a new perspective I am learning. I have added it to my code and I am getting the following on the compiler:
'array_symbols' - undeclared identifier
'array_symbols' - variable expected.
Given that the symbols will be open randomly, please guide me on how to declare them and have them as variables.
I gave you a description that ...
So you need to either create and fill this array or use the name of any symbol instead of an array.
I created an array but still the EA opens more positions per currency. Kinly guide me on where the issue could be. Thank you.
string array_symbols[] = { "EURUSD","GBPUSD","USDCHF","NZDUSD"}; int total = ArraySize(array_symbols); for(int i=0; i<total; i++) { string symbol=array_symbols[i]; //--Loop through open positions uint total1=PositionsTotal(); for(uint j=0; j<total1; j++) { string symbol1=PositionGetSymbol(j); if(symbol==symbol1) //If position symbol equals the array_size symbol { Print("This ",array_symbols[i]," has a position already"); continue; } else { //------------ } } }
I created an array but still the EA opens more positions per currency. Kinly guide me on where the issue could be. Thank you.
Use like this:
string array_symbols[4] = {"EURUSD","GBPUSD","USDCHF","NZDUSD"}; int total = ArraySize(array_symbols); for(int i=0; i<total; i++) { for(int j=PositionsTotal()-1; j>=0; j--) // returns the number of current positions { ulong ticket=PositionGetTicket(j); if(ticket>0); { if(PositionGetString(POSITION_SYMBOL)==array_symbols[i]) { Print("This ",array_symbols[i]," has a position already"); continue; } else { /* Open position AND return!!! */ } } } }
and note: after opening a position, execute return.
Use like this:
and note: after opening a position, execute return.
Hi,
Thank you for your effort in assisting me this far. I have made the necessary changes but still it is opening multiple positions.
I have added a very simple EA that can open positions quickly for testing purposes. I wish it limits the positions to just one per currency in the array. Thank you.
//+------------------------------------------------------------------+ //| Code_From_Scratch.mq5 | //| Copyright 2021, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2021, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ #include <Trade\PositionInfo.mqh> #include <Trade\Trade.mqh> CTrade trade; int handleTrendFastMa; int handleTrendSlowMa; int OnInit() { //--- handleTrendFastMa = iMA(_Symbol,PERIOD_H1,12,0,MODE_EMA,PRICE_CLOSE); handleTrendSlowMa = iMA(_Symbol,PERIOD_H1,21,0,MODE_EMA,PRICE_CLOSE); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- double Ask = NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_ASK),_Digits); //Get the ask price double Bid = NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_BID), _Digits); MqlRates rates[]; ArraySetAsSeries(rates, true); int copied = CopyRates(_Symbol,PERIOD_H1,0,4,rates); double pr0_close = rates[0].close; double pr0_open = rates[0].open; double pr1_close = rates[1].close; double pr1_open = rates[1].open; double pr2_close = rates[2].close; double pr2_open = rates[2].open; //-------------------------------------------------------------------- //------------------------------------------------------------------- string array_symbols[4] = {"EURUSD","GBPUSD","USDCHF","NZDUSD"}; int total = ArraySize(array_symbols); for(int i=0; i<total; i++) { for(int j=PositionsTotal()-1; j>=0; j--) // returns the number of current positions { ulong ticket=PositionGetTicket(j); if(ticket>0); { if(PositionGetString(POSITION_SYMBOL)==array_symbols[i]) { Print("This ",array_symbols[i]," has a position already"); continue; } else { //----------------------------------------------------------------- if(pr1_close > pr1_open && pr2_close > pr2_open){ CheckTrailingStop(Ask); if(PositionsTotal() <= 2){ trade.Buy(0.01,_Symbol,Ask,(Ask-500*_Point),(Ask+500*_Point),NULL); } } else if(pr1_close < pr1_open && pr2_close < pr2_open){ CheckTrailingStop1(Bid); if(PositionsTotal() <= 2){ trade.Sell(0.01,_Symbol,Bid,(Bid+500*_Point),(Bid-500*_Point),NULL); } } }return; } } } } void CheckTrailingStop(double Ask){ //set the desired stop loss to 150 points double SL=NormalizeDouble(Ask-500*_Point,_Digits); //Check all the current open positions for the current symbol for(int i=PositionsTotal()-1;i>=0;i--)// Count all currency pair positions { string symbol=PositionGetSymbol(i); if(_Symbol==symbol){ //If chart symbol equals position symbol //Get the ticket number ulong PositionTicket=PositionGetInteger(POSITION_TICKET); //Get the current Stop Loss double CurrentStopLoss=PositionGetDouble(POSITION_SL); //if current stop loss is below 150 points from Ask price if(CurrentStopLoss<SL){ //Modify the stop loss by 10 points trade.PositionModify(PositionTicket,(CurrentStopLoss+10*_Point),0); } }//End Symbol in loop }//End for loop }//End trailing stop void CheckTrailingStop1(double Bid){ double SL=NormalizeDouble(Bid+500*_Point,_Digits);//Set the stop loss to 150 points for(int i=PositionsTotal()-1; i>=0; i--){ string symbol=PositionGetSymbol(i); //get the symbol position if(_Symbol==symbol)//if the currency pair is equal if(PositionGetInteger(POSITION_TYPE)==ORDER_TYPE_SELL){//IF WE HAVE A sell position ulong PositionTicket=PositionGetInteger(POSITION_TICKET);//calculate the current stop loss double CurrentStopLoss = PositionGetDouble(POSITION_SL);//Get the ticket type if(CurrentStopLoss>SL){//If the current top loss is more than 180 trade.PositionModify(PositionTicket,(CurrentStopLoss-10*_Point),0); } } } } //+------------------------------------------------------------------+ //| Trade function | //+------------------------------------------------------------------+ void OnTrade() { //--- } //+------------------------------------------------------------------+
Hi,
Thank you for your effort in assisting me this far. I have made the necessary changes but still it is opening multiple positions.
I have added a very simple EA that can open positions quickly for testing purposes. I wish it limits the positions to just one per currency in the array. Thank you.
I cannot read your code - please use the Code Styler in MetaEditor. Also tell me - is it important for you to check the signal AT EVERY TICK or is it possible to check the signal ONLY AT THE MOMENT OF A NEW BAR'S BIRTH?
//+------------------------------------------------------------------+ //| Code_From_Scratch.mq5 | //| Copyright 2021, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2021, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ #include <Trade\PositionInfo.mqh> #include <Trade\Trade.mqh> CTrade trade; int handleTrendFastMa; int handleTrendSlowMa; int OnInit() { //--- handleTrendFastMa = iMA(_Symbol,PERIOD_H1,12,0,MODE_EMA,PRICE_CLOSE); handleTrendSlowMa = iMA(_Symbol,PERIOD_H1,21,0,MODE_EMA,PRICE_CLOSE); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- double Ask = NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_ASK),_Digits); //Get the ask price double Bid = NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_BID), _Digits); MqlRates rates[]; ArraySetAsSeries(rates, true); int copied = CopyRates(_Symbol,PERIOD_H1,0,4,rates); double pr0_close = rates[0].close; double pr0_open = rates[0].open; double pr1_close = rates[1].close; double pr1_open = rates[1].open; double pr2_close = rates[2].close; double pr2_open = rates[2].open; //-------------------------------------------------------------------- //------------------------------------------------------------------- string array_symbols[4] = {"EURUSD","GBPUSD","USDCHF","NZDUSD"}; int total = ArraySize(array_symbols); for(int i=0; i<total; i++) { for(int j=PositionsTotal()-1; j>=0; j--) // returns the number of current positions { ulong ticket=PositionGetTicket(j); if(ticket>0); { if(PositionGetString(POSITION_SYMBOL)==array_symbols[i]) { Print("This ",array_symbols[i]," has a position already"); continue; } else { //----------------------------------------------------------------- if(pr1_close > pr1_open && pr2_close > pr2_open){ CheckTrailingStop(Ask); if(PositionsTotal() <= 2){ trade.Buy(0.01,_Symbol,Ask,(Ask-500*_Point),(Ask+500*_Point),NULL); } } else if(pr1_close < pr1_open && pr2_close < pr2_open){ CheckTrailingStop1(Bid); if(PositionsTotal() <= 2){ trade.Sell(0.01,_Symbol,Bid,(Bid+500*_Point),(Bid-500*_Point),NULL); } } }return; } } } } void CheckTrailingStop(double Ask){ //set the desired stop loss to 150 points double SL=NormalizeDouble(Ask-500*_Point,_Digits); //Check all the current open positions for the current symbol for(int i=PositionsTotal()-1;i>=0;i--)// Count all currency pair positions { string symbol=PositionGetSymbol(i); if(_Symbol==symbol){ //If chart symbol equals position symbol //Get the ticket number ulong PositionTicket=PositionGetInteger(POSITION_TICKET); //Get the current Stop Loss double CurrentStopLoss=PositionGetDouble(POSITION_SL); //if current stop loss is below 150 points from Ask price if(CurrentStopLoss<SL){ //Modify the stop loss by 10 points trade.PositionModify(PositionTicket,(CurrentStopLoss+10*_Point),0); } }//End Symbol in loop }//End for loop }//End trailing stop void CheckTrailingStop1(double Bid){ double SL=NormalizeDouble(Bid+500*_Point,_Digits);//Set the stop loss to 150 points for(int i=PositionsTotal()-1; i>=0; i--){ string symbol=PositionGetSymbol(i); //get the symbol position if(_Symbol==symbol)//if the currency pair is equal if(PositionGetInteger(POSITION_TYPE)==ORDER_TYPE_SELL){//IF WE HAVE A sell position ulong PositionTicket=PositionGetInteger(POSITION_TICKET);//calculate the current stop loss double CurrentStopLoss = PositionGetDouble(POSITION_SL);//Get the ticket type if(CurrentStopLoss>SL){//If the current top loss is more than 180 trade.PositionModify(PositionTicket,(CurrentStopLoss-10*_Point),0); } } } } //+------------------------------------------------------------------+ //| Trade function | //+------------------------------------------------------------------+ void OnTrade() { //--- } //+------------------------------------------------------------------+
I cannot read your code - please use the Code Styler in MetaEditor. Also tell me - is it important for you to check the signal AT EVERY TICK or is it possible to check the signal ONLY AT THE MOMENT OF A NEW BAR'S BIRTH?
Hi to you,
I hope you can read it now. My full code relies on new bars and not every tick. Therefore, I will be more comfortable if the code runs once a new bar starts forming. After that, it will remain dormant until another bar forms.
Thank you.
Here is the code after the styler:
//+------------------------------------------------------------------+ //| Code_From_Scratch.mq5 | //| Copyright 2021, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2021, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ #include <Trade\PositionInfo.mqh> #include <Trade\Trade.mqh> CTrade trade; int handleTrendFastMa; int handleTrendSlowMa; //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ int OnInit() { //--- handleTrendFastMa = iMA(_Symbol,PERIOD_H1,12,0,MODE_EMA,PRICE_CLOSE); handleTrendSlowMa = iMA(_Symbol,PERIOD_H1,21,0,MODE_EMA,PRICE_CLOSE); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- double Ask = NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_ASK),_Digits); //Get the ask price double Bid = NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_BID), _Digits); MqlRates rates[]; ArraySetAsSeries(rates, true); int copied = CopyRates(_Symbol,PERIOD_H1,0,4,rates); double pr0_close = rates[0].close; double pr0_open = rates[0].open; double pr1_close = rates[1].close; double pr1_open = rates[1].open; double pr2_close = rates[2].close; double pr2_open = rates[2].open; //-------------------------------------------------------------------- //------------------------------------------------------------------- string array_symbols[4] = {"EURUSD","GBPUSD","USDCHF","NZDUSD"}; int total = ArraySize(array_symbols); for(int i=0; i<total; i++) { for(int j=PositionsTotal()-1; j>=0; j--) // returns the number of current positions { ulong ticket=PositionGetTicket(j); if(ticket>0); { if(PositionGetString(POSITION_SYMBOL)==array_symbols[i]) { Print("This ",array_symbols[i]," has a position already"); continue; } else { //----------------------------------------------------------------- if(pr1_close > pr1_open && pr2_close > pr2_open) { CheckTrailingStop(Ask); if(PositionsTotal() <= 2) { trade.Buy(0.01,_Symbol,Ask,(Ask-500*_Point),(Ask+500*_Point),NULL); } } else if(pr1_close < pr1_open && pr2_close < pr2_open) { CheckTrailingStop1(Bid); if(PositionsTotal() <= 2) { trade.Sell(0.01,_Symbol,Bid,(Bid+500*_Point),(Bid-500*_Point),NULL); } } } return; } } } } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void CheckTrailingStop(double Ask) { //set the desired stop loss to 150 points double SL=NormalizeDouble(Ask-500*_Point,_Digits); //Check all the current open positions for the current symbol for(int i=PositionsTotal()-1; i>=0; i--) // Count all currency pair positions { string symbol=PositionGetSymbol(i); if(_Symbol==symbol) //If chart symbol equals position symbol { //Get the ticket number ulong PositionTicket=PositionGetInteger(POSITION_TICKET); //Get the current Stop Loss double CurrentStopLoss=PositionGetDouble(POSITION_SL); //if current stop loss is below 150 points from Ask price if(CurrentStopLoss<SL) { //Modify the stop loss by 10 points trade.PositionModify(PositionTicket,(CurrentStopLoss+10*_Point),0); } }//End Symbol in loop }//End for loop }//End trailing stop //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void CheckTrailingStop1(double Bid) { double SL=NormalizeDouble(Bid+500*_Point,_Digits);//Set the stop loss to 150 points for(int i=PositionsTotal()-1; i>=0; i--) { string symbol=PositionGetSymbol(i); //get the symbol position if(_Symbol==symbol)//if the currency pair is equal if(PositionGetInteger(POSITION_TYPE)==ORDER_TYPE_SELL) //IF WE HAVE A sell position { ulong PositionTicket=PositionGetInteger(POSITION_TICKET);//calculate the current stop loss double CurrentStopLoss = PositionGetDouble(POSITION_SL);//Get the ticket type if(CurrentStopLoss>SL) //If the current top loss is more than 180 { trade.PositionModify(PositionTicket,(CurrentStopLoss-10*_Point),0); } } } } //+------------------------------------------------------------------+ //| Trade function | //+------------------------------------------------------------------+ void OnTrade() { //--- } //+------------------------------------------------------------------+
You need to use this construction (catch a new bar)
//+------------------------------------------------------------------+ //| Code_From_Scratch.mq5 | //| Copyright 2021, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2021, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ #include <Trade\PositionInfo.mqh> #include <Trade\Trade.mqh> CTrade trade; int handleTrendFastMa; int handleTrendSlowMa; //--- datetime m_prev_bars = 0; // "0" -> D'1970.01.01 00:00'; //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ int OnInit() { //--- handleTrendFastMa = iMA(_Symbol,PERIOD_H1,12,0,MODE_EMA,PRICE_CLOSE); handleTrendSlowMa = iMA(_Symbol,PERIOD_H1,21,0,MODE_EMA,PRICE_CLOSE); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { datetime time_0=iTime(Symbol(),Period(),0); if(time_0==m_prev_bars) return; m_prev_bars=time_0; //--- double Ask = NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_ASK),_Digits); //Get the ask price double Bid = NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_BID), _Digits); MqlRates rates[]; ArraySetAsSeries(rates, true); int copied = CopyRates(_Symbol,PERIOD_H1,0,4,rates); double pr0_close = rates[0].close; double pr0_open = rates[0].open; double pr1_close = rates[1].close; double pr1_open = rates[1].open; double pr2_close = rates[2].close; double pr2_open = rates[2].open; //-------------------------------------------------------------------- //------------------------------------------------------------------- string array_symbols[4] = {"EURUSD","GBPUSD","USDCHF","NZDUSD"}; int total = ArraySize(array_symbols); for(int i=0; i<total; i++) { for(int j=PositionsTotal()-1; j>=0; j--) // returns the number of current positions { ulong ticket=PositionGetTicket(j); if(ticket>0); { if(PositionGetString(POSITION_SYMBOL)==array_symbols[i]) { Print("This ",array_symbols[i]," has a position already"); continue; } else { //----------------------------------------------------------------- if(pr1_close > pr1_open && pr2_close > pr2_open) { CheckTrailingStop(Ask); if(PositionsTotal() <= 2) { trade.Buy(0.01,_Symbol,Ask,(Ask-500*_Point),(Ask+500*_Point),NULL); } } else if(pr1_close < pr1_open && pr2_close < pr2_open) { CheckTrailingStop1(Bid); if(PositionsTotal() <= 2) { trade.Sell(0.01,_Symbol,Bid,(Bid+500*_Point),(Bid-500*_Point),NULL); } } } return; } } } } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void CheckTrailingStop(double Ask) { //set the desired stop loss to 150 points double SL=NormalizeDouble(Ask-500*_Point,_Digits); //Check all the current open positions for the current symbol for(int i=PositionsTotal()-1; i>=0; i--) // Count all currency pair positions { string symbol=PositionGetSymbol(i); if(_Symbol==symbol) //If chart symbol equals position symbol { //Get the ticket number ulong PositionTicket=PositionGetInteger(POSITION_TICKET); //Get the current Stop Loss double CurrentStopLoss=PositionGetDouble(POSITION_SL); //if current stop loss is below 150 points from Ask price if(CurrentStopLoss<SL) { //Modify the stop loss by 10 points trade.PositionModify(PositionTicket,(CurrentStopLoss+10*_Point),0); } }//End Symbol in loop }//End for loop }//End trailing stop //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void CheckTrailingStop1(double Bid) { double SL=NormalizeDouble(Bid+500*_Point,_Digits);//Set the stop loss to 150 points for(int i=PositionsTotal()-1; i>=0; i--) { string symbol=PositionGetSymbol(i); //get the symbol position if(_Symbol==symbol)//if the currency pair is equal if(PositionGetInteger(POSITION_TYPE)==ORDER_TYPE_SELL) //IF WE HAVE A sell position { ulong PositionTicket=PositionGetInteger(POSITION_TICKET);//calculate the current stop loss double CurrentStopLoss = PositionGetDouble(POSITION_SL);//Get the ticket type if(CurrentStopLoss>SL) //If the current top loss is more than 180 { trade.PositionModify(PositionTicket,(CurrentStopLoss-10*_Point),0); } } } } //+------------------------------------------------------------------+ //| Trade function | //+------------------------------------------------------------------+ void OnTrade() { //--- } //+------------------------------------------------------------------+

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi all,
I have had quite a challenge in that my code opens positions up to the limit of PositionsTotal()<=say 10.
I wish to have one position per currency pair at any given time. Kindly find attached part of my code for that part which is not limiting open orders. Please point me to what I could am not doing right.
Thank you.