Josh Pilisuk :
I want to lower the TP of my first opened position by 10 pips, how would you do this?
The "first" position is the position with the shortest opening time. You started using Ctrade - that's good. You need to go ahead and use CPositionInfo.
//+------------------------------------------------------------------+ //| 1.mq5 | //| Copyright 2020, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2020, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" //--- #include <Trade\PositionInfo.mqh> #include <Trade\Trade.mqh> //--- CPositionInfo m_position; // object of CPositionInfo class CTrade m_trade; // object of CTrade class //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- m_trade.SetExpertMagicNumber(300); m_trade.SetMarginMode(); m_trade.SetTypeFillingBySymbol(Symbol()); m_trade.SetDeviationInPoints(10); //--- datetime time=D'2500.01.01 00:00'; ulong ticket=0; for(int i=PositionsTotal()-1; i>=0; i--) // returns the number of current positions if(m_position.SelectByIndex(i)) // selects the position by index for further access to its properties { if(m_position.Time()<time) { time=m_position.Time(); ticket=m_position.Ticket(); } } //--- if(time!=D'2500.01.01 00:00') { m_trade.PositionModify(ticket,...); } //--- } //+------------------------------------------------------------------+
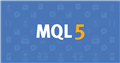
Documentation on MQL5: Constants, Enumerations and Structures / Trade Constants / Position Properties
- www.mql5.com
Position Properties - Trade Constants - Constants, Enumerations and Structures - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
I want to lower the TP of my first opened position by 10 pips, how would you do this?