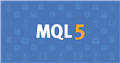
- www.mql5.com
- Error 131 when trying to publish a simple EA to the market.
- [WARNING CLOSED!] Any newbie question, so as not to clutter up the forum. Professionals, don't go by. Can't go anywhere without you.
- How to open a second order when the first reach some points in loss
Martingale, guaranteed to blow your account eventually. If it's not profitable without, it is definitely not profitable with.
Martingale vs. Non Martingale (Simplified RoR vs Profit and the Illusions) - MQL5 programming forum 2015.02.11
Why it won't work: Calculate Loss from Lot Pips - MQL5 programming forum 2017.07.11
Martingale, guaranteed to blow your account eventually. If it's not profitable without, it is definitely not profitable with.
Martingale vs. Non Martingale (Simplified RoR vs Profit and the Illusions) - MQL5 programming forum 2015.02.11
Why it won't work: Calculate Loss from Lot Pips - MQL5 programming forum 2017.07.11
Much appreciated your concern and advise. Because I would like to test it, but having the error, it's possible you could guide me about the problem mention above.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use