It is doing exactly what the specifier says. Only an int argument is a valid override of the base class method.
'CBar::func' method is declared with 'override' specifier, but does not override any base class method testscr.mq4 9 22
'CBar::func<short>' method is declared with 'override' specifier, but does not override any base class method testscr.mq4 9 22
'CBar::func<long>' method is declared with 'override' specifier, but does not override any base class method testscr.mq4 9 22
'CBar::func<string>' method is declared with 'override' specifier, but does not override any base class method testscr.mq4 9 22
'CBar::func<char>' method is declared with 'override' specifier, but does not override any base class method testscr.mq4 9 22
'CBar::func<datetime>' method is declared with 'override' specifier, but does not override any base class method testscr.mq4 9 22
'CBar::func<color>' method is declared with 'override' specifier, but does not override any base class method testscr.mq4 9 22
Do you see int in the errors?
Because of your trolling, snowflake rant and your vague question I am ignoring you as you requested
It is doing exactly what the specifier says. Only an int argument is a valid override of the base class method.
'CBar::func' method is declared with 'override' specifier, but does not override any base class method testscr.mq4 9 22
'CBar::func<short>' method is declared with 'override' specifier, but does not override any base class method testscr.mq4 9 22
'CBar::func<long>' method is declared with 'override' specifier, but does not override any base class method testscr.mq4 9 22
'CBar::func<string>' method is declared with 'override' specifier, but does not override any base class method testscr.mq4 9 22
'CBar::func<char>' method is declared with 'override' specifier, but does not override any base class method testscr.mq4 9 22
'CBar::func<datetime>' method is declared with 'override' specifier, but does not override any base class method testscr.mq4 9 22
'CBar::func<color>' method is declared with 'override' specifier, but does not override any base class method testscr.mq4 9 22
Do you see int in the errors?
Because of your trolling, snowflake rant and your vague question I am ignoring you as you requested
Do me a favor, and write me a class where the 'override' has been used successfully, without errors. Infact, I copy-pasted the classes from the documented examples.
I added 'override' because the compiler kept going for the method in the base class, without it, I get this:
deprecated behavior, hidden method calling will be disabled in a future MQL compiler version Code_it.mq5 31 9
How to avoid it? The docs say, "Use override." How? I just want to tell the compiler to use the method in the child class. That's all. If you can tell me how to do that, I'll be quiet.
Do me a favor, and write me a class where the 'override' has been used successfully, without errors. Infact, I copy-pasted the classes from the documented examples.
I added 'override' because the compiler kept going for the method in the base class, without it, I get this:
How to avoid it? The docs say, "Use override." How? I just want to tell the compiler to use the method in the child class. That's all. If you can tell me how to do that, I'll be quiet.
//+------------------------------------------------------------------+ //| Code_it.mq5 | //| Copyright 2020, Anonymous3. | //+------------------------------------------------------------------+ class CFoo { public: virtual void func(void) {} }; template <typename t> class CBar : public CFoo { protected: t x; public: void CBar(void) {} void CBar(t xx){ x = xx;} void func(void) { Print(x); } void functype(void) { Print(typename(x)); } }; //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnStart() { int val1 = 1; short val2 = 2; long val3 = 3; string val4 = "4"; char val5 = 5; datetime val6 = 6; color val7 = 7; CBar<int> theint; theint = new CBar<int>(val1); theint.func(); theint.functype(); CBar<short> theshort; theshort = new CBar<short>(val2); theshort.func(); theshort.functype(); CBar<long> thelong; thelong = new CBar<long>(val3); thelong.func(); thelong.functype(); CBar<string> *thestring; thestring = new CBar<string>(val4); thestring.func(); thestring.functype(); CBar<char> thechar; thechar = new CBar<char>(val5); thechar.func(); thechar.functype(); CBar<datetime> thedate; thedate = new CBar<datetime>(val6); thedate.func(); thedate.func(); CBar<color> thecolor; thecolor = new CBar<color>(val7); thecolor.func(); thecolor.functype(); //--- /* CBar<int> bar; bar = new CBar<int>(val1); bar.func(); /* bar.func(val2); bar.func(val3); bar.func(val4); bar.func(val5); bar.func(val6); bar.func(val7);*/ } //+------------------------------------------------------------------+
mql5
mql5
I'm interested in parameterised methods. I'm trying to figure our where and when the override specifier should be used. I'm debugging some unexpected behaviors in my code.
I know this is an old post, but it comes up early in google searches if you are looking for the answer to this question.
The problem with the basic documentation (https://www.mql5.com/en/docs/basis/oop/virtual) is that it does not show how to declare the variable containing the newly instantiated object (m_shape in the example used).
Also, the above response from Sardion uses the class CBar as the variable type containing the instantiated object, but really it would have been a better example to use CFoo.
The answer is found in this post (https://www.mql5.com/en/articles/351) where you must declare a variable as a pointer to the class, not just of the class itself. This I think is where the confusion is coming from.
A very simple example:
class Vehicle { public: virtual int NumWheels(void) { return 0; } }; class Car : public Vehicle { public: int NumWheels(void) override { return 4; } }; //--- inputs for expert input string Expert_Title = "ClassTest"; int OnInit() { Print("OnInit .. Starting Class Test..."); Vehicle *myCar = new Car; //--- NOT --- Vehicle myCar = new Car; myCar.NumWheels(); Print("Number of wheels: ", myCar.NumWheels()); return(INIT_SUCCEEDED); }
Hope this helps solve some of the confusion, and would be great if the documentation was also updated to show the variable declared as a pointer.
Regards.
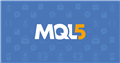
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Copy-paste the documented code and watch the compiler throw an error at you. The specifier does not do what it was created to do. AT ALL!!