Yes.
- Multi-Currency Expert Advisors in MT5 - backtesting and optimization - summary post #19
- Multicurrency strategy tester (MT5) - MT5 help file post #25
- and more here (All (not yet) about Strategy Tester, Optimization and Cloud)
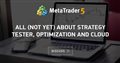
All (not yet) about Strategy Tester, Optimization and Cloud
- 2017.03.26
- www.mql5.com
General: All (not yet) about Strategy Tester, Optimization and Cloud
ffsss :
How exactly? just writing that pair on the order in the code right? i mean you dont need to do anything in the backtest option?
Here is a simple example: two symbols are set in the EA ('AUDJPY' and 'EURCAD'), and we run the test on 'GBPUSD'. And the advisor (working on GBPUSD) quietly opens positions on 'AUDJPY' and 'EURCAD'.
The Expert Advisor itself is given only as an example - it has neither Stop Loss nor Take Profit. No position closing - only one position will be opened.
Code: Example MultiSymbol EA.mq5
//+------------------------------------------------------------------+ //| Example MultiSymbol EA.mq5 | //| Copyright © 2020, Vladimir Karputov | //+------------------------------------------------------------------+ #property copyright "Copyright © 2020, Vladimir Karputov" #property version "1.000" /* barabashkakvn Trading engine 3.138 */ #include <Trade\PositionInfo.mqh> #include <Trade\Trade.mqh> //--- CPositionInfo m_position; // object of CPositionInfo class CTrade m_trade; // object of CTrade class //--- input parameters input group "Symbols" input string InpSymbol_0 = "AUDJPY"; // Symbol #0: Name input string InpSymbol_1 = "EURCAD"; // Symbol #1: Name input group "Additional features" input ulong InpDeviation = 10; // Deviation input ulong InpMagic = 200; // Magic number //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- initialize the generator of random numbers MathSrand(GetTickCount()); //--- m_trade.SetExpertMagicNumber(InpMagic); m_trade.SetMarginMode(); m_trade.SetTypeFillingBySymbol(Symbol()); m_trade.SetDeviationInPoints(InpDeviation); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- if(!IsPositionExists(InpSymbol_0)) { int math_rand=MathRand(); // value within the range of 0 to 32767 if(math_rand<32767/2) m_trade.Buy(1.0,InpSymbol_0); else m_trade.Sell(1.0,InpSymbol_0); } if(!IsPositionExists(InpSymbol_1)) { int math_rand=MathRand(); // value within the range of 0 to 32767 if(math_rand<32767/2) m_trade.Buy(1.0,InpSymbol_1); else m_trade.Sell(1.0,InpSymbol_1); } } //+------------------------------------------------------------------+ //| Is position exists | //+------------------------------------------------------------------+ bool IsPositionExists(const string symbol) { for(int i=PositionsTotal()-1; i>=0; i--) if(m_position.SelectByIndex(i)) // selects the position by index for further access to its properties if(m_position.Symbol()==symbol && m_position.Magic()==InpMagic) return(true); //--- return(false); } //+------------------------------------------------------------------+
Result
Files:

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hi, when you backtest you have to chose a pair for the backetest, but is it possible to buy or sell other currencies on this backtest. i dont know if i explained good enough.
Lets say i chose AUD/JPY and my indicator says buy, but i want the EA to buy on AUD/JPY and sell (or buy) on other pair (EUR/CAD) for a random example.
Is this possible on a backtest or not?