Jhonatan Breia Carvalho Maia :
Hi. I need some help. Im trying to do an average of OHCL in n periods. Thereis no error when i compile, but the indicator doesnt work. Could someone help me?
Prepare an indicator in the MQL5 Wizard - this is the only way to avoid mistakes.
The cat is the correct code:
//--- plot MA #property indicator_label1 "MA" #property indicator_type1 DRAW_COLOR_LINE #property indicator_color1 clrRed,clrLime #property indicator_style1 STYLE_SOLID #property indicator_width1 1 //--- input parameters input int InpPeriod = 2; int peso1 = 2; int peso2 = 3; int peso3 = 4; int peso4 = 5; double divisor = 1.618; //--- indicator buffers double MABufferSoma[]; double ColorBuffer[]; double MABuffer1[]; double MABuffer2[]; double MABuffer3[]; double MABuffer4[]; //+------------------------------------------------------------------+ int OnInit() { SetIndexBuffer(0,MABufferSoma,INDICATOR_DATA); SetIndexBuffer(1,ColorBuffer,INDICATOR_COLOR_INDEX); SetIndexBuffer(2,MABuffer1,INDICATOR_CALCULATIONS); SetIndexBuffer(3,MABuffer2,INDICATOR_CALCULATIONS); SetIndexBuffer(4,MABuffer3,INDICATOR_CALCULATIONS); SetIndexBuffer(5,MABuffer4,INDICATOR_CALCULATIONS); return(INIT_SUCCEEDED); }
Result:
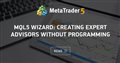
MQL5 Wizard: Creating Expert Advisors without Programming
- www.mql5.com
When you create automated trading systems it is necessary to write algorithms of analyzing market situation and generating trading signals, algorithms of trailing your open positions, as well as systems of money management and risk management. Once the modules' code is written the most difficult task is to assemble all parts and to debug the...
Vladimir Karputov:
Prepare an indicator in the MQL5 Wizard - this is the only way to avoid mistakes.
The cat is the correct code:
Result:
Thanks, it worked. As i can see, to do an indicator you really need to follow a restrict order in the code, dont you?

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hi. I need some help. Im trying to do an average of OHCL in n periods. Thereis no error when i compile, but the indicator doesnt work. Could someone help me?