- EA opening only BuY orders/ SELL NOT
- Buy and Sell Stop Orders
- Coding help
Hi Keith, i have created an Int to return the OrderSend value to, but still i have the same issue. Dont know if that is what you meant?
//open BuyStop int BuyStopOrderID; if (hourOfDay == 7 && minuteOfDay==59 && fastEMA>slowEMA && OrdersTotal() < 1) { BuyStopOrderID = OrderSend(Symbol(),OP_BUYSTOP,0.01,highestPrice, 20,highestPrice-200*Point,highestPrice+300*Point,NULL,0,TimeCurrent()+480*60,clrAqua); } else //open SellStop { int SellStopOrderID; if (hourOfDay == 7 && minuteOfDay==59 && fastEMA<slowEMA && OrdersTotal() < 1) { SellStopOrderID = OrderSend(Symbol(),OP_SELLSTOP,0.01,lowestPrice, 20,lowestPrice+200*Point,lowestPrice-300*Point,NULL,0,TimeCurrent()+480*60,clrAqua); } }
I tried to swop the buystop and sellstop OrderSend() function, so that the first bit of code is the sellstop and the second the buystop. Now the system only opens buy orders.. It seems that the first if statment gets ignored and the else statment always gets triggered, even though the trendfilter in the else statment is not correct.. I really cant see what is going wrong here, but must be something simple..
//open SellStop int SellStopOrderID; if(hourOfDay == 7 && minuteOfDay==59 && fastEMA>slowEMA && OrdersTotal() < 1) { SellStopOrderID = OrderSend(Symbol(),OP_SELLSTOP,0.01,lowestPrice, 20,lowestPrice+200*Point,lowestPrice-300*Point,NULL,0,TimeCurrent()+480*60,clrAqua); } else //open BuyStop { int BuyStopOrderID; if(hourOfDay == 7 && minuteOfDay==59 && fastEMA<slowEMA && OrdersTotal() < 1) { BuyStopOrderID = OrderSend(Symbol(),OP_BUYSTOP,0.01,highestPrice, 20,highestPrice-200*Point,highestPrice+300*Point,NULL,0,TimeCurrent()+480*60,clrAqua); } }
-
if (hourOfDay == 7 && minuteOfDay==59 &
This assumes that there will be a tick during that exact minute. There can be minutes between ticks during the Asian session, think M1 chart.
"Free-of-Holes" Charts - MQL4 Articles 20 June 2006
No candle if open = close ? - MQL4 programming forum 2010.06.06 -
&& OrdersTotal() < 1)
Magic number only allows an EA to identify its trades from all others. Using OrdersTotal/OrdersHistoryTotal (MT4) or PositionsTotal (MT5), directly and/or no Magic number filtering on your OrderSelect / Position select loop means your code is incompatible with every EA (including itself on other charts and manual trading.)
Symbol Doesn't equal Ordersymbol when another currency is added to another seperate chart . - MQL4 programming forum
PositionClose is not working - MQL5 programming forum
MagicNumber: "Magic" Identifier of the Order - MQL4 Articles
Orders, Positions and Deals in MetaTrader 5 - MQL5 Articles
Hi William, thank you for your inputs. I will implement a magic number.. For now though, do you see why the code skips the if-statement, and only reads the else-statement?
just to be clear about what i am trying to achieve: Basically i want to open either a sellstop or a buystop order at the set time. If its gonna be buy or sell is decided by a simple trendfilter with two EMA's. Thats the only difference between the two if statements. But for some reason the system only uses the second OrderSend() (the else statement). The first if statement (if-statement) is ignored, and even if the trendfilter shows an uptrend and the else-stetement needs a downtrend to be valid, the OrderSend function for a sellstop is still executed..
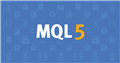
- www.mql5.com
hourOfDay == 7 && minuteOfDay==59 && fastEMA>slowEMA
//+------------------------------------------------------------------+ //| EA Asia Break.mq4 | //| Jesper | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Jesper" #property link "https://www.mql5.com" #property version "1.00" #property strict //trendfilter input string TRENDFILTER = "EMA Settings"; input int fastEMA = 50; input int slowEMA = 100; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { // Get high and low of range double highestPrice = iHigh(NULL,0,iHighest(NULL,0,MODE_HIGH,8,0)); double lowestPrice= iLow(NULL,0,iLowest(NULL,0,MODE_LOW,8,0)); Alert (highestPrice); Alert (lowestPrice); // set pending order at high and low int hourOfDay = Hour(); int minuteOfDay = Minute(); //open BuyStop int BuyStopOrderID; if(hourOfDay == 7 && minuteOfDay==59 && fastEMA>slowEMA && OrdersTotal() < 1) { BuyStopOrderID = OrderSend(Symbol(),OP_BUYSTOP,0.01,highestPrice, 20,highestPrice-200*Point,highestPrice+300*Point,NULL,0,TimeCurrent()+480*60,clrAqua); } else //open SellStop { int SellStopOrderID; if(hourOfDay == 7 && minuteOfDay==59 && fastEMA<slowEMA && OrdersTotal() < 1) { SellStopOrderID = OrderSend(Symbol(),OP_SELLSTOP,0.01,lowestPrice, 20,lowestPrice+200*Point,lowestPrice-300*Point,NULL,0,TimeCurrent()+480*60,clrAqua); } } } //+------------------------------------------------------------------+
input int fastEMA = 50; input int slowEMA = 100; ⋮ if(hourOfDay == 7 && minuteOfDay==59 && fastEMA<slowEMA && OrdersTotal() < 1)
50 is always less than 100.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use