You make a grave mistake! You create an indicator handle AT EVERY TICK! The indicator handle HAS TO BE CREATED ONCE (the best place for this is OnInit).
An example of correctly creating handles: Creating an iMA indicator handle, getting indicator values
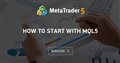
- 2020.03.05
- www.mql5.com
You make a grave mistake! You create an indicator handle AT EVERY TICK! The indicator handle HAS TO BE CREATED ONCE (the best place for this is OnInit).
An example of correctly creating handles: Creating an iMA indicator handle, getting indicator values
ой ... мой плохой, так что мне просто нужно переместить весь код внутри OnTick?
Check out my example first. The indicator handle MUST BE CREATED ONCE (in OnInit). And the trading functions are over, they are needed in OnTick.
The main thing to remember: the handle of the indicator SHOULD BE CREATED ONCE (in OnInit).
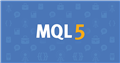
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi there, Complete beginner here...
so a few weeks ago I'm trying to make a simple Crossover MA EA using MQL5, everything seems fine and I got no error message, but after I try it on a demo account, I start having a problem.
so I want to make my EA open only one position for each market/pair, but can open several positions if there is a signal on another pair.
for example, let's say it's open a buy position on EUR/USD, I don't want my EA to open the same position again on the same pair, instead, only open a new position if there is a signal on another market (for example USD/JPY).
For now, what my EA does is opening 5 different entry on the same signal, even when there is another signal on another pair.
here is the code, I hope you guys can help me out with this, Thanks, and sorry for bad English