francesco.re1107:
Hi,
I'm trying to use this library for python.
I've successfully connected to my account and placed an order with this code:
But when I want to close that position with the following code, it gives me 10013 error, which means Invalid request
I can't figure out how to close positions. Also the docs is not explanatory.
I would like also to close positions partially.
Thanks
You have to add the position_id (position ticket number) to the 'position' key.
import pymt5adapter as mt5 def send_order(req, n=5): for _ in range(n): res = mt5.order_send(req) if res.retcode == mt5.TRADE_RETCODE_DONE: break return res def main(): symbol = mt5.symbols_get(function=lambda s: s.trade_mode == mt5.SYMBOL_TRADE_MODE_FULL)[0] buy_order = dict( action=mt5.TRADE_ACTION_DEAL, type=mt5.ORDER_TYPE_BUY, symbol=symbol.name, price=mt5.symbol_info_tick(symbol.name).ask, volume=symbol.volume_min, deviation=100, ) result = send_order(buy_order) print(result) if result.retcode == mt5.TRADE_RETCODE_DONE: deal = mt5.history_deals_get(ticket=result.deal)[0] position = mt5.positions_get(ticket=deal.position_id)[0] close_order = dict( action=mt5.TRADE_ACTION_DEAL, type=mt5.ORDER_TYPE_SELL, price=mt5.symbol_info_tick(symbol.name).bid, symbol=position.symbol, volume=position.volume, position=position.ticket, ) result = send_order(close_order) print(result) if __name__ == "__main__": with mt5.connected(): main()
On a side note you can also try the pymt5adapter package. https://pypi.org/project/pymt5adapter/
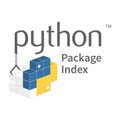
pymt5adapter
- 2020.04.06
- pypi.org
is a wrapper and drop-in replacement (wrapper) for the python package by MetaQuotes. The API functions simply pass through values from the functions, but adds the following functionality in addition to a more pythonic interface: Typing hinting has been added to all functions and return objects for linting and IDE integration. Now intellisense...

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hi,
I'm trying to use this library for python.
I've successfully connected to my account and placed an order with this code:
But when I want to close that position with the following code, it gives me 10013 error, which means Invalid request
I can't figure out how to close positions. Also the docs is not explanatory.
I would like also to close positions partially.
Thanks