To use the MQL5 Wizard, you need to use the trading signal modules. Here is a module: SignalCrossiMA
Based on the article, the MQL5 Wizard - Trading Signals at the Intersection of Two Exponential Moving Averages - now the signal module works in the new version of the terminal.
The signal file must be placed in the [data folder] \ MQL5 \ Include \ Expert \ Signal \ folder and restart the MetaEditor editor. After a reboot, the editor will see this module (look for the description " Signals based on crossover of two iMA ").
Next, you need to generate an adviser based on the signal module - for more details, see Help creating a ready-made advisor - MQL4 / MQL5 Wizard .
Only two conditions are left: for opening BUY ( LongCondition ) and for opening SELL ( ShortCondition )
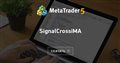
- www.mql5.com
To use the MQL5 Wizard, you need to use the trading signal modules. Here is a module: SignalCrossiMA
Thank You :)
hi again i cant find this signal in my editor
Only this signals
The signal file must be placed in the [data folder] \MQL5\Include\Expert\Signal\folder and restart the MetaEditor editor. After a reboot, the editor will see this module (look for the description " Signals based on crossover of two iMA ").
The signal file must be placed in the [data folder] \MQL5\Include\Expert\Signal\folder and restart the MetaEditor editor. After a reboot, the editor will see this module (look for the description " Signals based on crossover of two iMA ").
hi
I did as you said and its not there is there any way to downloaded or imported to my signals folder
hi
I did as you said and its not there is there any way to downloaded or imported to my signals folder
I repeat to you for the third time:
Here is a module: SignalCrossiMA. The signal file must be placed in the [data folder]\MQL5\Include\Expert\Signal\folder and the MetaEditor editor reloaded. After a reboot, the editor will see this module (look for the description " Signals based on crossover of two iMA ").
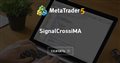
- www.mql5.com
I repeat to you for the third time:
Here is a module: SignalCrossiMA. The signal file must be placed in the [data folder]\MQL5\Include\Expert\Signal\folder and the MetaEditor editor reloaded. After a reboot, the editor will see this module (look for the description " Signals based on crossover of two iMA").
oh yea Sorry my man new at this got it thanks.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello I need to Know how to edit this code with Examples to make it crossover EA.