Basically, I want my EA to manage open trades with OrderModify().
My EA is programmed to take trades when 2 Moving Averages are crossing. Every time the 2 Moving Averages cross, it opens 2 trades.
Trade 1:
Take Profit = 1.0 * ATR Stop Loss = 1.5 * ATR
Trade 2:
No Take Profit Stop Loss = 1.5 * ATR
When price hits Take Profit on that one trade, I want my EA to simply move Stop Loss to Breakeven on the other trade.
It works sometimes, and sometimes it doesn't.
For some reason, the EA keeps modifying some of the Orders 100 times, moving the Stop Loss back and forward a couple of pips.
I don't see what is wrong in the code.. Have anyone ever experienced the same? Does anyone know what is wrong?
All feedback is greatly appreciated!
Focusing on the buys (same comments should apply for the sells):
(1) in the green block below, BuyOrderTP will not be getting any valid value once the order with TP is closed, so you need to devise a better way to capture the TP price for checking in your yellow block.
(2) Another big issue is of OrderSelect() - you cannot assume that '0' returns you the latest or earliest order, so going through the 'for' loop is necessary whenever you have more than one orders. However, using '0' within the yellow block may be fine if you also make sure that OrdersTotal()==1, with the order type and magic number checks already in place.
//For Buy Orders { int BuyOrderTP; //for(int b1=OrdersTotal()-1;b1>=0;b1--) { if(OrderSelect(0,SELECT_BY_POS,MODE_TRADES)) if(OrderMagicNumber()==1) if(OrderType()==OP_BUY) BuyOrderTP=OrderTakeProfit(); } //for(int b=OrdersTotal()-1;b>=0;b--) { if(OrderSelect(0,SELECT_BY_POS,MODE_TRADES)) if(OrderMagicNumber()==2) if(OrderType()==OP_BUY) if(Ask>BuyOrderTP) if(OrderOpenPrice()>OrderStopLoss()); OrderModify(OrderTicket(),OrderOpenPrice(),OrderOpenPrice()+(PipsLockedIn*pips),OrderTakeProfit(),0,clrGreen); } }
Focusing on the buys (same comments should apply for the sells):
(1) in the green block below, BuyOrderTP will not be getting any valid value once the order with TP is closed, so you need to devise a better way to capture the TP price for checking in your yellow block.
(2) Another big issue is of OrderSelect() - you cannot assume that '0' returns you the latest or earliest order, so going through the 'for' loop is necessary whenever you have more than one orders. However, using '0' within the yellow block may be fine if you also make sure that OrdersTotal()==1, with the order type and magic number checks already in place.
Thanks a lot for your reply! Appreciate the help!
I'm sad to hear that this solution doesn't work...
Do you know a better way to capture the TP price?
Btw, I only use OpenPrice when backtesting with optimization.
I fixed the OrderSelect() problem! Thanks!
Do you know a better way to capture the TP price?
You have many choices :), such as:
(1) draw an object on the chart to keep track of the TP value (e.g. horizontal line at the TP price), whenever you open an order with TP. This way, you can simply read that horizontal line for the TP value. (Opens up new possibilities, such as allowing you to change the TP value easily).
(2) store the TP value as https://book.mql4.com/variables/globals. (Simplest).
(3) loop through orders, including closed ones, to get the TP value of the most recently closed order. (Most robust).
(4) write that value to a file. (Overkill).
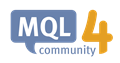
- book.mql4.com
You have many choices :), such as:
(1) draw an object on the chart to keep track of the TP value (e.g. horizontal line at the TP price), whenever you open an order with TP. This way, you can simply read that horizontal line for the TP value. (Opens up new possibilities, such as allowing you to change the TP value easily).
(2) store the TP value as https://book.mql4.com/variables/globals. (Simplest).
(3) loop through orders, including closed ones, to get the TP value of the most recently closed order. (Most robust).
(4) write that value to a file. (Overkill).
Thanks! I found out!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Basically, I want my EA to manage open trades with OrderModify().
My EA is programmed to take trades when 2 Moving Averages are crossing. Every time the 2 Moving Averages cross, it opens 2 trades.
Trade 1:
Take Profit = 1.0 * ATR Stop Loss = 1.5 * ATR
Trade 2:
No Take Profit Stop Loss = 1.5 * ATR
When price hits Take Profit on that one trade, I want my EA to simply move Stop Loss to Breakeven on the other trade.
It works sometimes, and sometimes it doesn't.
For some reason, the EA keeps modifying some of the Orders 100 times, moving the Stop Loss back and forward a couple of pips.
I don't see what is wrong in the code.. Have anyone ever experienced the same? Does anyone know what is wrong?
All feedback is greatly appreciated!