You can find it out yourself quite easily!
Just go with the debugger (F5) through your code and let the debugger show the various values even the time of time[bar] and at the very top _LastError!
You can find it out yourself quite easily!
Just go with the debugger (F5) through your code and let the debugger show the various values even the time of time[bar] and at the very top _LastError!
Thanks @Carl Schreiber for your answer...
I cannot see any error through debugging ...
The idea of using the debugger, is to step through your code, line by line, and see how the variables change. For example, when you step over this line : " CopyBuffer( MA_Handle_1m,0,0,1,MA_Array_1m );", you can add "MA_Array_10m[0]" to watch and see how or if the value changes.
But first, I suggest some code shifting, split this segment:
int MA_Handle_1m = iMA(_Symbol,PERIOD_M1,MAPeriod,0,MODE_SMA,PRICE_CLOSE); int MA_Handle_5m = iMA(_Symbol,PERIOD_M5,MAPeriod,0,MODE_SMA,PRICE_CLOSE); int MA_Handle_10m = iMA(_Symbol,PERIOD_M10,MAPeriod,0,MODE_SMA,PRICE_CLOSE); int MA_Handle_15m = iMA(_Symbol,PERIOD_M15,MAPeriod,0,MODE_SMA,PRICE_CLOSE); int MA_Handle_30m = iMA(_Symbol,PERIOD_M30,MAPeriod,0,MODE_SMA,PRICE_CLOSE); int MA_Handle_1h = iMA(_Symbol,PERIOD_H1,MAPeriod,0,MODE_SMA,PRICE_CLOSE);
into two parts, with first part (as follows) in the global declaration part:
int MA_Handle_1m,MA_Handle_5m,MA_Handle_10m,MA_Handle_15m,MA_Handle_30m,MA_Handle_1h;
and these into the OnInit() function:
MA_Handle_1m = iMA(_Symbol,PERIOD_M1,MAPeriod,0,MODE_SMA,PRICE_CLOSE); MA_Handle_5m = iMA(_Symbol,PERIOD_M5,MAPeriod,0,MODE_SMA,PRICE_CLOSE); MA_Handle_10m = iMA(_Symbol,PERIOD_M10,MAPeriod,0,MODE_SMA,PRICE_CLOSE); MA_Handle_15m = iMA(_Symbol,PERIOD_M15,MAPeriod,0,MODE_SMA,PRICE_CLOSE); MA_Handle_30m = iMA(_Symbol,PERIOD_M30,MAPeriod,0,MODE_SMA,PRICE_CLOSE); MA_Handle_1h = iMA(_Symbol,PERIOD_H1,MAPeriod,0,MODE_SMA,PRICE_CLOSE);
because you need to obtain the handles of the indicators just once, which will also initiate computation. Then in OnCalculate, you just use CopyBuffer to obtain the computed values of specific bars.
Then fire up your debugger and check how the variables change over time (*hint* your 'bar' variable doesn't seem to be used fruitfully, and don't forget that the same 'bar' cannot be used across different timeframe, except bar 0 which refers to the latest non-complete bar, if that is what you want).
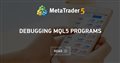
- www.mql5.com
Hello Everyone,
By ready the instructions of this tutorial I tried to sum SMA's of different Time Frames inside to one new indicator and I take current value but the line is straight (not assembled to the previous bars).
The code to set up is this
and the code to calculate is this:
What i am doing wrong ?
For sure, the line is straight, you are always copying the same values :
CopyBuffer( MA_Handle_1m,0,0,1,MA_Array_1m ); CopyBuffer( MA_Handle_5m,0,0,1,MA_Array_5m ); CopyBuffer( MA_Handle_10m,0,0,1,MA_Array_10m ); CopyBuffer( MA_Handle_15m,0,0,1,MA_Array_15m ); CopyBuffer( MA_Handle_30m,0,0,1,MA_Array_30m ); CopyBuffer( MA_Handle_1h,0,0,1,MA_Array_1h );
Anyway, you will need to synchronize your values between timeframes.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello Everyone,
By ready the instructions of this tutorial I tried to sum SMA's of different Time Frames inside to one new indicator and I take current value but the line is straight (not assembled to the previous bars).
The code to set up is this
and the code to calculate is this:
What i am doing wrong ?