Daniel Cardoso:
We have tons of EA's around, but never with a smart SL and TP. My point is simple. When we place a trade, to place your SL you'll do one of these two options:
Use support and resistant previews levels, or.... chose a number of candle (e.g. the last 5 or 10 candles) and pick the lower low (in case of long trades) and lower lows (in case of short trades). My best trading system is made with HMA in the current chart to spot good entries and another HMA in a higher time frame, to pick the trend, and then this "adaptative" SL and TP. I know I little bit of coding, but have no time to work on this... if you're trying your coding skills, feel free to help us :)
We have tons of EA's around, but never with a smart SL and TP. My point is simple. When we place a trade, to place your SL you'll do one of these two options:
Use support and resistant previews levels, or.... chose a number of candle (e.g. the last 5 or 10 candles) and pick the lower low (in case of long trades) and lower lows (in case of short trades). My best trading system is made with HMA in the current chart to spot good entries and another HMA in a higher time frame, to pick the trend, and then this "adaptative" SL and TP. I know I little bit of coding, but have no time to work on this... if you're trying your coding skills, feel free to help us :)
Let me know how this works out for you.
//+------------------------------------------------------------------+ //| AdaptiveTargets.mq4 | //| nicholishen | //| https://www.forexfactory.com/nicholishen | //+------------------------------------------------------------------+ #property copyright "nicholishen" #property link "https://www.forexfactory.com/nicholishen" #property version "1.00" #property strict #include <stdlib.mqh> //--- input parameters input int inp_points_beyond=0;//Points beyond extremum input int inp_bars_extremum=5;//Number of bars to find extremums //+------------------------------------------------------------------+ int OnInit(){return(INIT_SUCCEEDED);} //+------------------------------------------------------------------+ void OnTick() { for(int i=OrdersTotal()-1; i>=0; --i){ if(OrderSelect(i, SELECT_BY_POS) && OrderSymbol() == _Symbol && OrderType() < 2){ double sl, tp; if(OrderType() == OP_BUY){ sl = roundtick(Low[ArrayMinimum(Low, inp_bars_extremum, 1)] - inp_points_beyond * _Point); tp = roundtick(High[ArrayMaximum(High, inp_bars_extremum, 1)] + inp_points_beyond * _Point); }else{ tp = roundtick(Low[ArrayMinimum(Low, inp_bars_extremum, 1)] - inp_points_beyond * _Point); sl = roundtick(High[ArrayMaximum(High, inp_bars_extremum, 1)] + inp_points_beyond * _Point); } if(!CompareDoubles(OrderStopLoss(), sl) || !CompareDoubles(OrderTakeProfit(), tp)){ if(!OrderModify(OrderTicket(), OrderOpenPrice(), sl, tp, OrderExpiration(), clrYellow)){ Print("OrderModifyError: ", ErrorDescription(GetLastError())); } } } } } //+------------------------------------------------------------------+ double roundtick(double num) { static double step = DBL_MAX; if(step == DBL_MAX) step = SymbolInfoDouble(_Symbol, SYMBOL_TRADE_TICK_SIZE); return step * round(num / step); }
Nice algo @nicholi shen .
I have to ask about the roundtick function . what is its purpose
I have to ask about the roundtick function . what is its purpose
Lorentzos Roussos:
Nice algo @nicholi shen .
I have to ask about the roundtick function . what is its purpose
https://www.mql5.com/pt/docs/standardlibrary/tradeclasses/csymbolinfo/csymbolinfonormalizepriceNice algo @nicholi shen .
I have to ask about the roundtick function . what is its purpose
nicholi shen:
Let me know how this works out for you.
https://www.mql5.com/pt/docs/standardlibrary/tradeclasses/csymbolinfo/csymbolinfonormalizeprice
#include <Trade\SymbolInfo.mqh>
CSymbolInfo _CSymbolInfo;
_CSymbolInfo.NormalizePrice(price);
Or simply copy and paste NormalizePrice from SymbolInfo.mqh.
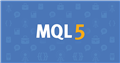
Documentação sobre MQL5: Biblioteca Padrão / Classes de negociação / CSymbolInfo / NormalizePrice
- www.mql5.com
Biblioteca Padrão / Classes de negociação / CSymbolInfo / NormalizePrice - Referência sobre algorítimo/automatização de negociação na linguagem para MetaTrader 5
Arthur Albano:
https://www.mql5.com/pt/docs/standardlibrary/tradeclasses/csymbolinfo/csymbolinfonormalizeprice
https://www.mql5.com/pt/docs/standardlibrary/tradeclasses/csymbolinfo/csymbolinfonormalizeprice
https://www.mql5.com/pt/docs/standardlibrary/tradeclasses/csymbolinfo/csymbolinfonormalizeprice
Or simply copy and paste NormalizePrice from SymbolInfo.mqh.
//+------------------------------------------------------------------+ //| AdaptiveTargets.mq4 | //| nicholishen | //| https://www.forexfactory.com/nicholishen | //+------------------------------------------------------------------+
Nice algo @nicholi shen .
I have to ask about the roundtick function . what is its purpose
It rounds to the tick-size of the instrument. It's common for CFDs to have a different tick-size than the "Point". If the prices aren't rounded it could fail on error.
I have to ask about the roundtick function . what is its purpose

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Use support and resistant previews levels, or.... chose a number of candle (e.g. the last 5 or 10 candles) and pick the lower low (in case of long trades) and lower lows (in case of short trades). My best trading system is made with HMA in the current chart to spot good entries and another HMA in a higher time frame, to pick the trend, and then this "adaptative" SL and TP. I know I little bit of coding, but have no time to work on this... if you're trying your coding skills, feel free to help us :)