You start to draw from the previous bar and continue drawing to the current bar.
This means that for time1 you need one bar up or older.
Thanks ill try it and give feedback
time1 is where the trendlines starts and time2 parameter is where it stops.
So if you have a price value you can start to draw at the current bar + 1 which is one bar older then the current bar and end drawing at the current bar.
You can also draw to the next bar that is bar -1 but you will run into problems when trying to draw bar 0 because bar 0 -1 minus one is -1 and your line will connect to 1970.
But this is for drawing in experts.
In indicators you only have to write your price value to a indicator buffer and it will show up just fine.
so you have to replace the
bool TrendCreate()
function with an indicator buffer and fill it's value with the desired price reading.
have you tried to generate a indicator template ?
You can specify number of buffers and needed parameters and it will generate a nice template for you.
//+------------------------------------------------------------------+ //| Template.mq5 | //| Copyright 2018,Marco vd Heijden,MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2018,Marco vd Heijden,MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #property indicator_separate_window #property indicator_buffers 1 #property indicator_plots 1 //--- plot Label1 #property indicator_label1 "Label1" #property indicator_type1 DRAW_LINE #property indicator_color1 clrRed #property indicator_style1 STYLE_SOLID #property indicator_width1 1 //--- input parameters input int parameter_1=80; input int parameter_2=20; //--- indicator buffers double Label1Buffer[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping SetIndexBuffer(0,Label1Buffer,INDICATOR_DATA); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- int limit; if(prev_calculated==0) { limit=0; } else { limit=prev_calculated-1; } //--- calculate value for(int i=limit;i<rates_total && !IsStopped();i++) { Label1Buffer[i]=open[i];// <-- assign some value to the buffer here, in this case just the open price } //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+
I took the time to generate a very simple example for you so easy to understand because it will just fill the buffer with the open price.
Of course you can do that, it was designed to do that.
Just add more buffers.
Here is a quick and simple Donchian Channel example with 3 Buffers:
//+------------------------------------------------------------------+ //| Donchian-reversed.mq5 | //| Copyright 2018,Marco vd Heijden,MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2018,Marco vd Heijden,MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #property indicator_chart_window #property indicator_buffers 3 #property indicator_plots 3 //--- plot High #property indicator_label1 "High" #property indicator_type1 DRAW_LINE #property indicator_color1 clrAliceBlue #property indicator_style1 STYLE_SOLID #property indicator_width1 1 //--- plot Center #property indicator_label2 "Center" #property indicator_type2 DRAW_LINE #property indicator_color2 clrYellow #property indicator_style2 STYLE_SOLID #property indicator_width2 1 //--- plot Low #property indicator_label3 "Low" #property indicator_type3 DRAW_LINE #property indicator_color3 clrAliceBlue #property indicator_style3 STYLE_SOLID #property indicator_width3 1 //--- input parameters input int period=20;// Period //--- indicator buffers double HighBuffer[]; double CenterBuffer[]; double LowBuffer[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping SetIndexBuffer(0,HighBuffer,INDICATOR_DATA); SetIndexBuffer(1,CenterBuffer,INDICATOR_DATA); SetIndexBuffer(2,LowBuffer,INDICATOR_DATA); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- int limit; if(prev_calculated==0) { limit=0; } else { limit=prev_calculated-1; } //--- calculate values for(int i=limit;i<rates_total && !IsStopped();i++) { //--- high HighBuffer[i]=high[ArrayMaximum(high,i,period)]; //--- low LowBuffer[i]=low[ArrayMinimum(low,i,period)]; //--- center CenterBuffer[i]=(HighBuffer[i]+LowBuffer[i])/2; } //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+
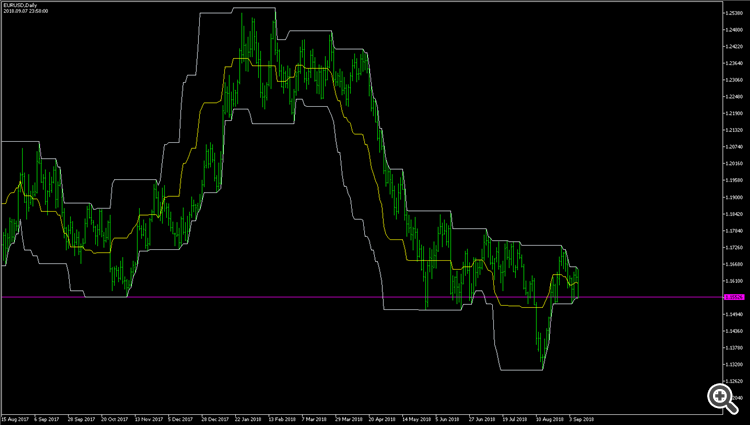
Thanks !
I appreciate it
But is this achievable this way !
its quite different from what you have sent
with what code did you produce this chart ? This is exactly what i'm looking for :) Would you share ?
with what code did you produce this chart ?
I havent produced it yet, its what im aiming for ! I found it as image from a friend

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello,
Im having some trouble building trend lines around a RSI indicator - what seems to be the problem is the "time" coordinate for setting lines. The result is :
This is the first time i build such indicator !
Hope you can help ! The code is below :