Probably not, but you can make your own hotkeys that activate the default.
thank you. how is it possible? I can figure out only personal hotkeys for EAs and indicators
https://www.mql5.com/en/docs/constants/chartconstants/enum_chartevents
#define KEY_NUMPAD_5 12 #define KEY_LEFT 37 #define KEY_UP 38 #define KEY_RIGHT 39 #define KEY_DOWN 40 #define KEY_NUMLOCK_DOWN 98 #define KEY_NUMLOCK_LEFT 100 #define KEY_NUMLOCK_5 101 #define KEY_NUMLOCK_RIGHT 102 #define KEY_NUMLOCK_UP 104 //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- Print("The expert with name ",MQL5InfoString(MQL5_PROGRAM_NAME)," is running"); //--- enable object create events ChartSetInteger(ChartID(),CHART_EVENT_OBJECT_CREATE,true); //--- enable object delete events ChartSetInteger(ChartID(),CHART_EVENT_OBJECT_DELETE,true); //--- forced updating of chart properties ensures readiness for event processing ChartRedraw(); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| ChartEvent function | //+------------------------------------------------------------------+ void OnChartEvent(const int id, // Event identifier const long& lparam, // Event parameter of long type const double& dparam, // Event parameter of double type const string& sparam // Event parameter of string type ) { //--- the left mouse button has been pressed on the chart if(id==CHARTEVENT_CLICK) { Print("The coordinates of the mouse click on the chart are: x = ",lparam," y = ",dparam); } //--- the mouse has been clicked on the graphic object if(id==CHARTEVENT_OBJECT_CLICK) { Print("The mouse has been clicked on the object with name '"+sparam+"'"); } //--- the key has been pressed if(id==CHARTEVENT_KEYDOWN) { switch(lparam) { case KEY_NUMLOCK_LEFT: Print("The KEY_NUMLOCK_LEFT has been pressed"); break; case KEY_LEFT: Print("The KEY_LEFT has been pressed"); break; case KEY_NUMLOCK_UP: Print("The KEY_NUMLOCK_UP has been pressed"); break; case KEY_UP: Print("The KEY_UP has been pressed"); break; case KEY_NUMLOCK_RIGHT: Print("The KEY_NUMLOCK_RIGHT has been pressed"); break; case KEY_RIGHT: Print("The KEY_RIGHT has been pressed"); break; case KEY_NUMLOCK_DOWN: Print("The KEY_NUMLOCK_DOWN has been pressed"); break; case KEY_DOWN: Print("The KEY_DOWN has been pressed"); break; case KEY_NUMPAD_5: Print("The KEY_NUMPAD_5 has been pressed"); break; case KEY_NUMLOCK_5: Print("The KEY_NUMLOCK_5 has been pressed"); break; default: Print("Some not listed key has been pressed"); } ChartRedraw(); } //--- the object has been deleted if(id==CHARTEVENT_OBJECT_DELETE) { Print("The object with name ",sparam," has been deleted"); } //--- the object has been created if(id==CHARTEVENT_OBJECT_CREATE) { Print("The object with name ",sparam," has been created"); } //--- the object has been moved or its anchor point coordinates has been changed if(id==CHARTEVENT_OBJECT_DRAG) { Print("The anchor point coordinates of the object with name ",sparam," has been changed"); } //--- the text in the Edit of object has been changed if(id==CHARTEVENT_OBJECT_ENDEDIT) { Print("The text in the Edit field of the object with name ",sparam," has been changed"); } }
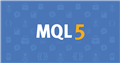
- www.mql5.com
This from a code I'm using:
#import "user32.dll" void keybd_event(int bVk, int bScan, int dwFlags, int dwExtraInfo); int GetParent(long hWnd); bool SetWindowPos(long hWnd, long hWndInsertAfter, int x, int y, int width, int height, uint uFlags); #import void OnChartEvent(const int id, const long& lparam, const double& dparam, const string& sparam ) { if(id==CHARTEVENT_KEYDOWN) { if(lparam==49) NumberOne(); if(lparam==50) NumberTwo(); if(lparam==51) PlusMinus(); if(lparam==192) ChartRescale(); if(lparam==219) TimeFrameDown(); if(lparam==221) TimeFrameUp(); if(lparam==222) TimeFrameInTheMiddle(); } ChartRedraw(); } void NumberOne() { if(ChartGetInteger(0,CHART_IS_MAXIMIZED,0)==1) { keybd_event(0x12,0,0,0); keybd_event(0x52,0,0,0); keybd_event(0x12,0,0x0002,0); keybd_event(0x52,0,0x0002,0); } long FirstChartID = ChartFirst(); long SecondChartID = ChartNext(FirstChartID); long ThirdChartID = ChartNext(SecondChartID); long FourthChartID = ChartNext(ThirdChartID); long FirstChartHandle, SecondChartHandle, ThirdChartHandle, FourthChartHandle; long FirstChartParentHandle, SecondChartParentHandle, ThirdChartParentHandle, FourthChartParentHandle; ChartGetInteger(FirstChartID,CHART_WINDOW_HANDLE,0,FirstChartHandle); ChartGetInteger(SecondChartID,CHART_WINDOW_HANDLE,0,SecondChartHandle); ChartGetInteger(ThirdChartID,CHART_WINDOW_HANDLE,0,ThirdChartHandle); ChartGetInteger(FourthChartID,CHART_WINDOW_HANDLE,0,FourthChartHandle); FirstChartParentHandle=GetParent(FirstChartHandle); SecondChartParentHandle=GetParent(SecondChartHandle); ThirdChartParentHandle=GetParent(ThirdChartHandle); FourthChartParentHandle=GetParent(FourthChartHandle); SetWindowPos(FourthChartParentHandle,0,1288,0,640,958,0); SetWindowPos(ThirdChartParentHandle,0,900,0,640,958,0); SetWindowPos(SecondChartParentHandle,0,450,0,640,958,0); SetWindowPos(FirstChartParentHandle,0,0,0,620,958,0); } void NumberTwo() { if(ChartGetInteger(0,CHART_IS_MAXIMIZED,0)==1) { keybd_event(0x12,0,0,0); keybd_event(0x52,0,0,0); keybd_event(0x12,0,0x0002,0); keybd_event(0x52,0,0x0002,0); } long ThisChartID = ChartID(); long FirstChartID = ChartFirst(); long SecondChartID = ChartNext(FirstChartID); long ThirdChartID = ChartNext(SecondChartID); long FourthChartID = ChartNext(ThirdChartID); long FirstChartHandle, SecondChartHandle, ThirdChartHandle, FourthChartHandle; long FirstChartParentHandle, SecondChartParentHandle, ThirdChartParentHandle, FourthChartParentHandle; ChartGetInteger(FirstChartID,CHART_WINDOW_HANDLE,0,FirstChartHandle); ChartGetInteger(SecondChartID,CHART_WINDOW_HANDLE,0,SecondChartHandle); ChartGetInteger(ThirdChartID,CHART_WINDOW_HANDLE,0,ThirdChartHandle); ChartGetInteger(FourthChartID,CHART_WINDOW_HANDLE,0,FourthChartHandle); FirstChartParentHandle=GetParent(FirstChartHandle); SecondChartParentHandle=GetParent(SecondChartHandle); ThirdChartParentHandle=GetParent(ThirdChartHandle); FourthChartParentHandle=GetParent(FourthChartHandle); if(ThisChartID==FirstChartID) { SetWindowPos(FourthChartParentHandle,0,1608,0,320,958,0); SetWindowPos(ThirdChartParentHandle,0,1440,0,320,958,0); SetWindowPos(SecondChartParentHandle,0,1280,0,320,958,0); SetWindowPos(FirstChartParentHandle,0,0,0,1440,958,0); } if(ThisChartID==SecondChartID) { SetWindowPos(FourthChartParentHandle,0,1608,0,320,958,0); SetWindowPos(ThirdChartParentHandle,0,1440,0,320,958,0); SetWindowPos(FirstChartParentHandle,0,0,0,320,958,0); SetWindowPos(SecondChartParentHandle,0,320,0,1280,958,0); } if(ThisChartID==ThirdChartID) { SetWindowPos(FourthChartParentHandle,0,1608,0,320,958,0); SetWindowPos(SecondChartParentHandle,0,180,0,240,958,0); SetWindowPos(FirstChartParentHandle,0,0,0,240,958,0); SetWindowPos(ThirdChartParentHandle,0,420,0,1280,958,0); } if(ThisChartID==FourthChartID) { SetWindowPos(ThirdChartParentHandle,0,252,0,240,958,0); SetWindowPos(SecondChartParentHandle,0,100,0,240,958,0); SetWindowPos(FirstChartParentHandle,0,0,0,200,958,0); SetWindowPos(FourthChartParentHandle,0,488,0,1440,958,0); } } void PlusMinus() { if(ChartGetInteger(0,CHART_SCALE)<4) ChartSetInteger(0,CHART_SCALE,4); else ChartSetInteger(0,CHART_SCALE,3); ChartRedraw(); keybd_event(0x23,0,0,0); keybd_event(0x23,0,0x0002,0); } void ChartRescale() { ChartSetDouble(0,CHART_FIXED_MIN,0); ChartSetDouble(0,CHART_FIXED_MAX,2*SymbolInfoDouble(Symbol(),SYMBOL_BID)); ChartRedraw(); keybd_event(0x65,0,0,0); keybd_event(0x65,0,0x0002,0); } void TimeFrameUp() { ENUM_TIMEFRAMES timeframe; switch(_Period) { case PERIOD_M1: timeframe=PERIOD_M2; break; case PERIOD_M2: timeframe=PERIOD_M3; break; case PERIOD_M3: timeframe=PERIOD_M5; break; case PERIOD_M5: timeframe=PERIOD_M10; break; case PERIOD_M10: timeframe=PERIOD_M15; break; case PERIOD_M15: timeframe=PERIOD_M30; break; case PERIOD_M30: timeframe=PERIOD_H1; break; case PERIOD_H1: timeframe=PERIOD_H4; break; case PERIOD_H4: timeframe=PERIOD_D1; break; case PERIOD_D1: timeframe=PERIOD_W1; break; case PERIOD_W1: timeframe=PERIOD_MN1; break; default: timeframe=PERIOD_M3; } ChartSetSymbolPeriod(0,_Symbol,timeframe); } void TimeFrameDown() { ENUM_TIMEFRAMES timeframe; switch(_Period) { case PERIOD_M2: timeframe=PERIOD_M1; break; case PERIOD_M3: timeframe=PERIOD_M2; break; case PERIOD_M5: timeframe=PERIOD_M3; break; case PERIOD_M10: timeframe=PERIOD_M5; break; case PERIOD_M15: timeframe=PERIOD_M10; break; case PERIOD_M30: timeframe=PERIOD_M15; break; case PERIOD_H1: timeframe=PERIOD_M30; break; case PERIOD_H4: timeframe=PERIOD_H1; break; case PERIOD_D1: timeframe=PERIOD_H4; break; case PERIOD_W1: timeframe=PERIOD_D1; break; case PERIOD_MN1: timeframe=PERIOD_W1; break; default: timeframe=PERIOD_M3; } ChartSetSymbolPeriod(0,_Symbol,timeframe); } void TimeFrameInTheMiddle() { ChartSetSymbolPeriod(0,_Symbol,PERIOD_M3); }
keybd_event is used for pressing Alt+R (tile arranging chart windows), numpad 5 (stretches the chart so price reaches top and bottom) and End (shifts chart to the end after zoom change).
It's better to use include/virtualkeys.mqh than key codes.
This from a code I'm using:
keybd_event is used for pressing Alt+R (tile arranging chart windows), numpad 5 (stretches the chart so price reaches top and bottom) and End (shifts chart to the end after zoom change).
It's better to use include/virtualkeys.mqh than key codes.
Thank you all. I will try.
This from a code I'm using:
keybd_event is used for pressing Alt+R (tile arranging chart windows), numpad 5 (stretches the chart so price reaches top and bottom) and End (shifts chart to the end after zoom change).
It's better to use include/virtualkeys.mqh than key codes.
Thank you VEEEEEEERY Much.
God bless you.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use