Hello everyone, I'm really curious to do some backtests of the famous candle patterns. I'm not very expert in programming, I wanted to ask you if you think this code is right to identify the three white soldiers .. thanks in advance for your answers
void OnTick()
- Leverage for each symbol
- Questions from Beginners MQL5 MT5 MetaTrader 5
- I want a simple trailing stop & SL & TP CodeBase for mt5
Roberto Danilo:
Check out this library: https://www.mql5.com/en/code/291
Hello everyone, I'm really curious to do some backtests of the famous candle patterns. I'm not very expert in programming, I wanted to ask you if you think this code is right to identify the three white soldiers .. thanks in advance for your answers
void OnTick()
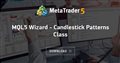
MQL5 Wizard - Candlestick Patterns Class
- www.mql5.com
The generic idea is the following: the class of trading signals is derived from CExpertSignal, the next, it's necessary to override the LongCondition() and ShortCondition() virtual methods with your own methods. The best way is to create the separate class, derived from CExpertSignal for checking of formation of candlestick patterns. For...
Roberto Danilo:
Hello everyone, I'm really curious to do some backtests of the famous candle patterns. I'm not very expert in programming, I wanted to ask you if you think this code is right to identify the three white soldiers .. thanks in advance for your answers
void OnTick()
Dear,
These are the conditions for Three White Soldiers:
Close[1]>Open[1] && Close[2]>Open[2] && Close[3]>Open[3] && High[1]>High[2] && High[2]>High[3] && Close[1]>Close[2] && Close[2]>Close[3]
fantastic library but I have never used mt5. It would be fantastic such a thing for mt4!
Arash Lakzadeh:
Dear,
These are the conditions for Three White Soldiers:
seems to work, thank you very much for your help. Now I have to refine the backtest, the average winnings is 48% but you could get up with some sort of filter.
Roberto Danilo:
fantastic library but I have never used mt5. It would be fantastic such a thing for mt4!
seems to work, thank you very much for your help. Now I have to refine the backtest, the average winnings is 48% but you could get up with some sort of filter.
Great.
Good luck
I think something is wrong. I tried with visual mode, and it seems to me that you open orders without respecting the function of the candle!
the first order does not seem to respect the parameters. This is the code :
//+------------------------------------------------------------------+ //| pricepattern1.mq4 | //| Copyright 2018, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2018, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #property strict double open1, open2, open3, close1, close2, close3, low1, low2, low3, high1, high2, high3, ma; extern int stop_loss=100; extern int take_profit=100; double TP= NormalizeDouble(take_profit*10*Point,5); double SL= NormalizeDouble(stop_loss*10*Point,5); double lotto = 0.01; extern bool Compounding=true; extern double Rischio=2.0; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { ma = iMA(Symbol(),PERIOD_CURRENT,50,0,MODE_SMA,PRICE_CLOSE,1); open1 = NormalizeDouble(iOpen(Symbol(), Period(), 1), 5); open2 = NormalizeDouble(iOpen(Symbol(), Period(), 2), 5); open3 = NormalizeDouble(iOpen(Symbol(), Period(), 3), 5); close1 = NormalizeDouble(iClose(Symbol(), Period(), 1), 5); close2 = NormalizeDouble(iClose(Symbol(), Period(), 2), 5); close3 = NormalizeDouble(iClose(Symbol(), Period(), 3), 5); low1 = NormalizeDouble(iLow(Symbol(), Period(), 1), 5); low2 = NormalizeDouble(iLow(Symbol(), Period(), 2), 5); low3 = NormalizeDouble(iLow(Symbol(), Period(), 3), 5); high1 = NormalizeDouble(iHigh(Symbol(), Period(), 1), 5); high2 = NormalizeDouble(iHigh(Symbol(), Period(), 2), 5); high3 = NormalizeDouble(iHigh(Symbol(), Period(), 3), 5); if(NewBar()){ if (CheckCandle()<ma) EseguiOrdineLong(); } } //+------------------------------------------------------------------+ bool CheckCandle() { if( close1>open1 && close2>open2 && close3>open3 && high1>high2 && high2>high3 && close1>close2 && close2>close3) return true; else return false; } void EseguiOrdineLong() { if (OrdersTotal() == 0) { OrderSend(Symbol(),OP_BUYSTOP,lotto,high1,0,Ask-SL,Ask+TP,"Ordine Long",123,TimeCurrent()+3600); } } bool NewBar(){ static datetime lastbar; datetime curbar = Time[0]; if(lastbar!=curbar){ lastbar=curbar; return (true); } else{ return(false); } }
if (CheckCandle()<ma) EseguiOrdineLong(); bool CheckCandle() {
Since CheckCandle returns a bool (zero or one,) won't the condition always be true (rates above 1.00000)OrderSend(Symbol(),OP_BUYSTOP,lotto,high1,0,Ask-SL,Ask+TP,"Ordine Long",123,TimeCurrent()+3600);
- Since you are buying at the high1, shouldn't your stops be relative to that?
-
Check your return codes for errors, and report them including GLE/LE.
Don't look at it unless you have an error.
Don't just silence the compiler, it is trying to help you.
What are Function return values ? How do I use them ? - MQL4 and MetaTrader 4 - MQL4 programming forum
Common Errors in MQL4 Programs and How to Avoid Them - MQL4 Articles
Only those functions that return a value (e.g. iClose, MarketInfo, etc.) must you call ResetLastError before in order to check after.
if (OrdersTotal() == 0) {
Using OrdersTotal directly and/or no Magic number filtering on your OrderSelect loop means your code is incompatible with every EA (including itself on other charts and manual trading.)
Symbol Doesn't equal Ordersymbol when another currency is added to another seperate chart . - MQL4 and MetaTrader 4 - MQL4 programming forum
MagicNumber: "Magic" Identifier of the Order - MQL4 Articles
whroeder1:
- Since CheckCandle returns a bool (zero or one,) won't the condition always be true (rates above 1.00000)
because, however, opens orders even when the condition is false? thanks for your reply
whroeder1:
- Since CheckCandle returns a bool (zero or one,) won't the condition always be true (rates above 1.00000)
- Since you are buying at the high1, shouldn't your stops be relative to that?
-
Check your return codes for errors, and report them including GLE/LE.
Don't look at it unless you have an error.
Don't just silence the compiler, it is trying to help you.
What are Function return values ? How do I use them ? - MQL4 and MetaTrader 4 - MQL4 programming forum
Common Errors in MQL4 Programs and How to Avoid Them - MQL4 Articles
Only those functions that return a value (e.g. iClose, MarketInfo, etc.) must you call ResetLastError before in order to check after.
-
Using OrdersTotal directly and/or no Magic number filtering on your
OrderSelect loop means your code is incompatible with every EA (including itself on other charts and manual
trading.)
Symbol Doesn't equal Ordersymbol when another currency is added to another seperate chart . - MQL4 and MetaTrader 4 - MQL4 programming forum
MagicNumber: "Magic" Identifier of the Order - MQL4 Articles
Thank you for your answer. I added the ( ) to true and false in the bool function, and now everything works fine even in the visual.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register