So what you're doing is creating an array of static objects and then trying to copy the values from from one object to another. You actually need to be working with pointers and pointer arrays. Furthermore you should also ensure that you have implemented proper memory management. Thankfully, MQL has some pointer collections built into the standard library that automatically handle memory and size. Just make sure you're always inheriting from CObject and that you're using the pointers to dynamic objects. Here is an example.
#include<Arrays\List.mqh> class Line : public CObject { public: string name; double value; }; CList line_list; void void OnStart() { for(int i=10; i > 0; i--) { Line *line = new Line line.name = "line_"+string(i); line.value = i; line_list.Add(line); } Line *line = line_list.GetFirstNode(); for(; CheckPointer(line); line=line.Next()) Print(line.name); }
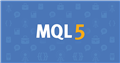
Documentation on MQL5: Standard Library / Data Collections
- www.mql5.com
This section contains the technical details on working with various data structures (arrays, linked lists, etc.) and description of the relevant components of the MQL5 Standard Library.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hi
The below code gives the error "structure have objects and cannot be copied"
any suggestions on how to get it working?