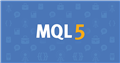
- www.mql5.com
koranged:
for(day=1; day<=30; day++) One_Farm=One_Farm*(1+Perc_day/100);
'Expression_1' of the 'for' loop again. This process continues until condition 'day<=30' is false, at which point control can be passed outside of the 'for' loop to the next line of the code - 'Mons++'
or
'Mons++' which will be executed as soon as the first iteration of 'day++' is completed - regardless of whether or not 'day<=30' is false.
Mons++ is not part of the for loop. It executes after the for loop exits.
Thankyou.
It is good practice to use the value with decimal point when you are manipulating double type values.
The operation (1 + Perc_day / 100) could generate an integer value that would always be equal to 1.
The correct method would be (1.0 + Perc_day / 100.0)
It is good practice to use the value with decimal point when you are manipulating double type values.
The operation (1 + Perc_day / 100) could generate an integer value that would always be equal to 1.
The correct method would be (1.0 + Perc_day / 100.0)
Okay cheers.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I am going step by step through the manual and have become a bit confused at this page - https://book.mql4.com/operators/continue. My question should be pretty simple for anyone who understands these loops and where control should/shouldn't be passed to next. Here's the full code:
The following section is leading to my confusion:
Following execution of the 'for' operator body, variable 'One_Farm' will be assigned the value of 1010, and control will then be passed to 'Expression_2' - 'day++', which will increment the value of 'day' by 1 and then pass control to either:
'Expression_1' of the 'for' loop again. This process continues until condition 'day<=30' is false, at which point control can be passed outside of the 'for' loop to the next line of the code - 'Mons++'
or
'Mons++' which will be executed as soon as the first iteration of 'day++' is completed - regardless of whether or not 'day<=30' is false.
-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Which of the above is correct?
Thanks for assistance.