Hello, great indy. Could you have this display up to 8 instruments at a time? tnx
Yes it seems that it would be easy to extend the code to as many pairs as you wanted. I am looking at making the update now. If I do I will post it here.
Here is the code for the indicator with 8 pairs
//+------------------------------------------------------------------+ //| Currency Strength Meter.mq4 | //| Copyright 2018, Besarion Turmanauli | //| https://www.mql5.com/en/users/dos.ge | //+------------------------------------------------------------------+ #property copyright "Copyright 2018, Besarion Turmanauli" #property link "https://www.mql5.com/en/users/dos.ge" #property version "1.00" #property strict #property indicator_separate_window #property indicator_minimum 0 #property indicator_maximum 100 #property indicator_buffers 8 #property indicator_plots 8 //--- plot Label1 //#property indicator_label1 "Label1" #property indicator_type1 DRAW_LINE #property indicator_color1 clrRed #property indicator_style1 STYLE_SOLID #property indicator_width1 1 //--- plot Label2 //#property indicator_label2 "Label2" #property indicator_type2 DRAW_LINE #property indicator_color2 clrViolet #property indicator_style2 STYLE_SOLID #property indicator_width2 1 //--- plot Label3 //#property indicator_label3 "Label3" #property indicator_type3 DRAW_LINE #property indicator_color3 clrGreen #property indicator_style3 STYLE_SOLID #property indicator_width3 1 //--- plot Label4 //#property indicator_label4 "Label4" #property indicator_type4 DRAW_LINE #property indicator_color4 clrBlue #property indicator_style4 STYLE_SOLID #property indicator_width4 1 //--- plot Label5 //#property indicator_label5 "Label5" #property indicator_type5 DRAW_LINE #property indicator_color5 clrLightGreen #property indicator_style5 STYLE_SOLID #property indicator_width5 1 //--- plot Label6 //#property indicator_label6 "Label6" #property indicator_type6 DRAW_LINE #property indicator_color6 clrLightGray #property indicator_style6 STYLE_SOLID #property indicator_width6 1 //--- plot Label7 //#property indicator_label7 "Label7" #property indicator_type7 DRAW_LINE #property indicator_color7 clrKhaki #property indicator_style7 STYLE_SOLID #property indicator_width7 1 //--- plot Label8 //#property indicator_label8 "Label8" #property indicator_type8 DRAW_LINE #property indicator_color8 clrSandyBrown #property indicator_style8 STYLE_SOLID #property indicator_width8 1 //--- indicator buffers double Label1Buffer[]; double Label2Buffer[]; double Label3Buffer[]; double Label4Buffer[]; double Label5Buffer[]; double Label6Buffer[]; double Label7Buffer[]; double Label8Buffer[]; #define ISN "Currency Strength Meter +8" int initial_limit=0; extern string instrOne = "EURUSD"; //Instrument 1 extern string instrTwo = "AUDUSD"; //Instrument 2 extern string instrThree= "NZDUSD"; //Instrument 3 extern string instrFour = "USDJPY"; //Instrument 4 extern string instrFive = "GBPJPY"; //Instrument 5 extern string instrSix = "GBPUSD"; //Instrument 6 extern string instrSeven= "EURNZD"; //Instrument 7 extern string instrEight = "GBPNZD"; //Instrument 8 extern color colorOne=clrRed; //Instrument 1 Color extern color colorTwo=clrViolet; //Instrument 2 Color extern color colorThree=clrGreen; //Instrument 3 Color extern color colorFour= clrBlue; //Instrument 4 Color extern color colorFive=clrLightGreen; //Instrument 5 Color extern color colorSix=clrLightGray; //Instrument 6 Color extern color colorSeven=clrKhaki; //Instrument 7 Color extern color colorEight= clrSandyBrown; //Instrument 8 Color extern int indicatorPeriod = 14; //Indicator Period extern ENUM_APPLIED_PRICE appliedPrice=PRICE_CLOSE; //Indicator Applied Price //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { if(!LabelCreate(0,instrOne,1,5,20,CORNER_LEFT_LOWER,instrOne,"Arial",10,colorOne)) { return (0); } if(!LabelCreate(0,instrTwo,1,75,20,CORNER_LEFT_LOWER,instrTwo,"Arial",10,colorTwo)) { return (0); } if(!LabelCreate(0,instrThree,1,145,20,CORNER_LEFT_LOWER,instrThree,"Arial",10,colorThree)) { return (0); } if(!LabelCreate(0,instrFour,1,215,20,CORNER_LEFT_LOWER,instrFour,"Arial",10,colorFour)) { return (0); } if(!LabelCreate(0,instrFive,1,285,20,CORNER_LEFT_LOWER,instrFive,"Arial",10,colorFive)) { return (0); } if(!LabelCreate(0,instrSix,1,355,20,CORNER_LEFT_LOWER,instrSix,"Arial",10,colorSix)) { return (0); } if(!LabelCreate(0,instrSeven,1,425,20,CORNER_LEFT_LOWER,instrSeven,"Arial",10,colorSeven)) { return (0); } if(!LabelCreate(0,instrEight,1,495,20,CORNER_LEFT_LOWER,instrEight,"Arial",10,colorEight)) { return (0); } SetLevelValue(0,80.0); SetLevelValue(1,50.0); SetLevelValue(2,20.0); //--- indicator buffers mapping SetIndexBuffer(0,Label1Buffer); SetIndexLabel(0,instrOne); SetIndexStyle(0,0,0,1,colorOne); SetIndexBuffer(1,Label2Buffer); SetIndexLabel(1,instrTwo); SetIndexStyle(1,0,0,1,colorTwo); SetIndexBuffer(2,Label3Buffer); SetIndexLabel(2,instrThree); SetIndexStyle(2,0,0,1,colorThree); SetIndexBuffer(3,Label4Buffer); SetIndexLabel(3,instrFour); SetIndexStyle(3,0,0,1,colorFour); SetIndexBuffer(4,Label5Buffer); SetIndexLabel(4,instrFive); SetIndexStyle(4,0,0,1,colorFive); SetIndexBuffer(5,Label6Buffer); SetIndexLabel(5,instrSix); SetIndexStyle(5,0,0,1,colorSix); SetIndexBuffer(6,Label7Buffer); SetIndexLabel(6,instrSeven); SetIndexStyle(6,0,0,1,colorSeven); SetIndexBuffer(7,Label8Buffer); SetIndexLabel(7,instrEight); SetIndexStyle(7,0,0,1,colorEight); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int start() { int i,// Bar index Counted_bars; // Number of counted bars Counted_bars=IndicatorCounted(); // Number of counted bars i=Bars-Counted_bars-1; // Index of the first uncounted if(initial_limit==0){initial_limit=i;} while(i>0) {//start iteration //getting initial values double open=Open[i]; double high=High[i]; double low=Low[i]; double close=Close[i]; Label1Buffer[i]=iRSI(instrOne,Period(),indicatorPeriod,PRICE_CLOSE,i); Label2Buffer[i]=iRSI(instrTwo,Period(),indicatorPeriod,PRICE_CLOSE,i); Label3Buffer[i]=iRSI(instrThree,Period(),indicatorPeriod,PRICE_CLOSE,i); Label4Buffer[i]=iRSI(instrFour,Period(),indicatorPeriod,PRICE_CLOSE,i); Label5Buffer[i]=iRSI(instrFive,Period(),indicatorPeriod,PRICE_CLOSE,i); Label6Buffer[i]=iRSI(instrSix,Period(),indicatorPeriod,PRICE_CLOSE,i); Label7Buffer[i]=iRSI(instrSeven,Period(),indicatorPeriod,PRICE_CLOSE,i); Label8Buffer[i]=iRSI(instrEight,Period(),indicatorPeriod,PRICE_CLOSE,i); i--; }//end iteration return (0); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ int deinit() { LabelDelete(0,instrOne); LabelDelete(0,instrTwo); LabelDelete(0,instrThree); LabelDelete(0,instrFour); LabelDelete(0,instrFive); LabelDelete(0,instrSix); LabelDelete(0,instrSeven); LabelDelete(0,instrEight); return(0); } //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ //| Create a text label | //+------------------------------------------------------------------+ bool LabelCreate(const long chart_ID=0, // chart's ID const string name="Label", // label name const int sub_window=0, // subwindow index const int x=0, // X coordinate const int y=0, // Y coordinate const ENUM_BASE_CORNER corner=CORNER_LEFT_UPPER, // chart corner for anchoring const string text="Label", // text const string font="Arial", // font const int font_size=10, // font size const color clr=clrRed, // color const double angle=0.0, // text slope const ENUM_ANCHOR_POINT anchor=ANCHOR_LEFT_UPPER, // anchor type const bool back=false, // in the background const bool selection=false, // highlight to move const bool hidden=true, // hidden in the object list const long z_order=0) // priority for mouse click { //--- reset the error value ResetLastError(); //--- create a text label if(!ObjectCreate(chart_ID,name,OBJ_LABEL,sub_window,0,0)) { Print(__FUNCTION__, ": failed to create text label! Error code = ",GetLastError()); return(false); } //--- set label coordinates ObjectSetInteger(chart_ID,name,OBJPROP_XDISTANCE,x); ObjectSetInteger(chart_ID,name,OBJPROP_YDISTANCE,y); //--- set the chart's corner, relative to which point coordinates are defined ObjectSetInteger(chart_ID,name,OBJPROP_CORNER,corner); //--- set the text ObjectSetString(chart_ID,name,OBJPROP_TEXT,text); //--- set text font ObjectSetString(chart_ID,name,OBJPROP_FONT,font); //--- set font size ObjectSetInteger(chart_ID,name,OBJPROP_FONTSIZE,font_size); //--- set the slope angle of the text ObjectSetDouble(chart_ID,name,OBJPROP_ANGLE,angle); //--- set anchor type ObjectSetInteger(chart_ID,name,OBJPROP_ANCHOR,anchor); //--- set color ObjectSetInteger(chart_ID,name,OBJPROP_COLOR,clr); //--- display in the foreground (false) or background (true) ObjectSetInteger(chart_ID,name,OBJPROP_BACK,back); //--- enable (true) or disable (false) the mode of moving the label by mouse ObjectSetInteger(chart_ID,name,OBJPROP_SELECTABLE,selection); ObjectSetInteger(chart_ID,name,OBJPROP_SELECTED,selection); //--- hide (true) or display (false) graphical object name in the object list ObjectSetInteger(chart_ID,name,OBJPROP_HIDDEN,hidden); //--- set the priority for receiving the event of a mouse click in the chart ObjectSetInteger(chart_ID,name,OBJPROP_ZORDER,z_order); //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Delete a text label | //+------------------------------------------------------------------+ bool LabelDelete(const long chart_ID=0, // chart's ID const string name="Label") // label name { //--- reset the error value ResetLastError(); //--- delete the label if(!ObjectDelete(chart_ID,name)) { Print(__FUNCTION__, ": failed to delete a text label! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+
thanks. it is possible due the cluster issue, that works with all 28 pairs?
hi how to download currency strenght meter sir..
Huzaifah Ajwad: hi how to download currency strenght meter sir..
Link is in the first post
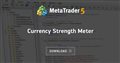
Currency Strength Meter
- www.mql5.com
Currency Strength Meter indicator gets RSI (Relative Strength Index) values from four different instruments of your choice and displays them in indicator window. Features Ability to set custom colors and styles for each instrument. Displays colored labels for instrument identification. You can adjust period and applied price. Supports all...
Hi tried to download the currency trength meter no success please help
Hi
I it possible to get this detail from Excel RTD code??
please give code detail for this.
thnaks
this is just the RSI Indicator showing other currencies as well
jusiur #:
thanks. it is possible due the cluster issue, that works with all 28 pairs?
thanks. it is possible due the cluster issue, that works with all 28 pairs?
Probably possibile using MT5 but unuseful IMHO. Crosses are mathematical relationships among majors so they do not add any more informations to those already embedded into majors. Then if you choose the majors as pairs of the indicator it Is enough.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Currency Strength Meter:
Currency Strength Meter indicator gets RSI (Relative Strength Index) values from four different instruments of your choice and displays them in indicator window.
Features
Author: Besarion Turmanauli