I made this ea to close all open trades when total pips reach +30, but it doesn't seem to be working, what's wrong with the code?
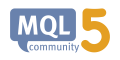
Automated Trading and Strategy Testing
- www.mql5.com
MQL5: language of trade strategies built-in the MetaTrader 5 Trading Platform, allows writing your own trading robots, technical indicators, scripts and libraries of functions
- Summing up open buy and sell orders profits
- [ARCHIVE] Any rookie question, so as not to clutter up the forum. Professionals, don't pass by. Nowhere without you - 3.
- Any questions from newcomers on MQL4 and MQL5, help and discussion on algorithms and codes
- Use the new Event Handling Functions - Functions - Language Basics - MQL4 Reference or use the old style. Do not use both.
double pipsare= (OrderOpenPrice()-Bid)/(Point*10);
Why Bid? What if it a sell order? Use OrderClosePrice().Code breaks on 4 digit brokers. Compute what a pip is. You are not adjusting SL, TP, and slippage; for 4/5 digit brokers and for JPY pairs. How to manage JPY pairs with parameters? - MQL4 and MetaTrader 4 - MQL4 programming forum
- case OP_SELL : result = OrderClose( OrderTicket(), OrderLots(), MarketInfo(OrderSymbol(), MODE_ASK), 5, White );Use OrderClosePrice() and you don't have to check it's type.
for(int i=total-1;i>=0;i--){ OrderSelect(i, SELECT_BY_POS);
In the presence of multiple orders (one EA multiple charts, multiple EAs, manual trading,) because while you are waiting for the current operation (closing, deleting, modifying) to complete, any number of other operations on other orders could have concurrently happened and changed the position indexing:For non-FIFO (US brokers,) (or the EA only opens one order per symbol,) you can simply count down in a position loop, and you won't miss orders. Get in the habit of always counting down. Loops and Closing or Deleting Orders - MQL4 forum - For FIFO (US brokers,) and you (potentially) process multiple orders, you must count up and on a successful operation, reprocess all positions (set index to -1 before continuing.)
-
and check OrderSelect. What are Function return values ? How do I use them ? - MQL4 forum Common Errors in MQL4 Programs and How to Avoid Them - MQL4 Articles - and if you (potentially) process multiple orders, must call RefreshRates() after server calls if you want to use the Predefined Variables (Bid/Ask) or OrderClosePrice() instead, on the next order/server call.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register