Time is a datetime datatype you can not convert it to integer datatype like that by typecasting.
In stead use
TimeHour()
TimeMinute()
Etc please see:
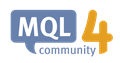
Jose Francisco Casado Fernandez: define the 'prevtime' variable as the datetime type,
| Exactly, no casting required. And simplify your code:
if(!IsTradeAllowed() || Time[0] == prevtime) return(0); prevtime = Time[0]; |
As we told you in the other post you have to declare the 'prevtime' variable as the datetime type, and you continue to declare it as int type.
extern bool PolLots = true; extern int MaxOrders = 1; // Other global variables // datetime prevtime; // Expert initialisation function int init() { return(0); } //-----------------------------------------------------
There is no need to double topics.
Many already pointed out to you that you are combining:
int prevtime;
with:
datetime Time[]
And pointed out that these datatypes are not compatible with each other which is why:
(int)Time[]
Will not work.
You solution for working with time can only be:
datetime prevtime;
As pointed out by Jose and i believe others too.
The warning is simply the compiler telling you, hey you are trying to mix whole integers with datetime datatype, which is kinda weird so there could be dataloss, it's a warning not an error.
As we told you in the other post you have to declare the 'prevtime' variable as the datetime type, and you continue to declare it as int type.
Thanks for your help Jose.
There is no need to double topics.
Many already pointed out to you that you are combining:
with:
And pointed out that these datatypes are not compatible with each other which is why:
Will not work.
You solution for working with time can only be:
As pointed out by Jose and i believe others too.
The warning is simply the compiler telling you, hey you are trying to mix whole integers with datetime datatype, which is kinda weird so there could be dataloss, it's a warning not an error.
Thanks for your help Marco.
Exactly, no casting required. And simplify your code: |
Thank you for your help.
Moved from duplicated topic - moderator
greenpar 2017.05.18 11:44
In my EA I am getting the error message, "possible loss of data due to type conversion", for both occurrences of "prevtime = Time" at the end of the EA code below:
#property strict // EA Inputs extern int TrailingStop = 20; extern int StopLoss = 0; extern double Lots = 0.1; extern int magicnumber = 777; extern bool PolLots = true; extern int MaxOrders = 1; // Other global variables // int prevtime; // Expert initialisation function int init() { return(0); } // Expert deinitialisation function int deinit() { return(0); } // Expert start function int start() { int i=0; int total = OrdersTotal(); for(i = 0; i <= total; i++) { if(TrailingStop>0) { OrderSelect(i, SELECT_BY_POS, MODE_TRADES); if(OrderMagicNumber() == magicnumber) { TrailingStairs(OrderTicket(),TrailingStop); } } } bool BuyOp=false; bool SellOp=false; if (High[0]>High[1]&&High[1]>High[2]&&High[2]>High[3]&&Open[0]>Open[1]&&Open[1]>Open[2]&&Open[2]>Open[3]) BuyOp=true; if (High[0]<High[1]&&High[1]<High[2]&&High[2]<High[3]&&Open[0]<Open[1]&&Open[1]<Open[2]&&Open[2]<Open[3]) SellOp=true; if(Time[0] == prevtime) return(0); prevtime = (int) Time[0]; if(!IsTradeAllowed()) { prevtime = (int) Time[1]; return(0); }
I had originally posted this in the wrong section of the forum and it was suggested that:
1) Time is a datetime datatype you can not convert it to integer datatype like that by typecasting.
Instead use:
TimeHour () TimeMinute ()
From - https://docs.mql4.com/dateandtime
2) You should define the 'prevtime' variable as the datetime type, and so you would not have to do typecasting with possible data loss. Regards.
3) Exactly, no casting required. And simplify your code:
if(!IsTradeAllowed() || Time[0] == prevtime) return(0); prevtime = Time[0];
Using the code from point 3 above (see code below) I still get the same error message, probably because I have used the datetime type. Would anyone be able to help me further?
Thank you.
#property strict // EA Inputs extern int TrailingStop = 20; extern int StopLoss = 0; extern double Lots = 0.1; extern int magicnumber = 777; extern bool PolLots = true; extern int MaxOrders = 1; // Other global variables // int prevtime; // Expert initialisation function int init() { return(0); } // Expert deinitialisation function int deinit() { return(0); } // Expert start function int start() { int i=0; int total = OrdersTotal(); for(i = 0; i <= total; i++) { if(TrailingStop>0) { OrderSelect(i, SELECT_BY_POS, MODE_TRADES); if(OrderMagicNumber() == magicnumber) { TrailingStairs(OrderTicket(),TrailingStop); } } } bool BuyOp=false; bool SellOp=false; if (High[0]>High[1]&&High[1]>High[2]&&High[2]>High[3]&&Open[0]>Open[1]&&Open[1]>Open[2]&&Open[2]>Open[3]) BuyOp=true; if (High[0]<High[1]&&High[1]<High[2]&&High[2]<High[3]&&Open[0]<Open[1]&&Open[1]<Open[2]&&Open[2]<Open[3]) SellOp=true; /* if(Time[0] == prevtime) return(0); prevtime = (int) Time[0]; if(!IsTradeAllowed()) { prevtime = (int) Time[1]; return(0); } */ if(!IsTradeAllowed() || Time[0] == prevtime) return(0); prevtime = Time[0];

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
In my EA I am getting the error message, "possible loss of data due to type conversion", for both occurrences of "prevtime = Time" in the EA code below:
As outlined in this article, Common Errors in MQL4 Programs and How to Avoid Them, these errors messages can be prevented by inserting "(int)" before "Time", as shown below:
Is this the correct approach, an incorrect use of "Time", or something else?
Thank you.