{//setorders
if(firstaction=="BUY")
int ticket1=OrderSend(FirstSymbol,OP_BUY,lotsize,Ask,slippage,0,0,NULL,magicnumber,0,clrBlue);
else if(firstaction=="SELL")
int ticket1=OrderSend(FirstSymbol,OP_SELL,lotsize,Bid,slippage,0,0,NULL,magicnumber,0,clrRed);
if(secondaction=="BUY")
int ticket2=OrderSend(SecondSymbol,OP_BUY,lotsize,Ask,slippage,0,0,NULL,magicnumber,0,clrBlue);
else if(secondaction=="SELL")
int ticket2=OrderSend(SecondSymbol,OP_SELL,lotsize,Bid,slippage,0,0,NULL,magicnumber,0,clrRed);
}//setorders
You should check your return codes on the OrderSend.
I'm guessing this EA is running on GBPAUD chart? If so, here is what I think is happening:
Your code is running twice, hence 2 orders opening on GBPAUD.
The OrderSend on EURUSD is failing because you are trying to open it at the Ask price on GBPAUD, which is a long way away from the Ask price on EURUSD!
You will find information on both checking the returned value and about opening trades on other symbols in the OrderSend documentation.
HTH
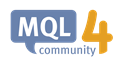
- docs.mql4.com
{//setorders
if(firstaction=="BUY")
int ticket1=OrderSend(FirstSymbol,OP_BUY,lotsize,Ask,slippage,0,0,NULL,magicnumber,0,clrBlue);
else if(firstaction=="SELL")
int ticket1=OrderSend(FirstSymbol,OP_SELL,lotsize,Bid,slippage,0,0,NULL,magicnumber,0,clrRed);
if(secondaction=="BUY")
int ticket2=OrderSend(SecondSymbol,OP_BUY,lotsize,Ask,slippage,0,0,NULL,magicnumber,0,clrBlue);
else if(secondaction=="SELL")
int ticket2=OrderSend(SecondSymbol,OP_SELL,lotsize,Bid,slippage,0,0,NULL,magicnumber,0,clrRed);
}//setorders
You should check your return codes on the OrderSend.
I'm guessing this EA is running on GBPAUD chart? If so, here is what I think is happening:
Your code is running twice, hence 2 orders opening on GBPAUD.
The OrderSend on EURUSD is failing because you are trying to open it at the Ask price on GBPAUD, which is a long way away from the Ask price on EURUSD!
You will find information on both checking the returned value and about opening trades on other symbols in the OrderSend documentation.
HTH
Thank you Knave
No. The ea is running once... It doesn't matter I am running theis on any chart. It will open its orders for the given symbols.
Because I did not use Symbol() in the ordersend().
Not using Symbol is only half the solution. You also need to use the correct price too.
Maybe this will help explain what I mean better. Please check your Expert tab for messages after you run the code.
{
Print("setorders() running");
if(firstaction=="BUY")
{
int ticket1=OrderSend(FirstSymbol,OP_BUY,lotsize,Ask,slippage,0,0,NULL,magicnumber,0,clrBlue);
if(ticket1<0) Print("OrderSend on FirstSymbol Buy failed with error #",GetLastError());
}
else if(firstaction=="SELL")
{
int ticket1=OrderSend(FirstSymbol,OP_SELL,lotsize,Bid,slippage,0,0,NULL,magicnumber,0,clrRed);
if(ticket1<0) Print("OrderSend on FirstSymbol Sell failed with error #",GetLastError());
}
if(secondaction=="BUY")
{
int ticket2=OrderSend(SecondSymbol,OP_BUY,lotsize,Ask,slippage,0,0,NULL,magicnumber,0,clrBlue);
if(ticket2<0) Print("OrderSend on SecondSymbol Buy failed with error #",GetLastError());
}
else if(secondaction=="SELL")
{
int ticket2=OrderSend(SecondSymbol,OP_SELL,lotsize,Bid,slippage,0,0,NULL,magicnumber,0,clrRed);
if(ticket2<0) Print("OrderSend on SecondSymbol Sell failed with error #",GetLastError());
}
}//setorders
Not using Symbol is only half the solution. You also need to use the correct price too.
Maybe this will help explain what I mean better. Please check your Expert tab for messages after you run the code.
{
Print("setorders() running");
if(firstaction=="BUY")
{
int ticket1=OrderSend(FirstSymbol,OP_BUY,lotsize,Ask,slippage,0,0,NULL,magicnumber,0,clrBlue);
if(ticket1<0) Print("OrderSend on FirstSymbol Buy failed with error #",GetLastError());
}
else if(firstaction=="SELL")
{
int ticket1=OrderSend(FirstSymbol,OP_SELL,lotsize,Bid,slippage,0,0,NULL,magicnumber,0,clrRed);
if(ticket1<0) Print("OrderSend on FirstSymbol Sell failed with error #",GetLastError());
}
if(secondaction=="BUY")
{
int ticket2=OrderSend(SecondSymbol,OP_BUY,lotsize,Ask,slippage,0,0,NULL,magicnumber,0,clrBlue);
if(ticket2<0) Print("OrderSend on SecondSymbol Buy failed with error #",GetLastError());
}
else if(secondaction=="SELL")
{
int ticket2=OrderSend(SecondSymbol,OP_SELL,lotsize,Bid,slippage,0,0,NULL,magicnumber,0,clrRed);
if(ticket2<0) Print("OrderSend on SecondSymbol Sell failed with error #",GetLastError());
}
}//setorders
when I use GBPAUD/BUY and GBPJPY/SELL as input. I have two GBPAUD/BUY orders.
But when I use GBPAUD/BUY and EURUSD/SELL . Two orders open correctly.
You really won't be able to get anywhere fixing this unless you check your OrderSend return values.
Also, I hate to labour the point but trying to open orders on other symbols using the predefined variables Bid and Ask is simply wrong.
From the documentation I linked you to:
- At opening of a market order (OP_SELL or OP_BUY), only the latest prices of Bid (for selling) or Ask (for buying) can be used as open price. If operation is performed with a security differing from the current one, the MarketInfo() function must be used with MODE_BID or MODE_ASK parameter for the latest quotes for this security to be obtained.
Your code should be looking more like this:
{
double price = SymbolInfoDouble(FirstSymbol,SYMBOL_ASK);
int ticket1=OrderSend(FirstSymbol,OP_BUY,lotsize,price,slippage,0,0,NULL,magicnumber,0,clrBlue);
if(ticket1<0) Print("OrderSend on FirstSymbol Buy failed with error #",GetLastError());
}
This works, Thank you.
void setorders()
This works, Thank you.
- At opening of a market order (OP_SELL or OP_BUY), only the latest prices of Bid (for selling) or Ask (for buying) can be used as open price. If operation is performed with a security differing from the current one, the MarketInfo() function must be used with MODE_BID or MODE_ASK parameter for the latest quotes for this security to be obtained.
- You can't use any predefined variables, can't use the tester, must poll (not OnTick,) and usually other problems.
- Code it to trade the chart pair only. Look at the others if you must.
- Then put it on other charts to trade the other pairs. Done.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi
I wrote an expert to open two position at the same time. and close them when the total profit/loss of the two order reach the profit/koss target.
User can enter the symbol names and type of two orders as input.
I did this:
input string firstaction="BUY";
input string SecondSymbol="EURUSD";
input string secondaction="SELL";
==================
I have a function that open orders:
{//setorders
if(firstaction=="BUY")
int ticket1=OrderSend(FirstSymbol,OP_BUY,lotsize,Ask,slippage,0,0,NULL,magicnumber,0,clrBlue);
else if(firstaction=="SELL")
int ticket1=OrderSend(FirstSymbol,OP_SELL,lotsize,Bid,slippage,0,0,NULL,magicnumber,0,clrRed);
if(secondaction=="BUY")
int ticket2=OrderSend(SecondSymbol,OP_BUY,lotsize,Ask,slippage,0,0,NULL,magicnumber,0,clrBlue);
else if(secondaction=="SELL")
int ticket2=OrderSend(SecondSymbol,OP_SELL,lotsize,Bid,slippage,0,0,NULL,magicnumber,0,clrRed);
}//setorders
=========================
The expert opens two orders but both of them are GBPAUD/BUY....