1- The order is first opened and then only after that the
2- the stop-loss and take profit are placed.
Most of the time this is not a problem but occassionally my stop loss order and take profit order are not placed so my position is left exposed with no stop loss.
Can anybody suggest a solution for this.
Yes, when the broker only offers "Market Execution", then you have to implement a two step order placement.
However, your placement of the stop-loss and the take-profit in the second step has to abide by the STOP-LEVEL rules. If you are placing the S/L or T/P too close to the STOP-LEVEL limits, and slippage occurs, then it is best to calculate your S/L and T/P based on the current market prices instead of the original opening price.
You should also have checks in place to detect when the S/L and T/P fail to update and to retry the operation with adjustments for current conditions. Alternatively, you can initially set wider stops and then after that, tighten them again so as not to leave your position initially unprotected.
Yes, when the broker only offers "Market Execution", then you have to implement a two step order placement.
However, your placement of the stop-loss and the take-profit in the second step has to abide by the STOP-LEVEL rules. If you are placing the S/L or T/P too close to the STOP-LEVEL limits, and slippage occurs, then it is best to calculate your S/L and T/P based on the current market prices instead of the original opening price.
You should also have checks in place to detect when the S/L and T/P fail to update and to retry the operation with adjustments for current conditions. Alternatively, you can initially set wider stops and then after that, tighten them again so as not to leave your position initially unprotected.
Hi Fernando,
Thanks for your help. I am a beginner when it comes to coding.
You said:
1. It is best to calculate your S/L and T/P based on the current market prices instead of the original opening price.
2. You should also have checks in place to detect when the S/L and T/P fail to update and to retry the operation with adjustments for current conditions.
I made a note to you on line 6 of the code below regarding your 2nd point.
Could you maybe show me in the code below how I might implement your suggestions. If it is not to complicated because I don't want to take too much of your time:)
Much appreciated.
Thanks.
void OrderSendSellStop(double priceStop,double stop)
{
int tik=-1;
int attempt = 0;
while (tik == -1 && attempt <=Attempt)////Fernando, I believe this line is to retry the original opening order. Do I need to do something similar for the Stop loss and TP order?
{
if (IsTradeAllowed() == true && IsTradeContextBusy() == false)
{
RefreshRates();
double price=NormalizeDouble(priceStop,Digits);
double stt=NormalizeDouble(stop,Digits);
if(stop==0){stt=0;}
double tpp=NormalizeDouble(price-Take_Profit*Point,Digits);
if(Take_Profit==0){tpp=0;}
int sleep=Slippage;
if(Slippage==0){sleep=3;}
double ltt=CalculateLots(Risk,X_Stop_Loss);
tik = OrderSend(Symbol(),OP_SELLSTOP,ltt,price,sleep,stt,tpp,"TrendLine",Magic_Number,0,clrRed);
LastTimeStopOrder=TimeCurrent();
if(tik==-1)
{
Print("error sell - ",GetLastError());
Sleep(1000);
}
attempt++;
}
}
}
//OrderSendSell--------------------------------------------------------
void OrderSendSell()
{
int tik=-1;
int attempt = 0;
while (tik == -1 && attempt <=Attempt)
{
if (IsTradeAllowed() == true && IsTradeContextBusy() == false)
{
RefreshRates();
double price=MarketInfo(Symbol(),MODE_BID);
int sleep=Slippage;
if(Slippage==0){sleep=3;}
double ltt=CalculateLots(Risk,X_Stop_Loss);
P=price;
tik = OrderSend(Symbol(),OP_SELL,ltt,price,sleep,0,0,"TrendLine",Magic_Number,0,clrRed);
LastTimeStopOrder=TimeCurrent();
if(tik==-1)
{
Print("error sell - ",GetLastError());
if(GetLastError()==134){Print("insufficient margin");}
Sleep(1000);
}
attempt++;
}
}
if(tik!=-1)
{
SendMail("TrendLine",(string)tik+" "+Symbol()+" SELL "+(string)P);
ModifySellTPSL();
}
}
Brian: Thanks for your help. I am a beginner when it comes to coding.
I made a note to you on line 6 of the code below regarding your 2nd point.Could you maybe show me in the code below how I might implement your suggestions. If it is not to complicated because I don't want to take too much of your time:)
@Brian: Part of your code is for Pending Orders and the other for Market Orders! So the requirements of a two step process is not required for Pending Orders. Only Market Orders require a two-step process for "Market Execution".
However, before setting a Stop-Loss or a Take Profit you should always take into account the values of "MarketInfo( _Symbol, MODE_STOPLEVEL )" and "MarketInfo( _Symbol, MODE_FREEZELEVEL )".
Please read the documentation Requirements and Limitations in Making Trades, especially about STOP-LEVEL and FREEZE-LEVEL, conditions which are set by your broker that affect the placement and minimum sizes for Stop-Loss and Take-Profit.
You can but you don't have to. SL/TP can be placed directly while opening the order. (and please don't argue without checking it firstly ).
@Alain: That is only possible with "Instant Execution" (or with Pending Orders). With "Market Execution" of Market Orders you CANNOT place the S/L and T/P at the same time as the Initial order placement. You HAVE to use a 2 step process.
And I am not just ARGUING for the sake of it, but because this I KNOW VERY WELL and it is common knowledge and many have discussed it here on the forum. Even in the Code Base, there are several implementations of the 2 setup process.
In the next two screen-shots, have a look at the execution "Type:" on your Order placement dialog box (non pending orders) and spot the differences in the S/L and T/P fields and the deviation/slippage as well.
PS! Screen-shots are old but still valid for this argument!
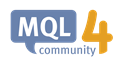
- book.mql4.com
@Alain: That is only possible with "Instant Execution" (or with Pending Orders). With "Market Execution" of Market Orders you CANNOT place the S/L and T/P at the same time as the Initial order placement. You HAVE to use a 2 step process.
And I am not just ARGUING for the sake of it, but because this I KNOW VERY WELL and it is common knowledge and many have discussed it here on the forum. Even in the Code Base, there are several implementations of the 2 setup process.
In the next two screen-shots, have a look at the execution "Type:" on your Order placement dialog box (non pending orders) and spot the differences in the S/L and T/P fields and the deviation/slippage as well.
PS! Screen-shots are old but still valid for this argument!
Hello,
I believe if I run an EA in an ecn account then market orders open in two stages:
1- The order is first opened and then only after that the
2- the stop-loss and take profit are placed.
Most of the time this is not a problem but occassionally my stop loss order and take profit order are not placed so my position is left exposed with no stop loss.
Can anybody suggest a solution for this.
Thanks.
Fernando, it's as you wish, I will not argue with you again, I already wasted enough time. You are just wrong, stay with your certainties.
Even when I show you proof (via the screen-shots)? Well, it seems you have never traded with a decent ECN broker then!
You really don't know what you are talking about.
- Where in the documentation do you see some restrictions about placing SL/TP on Market execution ? Via GUI or code.
- Here a screenshot from a real account, ECN broker :
- Here is the log from an order placed with SL/TP at once :
2016.11.08 19:18:17.430 'XXXXXX': order was opened : #11987283 sell 0.01 EURUSD at 1.10248 sl: 1.10258 tp: 1.10237
2016.11.08 19:18:16.996 'XXXXXX': order sell market 0.01 EURUSD sl: 1.10258 tp: 1.10237
- It's more than 2 years, it's was already well known on forum (by code). It was announced more than 3 years ago on build 500.Terminal: Removed check for the absence of SL and TP when opening positions for trading symbols of Market Execution type when trading from MQL4 applications.
- On the MT4 GUI it was available later, but I don't remember from which build.
- P.S: I found the announcement about MT4 GUI, it was on build 710.
Forum on trading, automated trading systems and testing trading strategies
stop loss order not being placed
Alain Verleyen, 2016.11.08 12:06
You can but you don't have to. SL/TP can be placed directly while opening the order. (and please don't argue without checking it firstly).
- Terminal: Allowed specification of Stop Loss and Take Profit when opening a position for a Market Execution type symbol.
Thank you Fernando and Alain for your suggestions.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello,
I believe if I run an EA in an ecn account then market orders open in two stages:
1- The order is first opened and then only after that the
2- the stop-loss and take profit are placed.
Most of the time this is not a problem but occassionally my stop loss order and take profit order are not placed so my position is left exposed with no stop loss.
Can anybody suggest a solution for this.
Thanks.