Hi,
why do you need to do like this:
HistorySelect(TimeCurrent() - (TimeCurrent() % PeriodSeconds(TimeFrame)), TimeCurrent());
and not like this:
HistorySelect(TimeCurrent() - PeriodSeconds(TimeFrame), TimeCurrent());
Hope this helps.
Andrey.
Hi,
why do you need to do like this:
and not like this:
HistorySelect(TimeCurrent() - PeriodSeconds(TimeFrame), TimeCurrent());
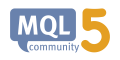
- www.mql5.com
What you have suggested is not the current bar but the time span from current time back the duration of a "TimeFrame" bar, it does not coincide with the start time of the current bar.
Correct. Only have problems on weekly period. Daily and below no problem. Any help?
What you have suggested is not the current bar but the time span from current time back the duration of a "TimeFrame" bar, it does not coincide with the start time of the current bar.
I am not suggesting anything here, I am asking.
It looks to me that this:
TimeCurrent() - (TimeCurrent() % PeriodSeconds(TimeFrame))
returns something very meaningless. Subtracting NUMBER OF WEEKS from SECONDS is like comparing apples and oranges...
The best way to approach this task is simply to find the open time of last weekly candle and select history from that point.
something like
datetime lastweekstart[1]={0}; CopyTime(_Symbol,PERIOD_W1,0,1,lastweekstart); // Plus standard routine to check if all operations are successful HistorySelect(lastweekstart[0],TimeCurrent());
Andrey.
I am not suggesting anything here, I am asking.
It looks to me that this:
returns something very meaningless. Subtracting NUMBER OF WEEKS from SECONDS is like comparing apples and oranges...
The best way to approach this task is simply to find the open time of last weekly candle and select history from that point.
something like
Andrey.
He doesn't subtract NUMBER OF WEEKS from SECONDS. He subtract seconds from a datetime (which is seconds too). So the approach is good, except the week doesn't start when he expect it. (see my post above).
Anyway your method is good and clearer, and can be generalized to :
datetime lastperiodstart[1]={0}; CopyTime(_Symbol,TimeFrame,0,1,lastperiodstart); // Plus standard routine to check if all operations are successful HistorySelect(lastperiodstart[0],TimeCurrent());
Because the 01.01.1970 is a Thursday, so with your method every week start on Thursday 00:00.
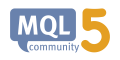
- www.mql5.com
Thanks for all the help. The code works like a charm now.
//+------------------------------------------------------------------+ //| Trade Count | //+------------------------------------------------------------------+ int TradeCount(ENUM_TIMEFRAMES TimeFrame) { //--- int Cnt; ulong Ticket; long EntryType; datetime DT[1]; Cnt = 0; if(CopyTime(Symbol(), TimeFrame, 0, 1, DT) <= 0) { Cnt = -1; } else { HistorySelect(DT[0], TimeCurrent()); for(int i = HistoryDealsTotal() - 1; i >= 0; i--) { Ticket = HistoryDealGetTicket(i); EntryType = HistoryDealGetInteger(Ticket, DEAL_ENTRY); if(EntryType == DEAL_ENTRY_IN || DEAL_ENTRY_INOUT) if(Symbol() == HistoryDealGetString(Ticket, DEAL_SYMBOL)) { Cnt++; } } } //--- return(Cnt); }
This is the code.
Is it optimized?
Thanks for all the help. The code works like a charm now.
This is the code.
Is it optimized?
Optimized for what ?

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
this is my code to trade one trade per bar
it works fine for PERIOD_D1 and below
but when I use it for PERIOD_W1, it is not working properly. any idea why?
usage like this