Found them!
OP_BUY | 0 | Buying position. |
OP_SELL | 1 | Selling position. |
OP_BUYLIMIT | 2 | Buy limit pending position. |
OP_SELLLIMIT | 3 | Sell limit pending position. |
OP_BUYSTOP | 4 | Buy stop pending position. |
OP_SELLSTOP | 5 | Sell stop pending position. |
for (int ix = 0; ix < OrdersHistoryTotal(); ix++)
- Means it returns the earliest order's type not the latest. That is if they were in date order, but they're not.
- Since you're selecting history, ALL of the orders will have a non-zero close time.
- Perhaps you should select the last closed order
looking at the earliest orders will never do. This is not coming clear to me so I think I need to study how orders are selected. I think you are suggesting I should use something like this line of code to select the latest order:
(OrderSelect(tickets[0], SELECT_BY_TICKET)) // Last Closed.
looking at the earliest orders will never do. This is not coming clear to me so I think I need to study how orders are selected. I think you are suggesting I should use something like this line of code to select the latest order:
NO! I said it was complicated, and WHR showed how not-complicated it was by making it even more complicated but hiding the code in a function call. In his code the first thing he did was create an array of all possible historic trades, sorted into order.
int tickets[], nTickets = GetHistoryOrderByCloseTime(tickets);
This cryptic code first declares an array of integers, tickets[].
Then it calls the function GetHistoryOrderByCloseTime, which is defined in a post he linked to. This function allocates memory to the ticket array, populates it with historic tickets and sorts them into date order. The zeroth element of the ticket array, ticket[0], then contains the most recent ticket. Then he can select this order by ticket number.
This is a desperately over-complicated way of performing the task, but "simple" if you already have the GetHistoryOrderByCloseTime, and understand it, and understand arrays. I personally don't think it is that helpful to a beginner. You are better off writing your own so you understand it.
Go though the order history, one order at a time and find the most recent by OrderCloseTime. It doesn't matter if you struggle with it. By continually hunting through all the available commands you begin to learn them without actually sitting down and trying to memorize them. Programming is all about doing "homework" questions. What function do you need to use to do this function? Read them all until you find one that is useful. You don't need to do this very often before you find that you remember, "ah I have seen a function that does that", and your speed increases. I wasn't born knowing MQL4. I have been through the commands list probably hundreds of times, in each little area (Objects, String functions, Trade Functions etc)
Thanks for the push dabbler. Here is the code I came up with -- it seems to work. It was a good learning experience.
//---- Find the OrderType of the last closed trade. ---- int LastOrderType() { datetime TicketTime = 0; int TicketN, LastOT; for (int ix = 0; ix < OrdersHistoryTotal(); ix++) { if(OrderSelect(ix, SELECT_BY_POS, MODE_HISTORY) && OrderMagicNumber() == Magic && OrderSymbol() == Symbol()) { if (OrderCloseTime() > TicketTime) { TicketTime = OrderCloseTime(); //For testing TicketN = OrderTicket(); //For testing LastOT = OrderType(); } } else Print("OrderSelect returned the error of ",GetLastError()); } Print("Last closed order: ",TicketN," ",TicketTime," ",LastOT); //For testing return(LastOT); // Buy==0, Sell==1, Others==2 through 5 }// End Last Order Type
- That should work. It would be slightly more efficient if you counted down - Get in the habit.
- You could combine the if magic/symbol with the if date. Put the date first, string compare last (symbol.)
Thanks for the push dabbler. Here is the code I came up with -- it seems to work. It was a good learning experience.
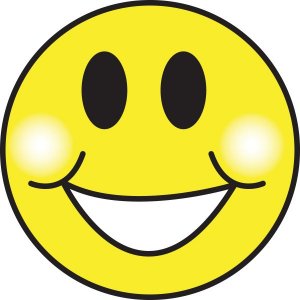
Forgive my noobiness but I would love some help with this very issue.
I am wanting to utilize the above code to make my EA create an order when the opposite order type was just made, or if there was no previous order at all. The logic sort of looks like:
If last order type = NONE or SELL
Buy Condition = True
If last order type = NONE or BUY
Sell Condition = True
I have pasted the above code to the bottom of my EA, but I am unclear about how to utilize it where my BUY/SELL conditions are. I'm assuming I need a line of code referencing the "int LastOrderType()" before my buy and sell condition lines, but what do I put? Thank you very much for the help.
int lastType = LastOrderType(); bool isBuyCondition = lastType != OP_SELL, isSellCondition = lastType != OP_BUY; // hedging EA (not in USA) if (isBuyCondition) .. if (isSellCondition) .. // Non hedging if (isBuyCondition) .. else .. // Not a buy then must be a sell by your conditionHis function returns the last order type. If you need other information about the last order, or about the last N closed orders, then my code would be better: Could EA Really Live By Order_History Alone? - MQL4 forum

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I'm trying to take a lesson from RaptorUK and his article, What are Function return values ? How do I use them ? I assume that an OrderType has a numeric return value; for example OP_BUY is 1 and OP_SELL is 2. But I'm not sure that's the case and I don't see it documented anywhere. Here's the Function I'm trying to use in order to determine the OrderType of the last closed trade. Perhaps there's something wrong with my code.
It's been pointed out that this code is not correct -- it does not select the latest order.