The program at the link has good features with structures.
But not relevant to my application.
The program at the link is writing and reading a file "Casting.tmp". I asked to transform one double to 8 bytes without FileOpen. It's the same way, I think.
The program at the link has good features with structures.
But not relevant to my application.
The program at the link is writing and reading a file "Casting.tmp". I asked to transform one double to 8 bytes without FileOpen. It's the same way, I think.
Are you kidding? The link is for the library, which you can use as you want. Example code on the page shows you how you can convert many different types to bytes, forward and back. No file operations at all.
Are you kidding? The link is for the library, which you can use as you want. Example code on the page shows you how you can convert many different types to bytes, forward and back. No file operations at all.
ok, i debugged the example again.
Again, for every printed example (regarding this thread, _R(Tick).Bytes[8] is the first byte for a double), it is opening "Casting.tmp", write, read, return value.
Of course it's just me who's debugging this way.
ok, i debugged the example again.
Again, for every printed example (regarding this thread, _R(Tick).Bytes[8] is the first byte for a double), it is opening "Casting.tmp", write, read, return value.
Of course it's just me who's debugging this way.
Just don't use TYPETOBYTES_FULL_SLOW declaration.
PS. The file routine is a new feature forcedly introduces after some crucial changes (limitations) in MQL itself.
In stdlib there is a function called IntegerToHexString that converts an integer to a hexadecimal string.
However I need a function that converts a double in MQL to a binary or hexadecimal string based on the IEEE-754 format.
The procedure is outlined here: IEEE 754 Standard for Floating Point Representation of Real NumbersHas anyone implemented the algorithm in MQL already?
Alternatively, is there a way to convert a double to a byte array? I know that there is no byte data type in MQL, but an array of integers with the values of the bytes (8 bits each) would do.
//+------------------------------------------------------------------+ //| Union to get binary/hexa IEEE754 representation of a double | //+------------------------------------------------------------------+ union IEEE754_64 { double value; uchar bytes[8]; //+------------------------------------------------------------------+ //| Convert uchar array into hex string | //+------------------------------------------------------------------+ string BytesToString(void) { string res="0x"; for(int i=7;i>=0;i--) { StringAdd(res,StringFormat("%.2X",(int)bytes[i])); } return(res); } };
Test code :
IEEE754_64 test={1.2345}; printf("Double value %s has IEEE754 hexadecimal representation %s",(string)test.value,test.BytesToString());
Output :
Double value 1.2345 has IEEE754 hexadecimal representation 0x3FF3C083126E978D
This
union
solution is the answer:
"Union is a special data type consisting of several variables sharing the same memory area."
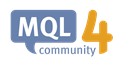
- docs.mql4.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
In stdlib there is a function called IntegerToHexString that converts an integer to a hexadecimal string.
However I need a function that converts a double in MQL to a binary or hexadecimal string based on the IEEE-754 format.
The procedure is outlined here: IEEE 754 Standard for Floating Point Representation of Real NumbersHas anyone implemented the algorithm in MQL already?
Alternatively, is there a way to convert a double to a byte array? I know that there is no byte data type in MQL, but an array of integers with the values of the bytes (8 bits each) would do.