不确定是你故意省略还是怎么的,目前有这几个地方可能存在问题
bool ma_upward = (price
// 根据条件选择平仓
for (int i = 0;
// 交易函数
void Trade()
{
Trade() 之前需要一个 }
这些不确定是你复制出错还是本身就缺少的
不确定是你故意省略还是怎么的,目前有这几个地方可能存在问题
bool ma_upward = (price
// 根据条件选择平仓
for (int i = 0;
// 交易函数
void Trade()
{
Trade() 之前需要一个 }
这些不确定是你复制出错还是本身就缺少的
for (int i = 0; i < OrdersTotal(); i++)
{
if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
if (OrderMagicNumber() == magic_number)
{
if (OrderType() == OP_BUY)
{
if (ma_upward || !in_bollinger)
{
OrderClose(OrderTicket(), OrderLots(), Bid, slippage, Red);
total_positions--;
position_direction--;
}
}
else if (OrderType() == OP_SELL)
{
if (ma_downward || !in_bollinger)
{
OrderClose(OrderTicket(), OrderLots(), Ask, slippage, Red);
total_positions--;
position_direction++;
}
}
}
}
}
第三个问题我还是不明白括号哪里少了 而且这三个错误提示还在......咋解决呀 大佬 能否请教一下 18078119785(微信同号)
具体代码如下:
// 定义参数
input double lot_size = 0.1; // 交易量
input double slippage = 3.0; // 滑点
input double stop_loss = 50; // 止损
input double take_profit = 100; // 止盈
input int magic_number = 123456; // 魔术数字
// 初始化全局变量
int total_positions = 0; // 持仓总数量
int position_direction = 0; // 持仓方向
// 定义均线指标参数
input int ma_period = 20; // 均线周期
// 定义MACD指标参数
input int fast_ema_period = 12; // 快速EMA周期
input int slow_ema_period = 26; // 慢速EMA周期
input int signal_period = 9; // 信号EMA周期
// 定义RSI指标参数
input int rsi_period = 14; // RSI周期
input double overbought_level = 70; // 超买水平
input double oversold_level = 30; // 超卖水平
// 定义布林带指标参数
input int bollinger_period = 20; // 布林带周期
input double deviation = 2.0; // 偏差
// 初始化指标变量
int ma_handle, macd_handle, rsi_handle, bollinger_handle;
// 初始化函数
void OnInit()
{
// 创建指标
ma_handle = iMA(_Symbol, _Period, ma_period, 0, MODE_SMA, PRICE_CLOSE);
macd_handle = iMACD(_Symbol, _Period, fast_ema_period, slow_ema_period, signal_period, PRICE_CLOSE);
rsi_handle = iRSI(_Symbol, _Period, rsi_period, PRICE_CLOSE);
bollinger_handle = iBands(_Symbol, _Period, bollinger_period, deviation, 0, PRICE_CLOSE);
}
// 开始交易函数
void OnTick()
{
// 计算当前价格和指标值
double price = SymbolInfoDouble(_Symbol, SYMBOL_ASK);
double ma = iMA(_Symbol, _Period, ma_period, 0, MODE_SMA, PRICE_CLOSE);
double macd = iMACD(_Symbol, _Period, fast_ema_period, slow_ema_period, signal_period, PRICE_CLOSE, MODE_MAIN, 0);
double rsi = iRSI(_Symbol, _Period, rsi_period, PRICE_CLOSE, 0);
double bollinger_upper = iBands(_Symbol, _Period, bollinger_period, deviation, 0, PRICE_CLOSE, MODE_UPPER, 0);
double bollinger_lower = iBands(_Symbol, _Period, bollinger_period, deviation, 0, PRICE_CLOSE, MODE_LOWER, 0);
bool ma_upward = (price
// 开始主循环
void OnTick()
{
double price = MarketInfo(_Symbol, MODE_BID);
double atr = iATR(_Symbol, _Period, 14, 0);
double sma = iMA(_Symbol, _Period, ma_period, 0, MODE_SMA, PRICE_CLOSE);
double upper_bollinger, lower_bollinger;
iBands(_Symbol, _Period, bollinger_period, bollinger_deviation, 0, 0, upper_bollinger, sma, lower_bollinger);
// 计算MACD指标
double macd, signal, hist;
iMACD(_Symbol, _Period, macd_fast, macd_slow, macd_signal, PRICE_CLOSE, MODE_MAIN, macd, signal, hist);
// 计算RSI指标
double rsi = iRSI(_Symbol, _Period, rsi_period, PRICE_CLOSE, 0);
// 获取持仓方向和持仓数量
int position_direction = 0;
int total_positions = 0;
for (int i = 0; i < OrdersTotal(); i++)
{
if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
if (OrderSymbol() == _Symbol && OrderMagicNumber() == magic_number && OrderType() <= OP_SELL)
{
total_positions += OrderLots() * (OrderType() == OP_BUY ? 1 : -1);
position_direction += (OrderType() == OP_BUY ? 1 : -1);
}
}
}
// 检查是否需要平仓
CheckCloseOrders(price, atr, sma, upper_bollinger, lower_bollinger, macd, signal, rsi, position_direction, total_positions);
// 检查是否需要开仓
CheckOpenOrders(price, atr, sma, upper_bollinger, lower_bollinger, macd, signal, rsi, position_direction, total_positions);
}
// 检查是否需要平仓
void CheckCloseOrders(double price, double atr, double sma, double upper_bollinger, double lower_bollinger, double macd, double signal, double rsi, int position_direction, int total_positions)
{
for (int i = 0; i < OrdersTotal(); i++)
{
if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
if (OrderSymbol() == _Symbol && OrderMagicNumber() == magic_number && OrderType() <= OP_SELL)
{
double order_open_price = OrderOpenPrice();
int order_type = OrderType();
// 如果价格穿越SMA,则平掉订单
if ((order_type == OP_BUY && price < sma) || (order_type == OP_SELL && price > sma))
{
double lots = OrderLots();
double close_price = (order_type == OP_BUY ? Bid : Ask);
int ticket = OrderTicket();
bool res = CloseOrder(ticket, lots, close_price, slippage);
if (res)
{
total_positions--;
position_direction -= (order_type == OP_BUY ? 1 : -1);
}
}
// 如果价格突破布林带,则平掉订单
if ((order_type == OP_BUY && price < lower_bollinger)
// 关闭订单函数
bool CloseOrder(int ticket, double lots, double price, int slippage)
{
bool res = OrderClose(ticket, lots, price, slippage, Black);
return res;
}
// 主函数
void OnTick()
{
// 获取当前K线上的价格信息和指标信息
double price = SymbolInfoDouble(_Symbol, SYMBOL_BID);
double sma = iMA(_Symbol, _Period, ma_period, 0, MODE_SMA, PRICE_CLOSE, 0);
double macd = iMACD(_Symbol, _Period, 12, 26, 9, PRICE_CLOSE, MODE_MAIN, 0);
double rsi = iRSI(_Symbol, _Period, rsi_period, PRICE_CLOSE, 0);
double atr = iATR(_Symbol, _Period, atr_period, 0);
double bollinger_upper = iBands(_Symbol, _Period, bollinger_period, 2, 0, PRICE_CLOSE, MODE_UPPER, 0);
double bollinger_lower = iBands(_Symbol, _Period, bollinger_period, 2, 0, PRICE_CLOSE, MODE_LOWER, 0);
// 判断当前是否有持仓
if (total_positions > 0)
{
// 遍历所有持仓订单
for (int i = 0; i < OrdersTotal(); i++)
{
if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
// 如果订单是本EA开仓的
if (OrderMagicNumber() == magic_number)
{
// 如果订单是买单并且价格跌破SMA线
if (OrderType() == OP_BUY && price < sma)
{
CloseOrder(OrderTicket(), OrderLots(), Bid, slippage);
}
// 如果订单是卖单并且价格上涨超过SMA线
else if (OrderType() == OP_SELL && price > sma)
{
CloseOrder(OrderTicket(), OrderLots(), Ask, slippage);
}
}
}
}
}
else
{
// 判断是否符合均值回归策略的开仓条件
bool ma_upward = (price > sma);
bool ma_downward = (price < sma);
bool macd_upward = (macd > 0);
bool macd_downward = (macd < 0);
bool rsi_upward = (rsi > 50);
bool rsi_downward = (rsi < 50);
bool in_bollinger = (price < bollinger_upper && price > bollinger_lower);
if (ma_downward && macd_downward && rsi_downward && in_bollinger)
{
int ticket = Buy(_Symbol, volume, Ask, slippage, 0, 0, 0, "Open Long - Mean Reversion", magic_number);
if (ticket > 0)
{
total_positions++;
position_direction++;
}
}
else if (ma_upward && !macd_upward && !rsi_upward && in_bollinger)
{
int ticket = Sell(_Symbol, volume, Bid, slippage, 0, 0, 0, "Open Short - Volatility", magic_number);
if (
int OnInit()
{
// 设置指标和时间框架
IndicatorSetInteger(INDICATOR_DIGITS, _Digits);
IndicatorSetInteger(INDICATOR_TIMEFRAME, PERIOD_CURRENT);
// 计算波动性
double atr = iATR(_Symbol, _Period, 14, 0);
// 计算布林带指标
double boll_upper, boll_lower;
iBands(_Symbol, _Period, 20, 2, 0, PRICE_CLOSE, boll_upper, boll_lower);
return(INIT_SUCCEEDED);
}
void OnTick()
{
double price = SymbolInfoDouble(_Symbol, SYMBOL_BID);
// 计算均线
double ma = iMA(_Symbol, _Period, 20, 0, MODE_SMA, PRICE_CLOSE);
// 计算MACD指标
double macd, macd_signal, macd_histogram;
iMACD(_Symbol, _Period, 12, 26, 9, PRICE_CLOSE, macd, macd_signal, macd_histogram);
bool macd_upward = (macd > macd_signal);
// 计算RSI指标
double rsi = iRSI(_Symbol, _Period, 14, PRICE_CLOSE, 0);
bool rsi_upward = (rsi > 50);
// 判断是否处于布林带内
bool in_bollinger = (price > boll_lower && price < boll_upper);
// 判断是否处于均线上方
bool ma_upward = (price > ma);
// 判断是否需要开仓
if (!OrdersTotal())
{
// 根据条件选择开仓
if (price > boll_upper && macd_upward && rsi_upward)
{
int ticket = Buy(_Symbol, volume, Ask, slippage, 0, 0, 0, "Open Long - Volatility and Trend", magic_number);
if (ticket > 0)
{
total_positions++;
position_direction++;
}
}
else if (price < boll_lower && !macd_upward && rsi_upward)
{
int ticket = Sell(_Symbol, volume, Bid, slippage, 0, 0, 0, "Open Short - Volatility and Trend", magic_number);
if (ticket > 0)
{
total_positions++;
position_direction--;
}
}
else if (ma_upward && !macd_upward && !rsi_upward && in_bollinger)
{
int ticket = Sell(_Symbol, volume, Bid, slippage, 0, 0, 0, "Open Short - Mean Reversion", magic_number);
if (ticket > 0)
{
total_positions++;
position_direction--;
}
}
else if (!ma_upward && !macd_upward && !rsi_upward && in_bollinger)
{
int ticket = Buy(_Symbol, volume, Ask, slippage, 0, 0, 0, "Open Long - Mean Reversion", magic_number);
if (ticket > 0)
{
total_positions++;
position_direction++;
}
}
}
else
{
// 根据条件选择平仓
for (int i = 0;
// 判断是否在布林通道内
bool InBollinger(double price, double bollinger_upper, double bollinger_lower)
{
return (price < bollinger_upper) && (price > bollinger_lower);
}
// 交易函数
void Trade()
{
// 获取当前价格
double price = MarketInfo(_Symbol, MODE_BID);
// 计算均线、MACD、RSI、布林带、ATR等指标
double ma = iMA(_Symbol, _Period, ma_period, 0, ma_method, PRICE_CLOSE, 0);
double macd = iMACD(_Symbol, _Period, macd_fast_period, macd_slow_period, macd_signal_period, PRICE_CLOSE, MODE_MAIN, 0);
double rsi = iRSI(_Symbol, _Period, rsi_period, PRICE_CLOSE, 0);
double bollinger_upper = iBands(_Symbol, _Period, bollinger_period, bollinger_deviation, 0, PRICE_CLOSE, MODE_UPPER, 0);
double bollinger_lower = iBands(_Symbol, _Period, bollinger_period, bollinger_deviation, 0, PRICE_CLOSE, MODE_LOWER, 0);
double atr = iATR(_Symbol, _Period, atr_period, 0);
// 判断价格趋势
bool price_upward = price > ma;
bool price_downward = price < ma;
// 判断MACD指标趋势
bool macd_upward = macd > 0;
bool macd_downward = macd < 0;
// 判断RSI指标趋势
bool rsi_upward = rsi > rsi_buy_level;
bool rsi_downward = rsi < rsi_sell_level;
// 判断是否在布林带内
bool in_bollinger = InBollinger(price, bollinger_upper, bollinger_lower);
// 判断是否在波动性策略执行时间范围内
bool in_volatility_time = (Hour() >= volatility_start_hour) && (Hour() <= volatility_end_hour);
// 判断是否在均值回归策略执行时间范围内
bool in_mean_reversion_time = (Hour() >= mean_reversion_start_hour) && (Hour() <= mean_reversion_end_hour);
// 计算持仓数量
int total_positions = PositionsTotal();
int position_direction = 0;
for (int i = 0; i < total_positions; i++)
{
// 获取订单信息
ulong ticket = PositionGetTicket(i);
double lots = PositionGetDouble(POSITION_VOLUME);
double open_price = PositionGetDouble(POSITION_PRICE_OPEN);
// 判断订单持仓方向
if (PositionGetInteger(POSITION_TYPE) == POSITION_TYPE_BUY)
{
position_direction++;
}
else
{
position_direction--;
}
// 计算当前订单浮动盈亏
double profit = PositionGetDouble(POSITION_PROFIT);
// 判断是否平掉订单
if (profit > take_profit)
{
CloseOrder(ticket, lots, Bid, slippage);
}
else if (profit < stop_loss)
{
CloseOrder(ticket, lots, Ask, slippage);
}
}
// 执行交易
编译器报错如下:
'}' - unexpected end of program 343 1 我删除或增加括号也还是报错
'{' - unbalanced parentheses 44 1 我删除或增加括号也还是报错
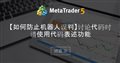
- 2023.06.13
- www.mql5.com
具体代码如下:
// 定义参数
input double lot_size = 0.1; // 交易量
input double slippage = 3.0; // 滑点
input double stop_loss = 50; // 止损
input double take_profit = 100; // 止盈
input int magic_number = 123456; // 魔术数字
// 初始化全局变量
int total_positions = 0; // 持仓总数量
int position_direction = 0; // 持仓方向
// 定义均线指标参数
input int ma_period = 20; // 均线周期
// 定义MACD指标参数
input int fast_ema_period = 12; // 快速EMA周期
input int slow_ema_period = 26; // 慢速EMA周期
input int signal_period = 9; // 信号EMA周期
// 定义RSI指标参数
input int rsi_period = 14; // RSI周期
input double overbought_level = 70; // 超买水平
input double oversold_level = 30; // 超卖水平
// 定义布林带指标参数
input int bollinger_period = 20; // 布林带周期
input double deviation = 2.0; // 偏差
// 初始化指标变量
int ma_handle, macd_handle, rsi_handle, bollinger_handle;
// 初始化函数
void OnInit()
{
// 创建指标
ma_handle = iMA(_Symbol, _Period, ma_period, 0, MODE_SMA, PRICE_CLOSE);
macd_handle = iMACD(_Symbol, _Period, fast_ema_period, slow_ema_period, signal_period, PRICE_CLOSE);
rsi_handle = iRSI(_Symbol, _Period, rsi_period, PRICE_CLOSE);
bollinger_handle = iBands(_Symbol, _Period, bollinger_period, deviation, 0, PRICE_CLOSE);
}
// 开始交易函数
void OnTick()
{
// 计算当前价格和指标值
double price = SymbolInfoDouble(_Symbol, SYMBOL_ASK);
double ma = iMA(_Symbol, _Period, ma_period, 0, MODE_SMA, PRICE_CLOSE);
double macd = iMACD(_Symbol, _Period, fast_ema_period, slow_ema_period, signal_period, PRICE_CLOSE, MODE_MAIN, 0);
double rsi = iRSI(_Symbol, _Period, rsi_period, PRICE_CLOSE, 0);
double bollinger_upper = iBands(_Symbol, _Period, bollinger_period, deviation, 0, PRICE_CLOSE, MODE_UPPER, 0);
double bollinger_lower = iBands(_Symbol, _Period, bollinger_period, deviation, 0, PRICE_CLOSE, MODE_LOWER, 0);
bool ma_upward = (price
// 开始主循环
void OnTick()
{
double price = MarketInfo(_Symbol, MODE_BID);
double atr = iATR(_Symbol, _Period, 14, 0);
double sma = iMA(_Symbol, _Period, ma_period, 0, MODE_SMA, PRICE_CLOSE);
double upper_bollinger, lower_bollinger;
iBands(_Symbol, _Period, bollinger_period, bollinger_deviation, 0, 0, upper_bollinger, sma, lower_bollinger);
// 计算MACD指标
double macd, signal, hist;
iMACD(_Symbol, _Period, macd_fast, macd_slow, macd_signal, PRICE_CLOSE, MODE_MAIN, macd, signal, hist);
// 计算RSI指标
double rsi = iRSI(_Symbol, _Period, rsi_period, PRICE_CLOSE, 0);
// 获取持仓方向和持仓数量
int position_direction = 0;
int total_positions = 0;
for (int i = 0; i < OrdersTotal(); i++)
{
if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
if (OrderSymbol() == _Symbol && OrderMagicNumber() == magic_number && OrderType() <= OP_SELL)
{
total_positions += OrderLots() * (OrderType() == OP_BUY ? 1 : -1);
position_direction += (OrderType() == OP_BUY ? 1 : -1);
}
}
}
// 检查是否需要平仓
CheckCloseOrders(price, atr, sma, upper_bollinger, lower_bollinger, macd, signal, rsi, position_direction, total_positions);
// 检查是否需要开仓
CheckOpenOrders(price, atr, sma, upper_bollinger, lower_bollinger, macd, signal, rsi, position_direction, total_positions);
}
// 检查是否需要平仓
void CheckCloseOrders(double price, double atr, double sma, double upper_bollinger, double lower_bollinger, double macd, double signal, double rsi, int position_direction, int total_positions)
{
for (int i = 0; i < OrdersTotal(); i++)
{
if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
if (OrderSymbol() == _Symbol && OrderMagicNumber() == magic_number && OrderType() <= OP_SELL)
{
double order_open_price = OrderOpenPrice();
int order_type = OrderType();
// 如果价格穿越SMA,则平掉订单
if ((order_type == OP_BUY && price < sma) || (order_type == OP_SELL && price > sma))
{
double lots = OrderLots();
double close_price = (order_type == OP_BUY ? Bid : Ask);
int ticket = OrderTicket();
bool res = CloseOrder(ticket, lots, close_price, slippage);
if (res)
{
total_positions--;
position_direction -= (order_type == OP_BUY ? 1 : -1);
}
}
// 如果价格突破布林带,则平掉订单
if ((order_type == OP_BUY && price < lower_bollinger)
// 关闭订单函数
bool CloseOrder(int ticket, double lots, double price, int slippage)
{
bool res = OrderClose(ticket, lots, price, slippage, Black);
return res;
}
// 主函数
void OnTick()
{
// 获取当前K线上的价格信息和指标信息
double price = SymbolInfoDouble(_Symbol, SYMBOL_BID);
double sma = iMA(_Symbol, _Period, ma_period, 0, MODE_SMA, PRICE_CLOSE, 0);
double macd = iMACD(_Symbol, _Period, 12, 26, 9, PRICE_CLOSE, MODE_MAIN, 0);
double rsi = iRSI(_Symbol, _Period, rsi_period, PRICE_CLOSE, 0);
double atr = iATR(_Symbol, _Period, atr_period, 0);
double bollinger_upper = iBands(_Symbol, _Period, bollinger_period, 2, 0, PRICE_CLOSE, MODE_UPPER, 0);
double bollinger_lower = iBands(_Symbol, _Period, bollinger_period, 2, 0, PRICE_CLOSE, MODE_LOWER, 0);
// 判断当前是否有持仓
if (total_positions > 0)
{
// 遍历所有持仓订单
for (int i = 0; i < OrdersTotal(); i++)
{
if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
// 如果订单是本EA开仓的
if (OrderMagicNumber() == magic_number)
{
// 如果订单是买单并且价格跌破SMA线
if (OrderType() == OP_BUY && price < sma)
{
CloseOrder(OrderTicket(), OrderLots(), Bid, slippage);
}
// 如果订单是卖单并且价格上涨超过SMA线
else if (OrderType() == OP_SELL && price > sma)
{
CloseOrder(OrderTicket(), OrderLots(), Ask, slippage);
}
}
}
}
}
else
{
// 判断是否符合均值回归策略的开仓条件
bool ma_upward = (price > sma);
bool ma_downward = (price < sma);
bool macd_upward = (macd > 0);
bool macd_downward = (macd < 0);
bool rsi_upward = (rsi > 50);
bool rsi_downward = (rsi < 50);
bool in_bollinger = (price < bollinger_upper && price > bollinger_lower);
if (ma_downward && macd_downward && rsi_downward && in_bollinger)
{
int ticket = Buy(_Symbol, volume, Ask, slippage, 0, 0, 0, "Open Long - Mean Reversion", magic_number);
if (ticket > 0)
{
total_positions++;
position_direction++;
}
}
else if (ma_upward && !macd_upward && !rsi_upward && in_bollinger)
{
int ticket = Sell(_Symbol, volume, Bid, slippage, 0, 0, 0, "Open Short - Volatility", magic_number);
if (
int OnInit()
{
// 设置指标和时间框架
IndicatorSetInteger(INDICATOR_DIGITS, _Digits);
IndicatorSetInteger(INDICATOR_TIMEFRAME, PERIOD_CURRENT);
// 计算波动性
double atr = iATR(_Symbol, _Period, 14, 0);
// 计算布林带指标
double boll_upper, boll_lower;
iBands(_Symbol, _Period, 20, 2, 0, PRICE_CLOSE, boll_upper, boll_lower);
return(INIT_SUCCEEDED);
}
void OnTick()
{
double price = SymbolInfoDouble(_Symbol, SYMBOL_BID);
// 计算均线
double ma = iMA(_Symbol, _Period, 20, 0, MODE_SMA, PRICE_CLOSE);
// 计算MACD指标
double macd, macd_signal, macd_histogram;
iMACD(_Symbol, _Period, 12, 26, 9, PRICE_CLOSE, macd, macd_signal, macd_histogram);
bool macd_upward = (macd > macd_signal);
// 计算RSI指标
double rsi = iRSI(_Symbol, _Period, 14, PRICE_CLOSE, 0);
bool rsi_upward = (rsi > 50);
// 判断是否处于布林带内
bool in_bollinger = (price > boll_lower && price < boll_upper);
// 判断是否处于均线上方
bool ma_upward = (price > ma);
// 判断是否需要开仓
if (!OrdersTotal())
{
// 根据条件选择开仓
if (price > boll_upper && macd_upward && rsi_upward)
{
int ticket = Buy(_Symbol, volume, Ask, slippage, 0, 0, 0, "Open Long - Volatility and Trend", magic_number);
if (ticket > 0)
{
total_positions++;
position_direction++;
}
}
else if (price < boll_lower && !macd_upward && rsi_upward)
{
int ticket = Sell(_Symbol, volume, Bid, slippage, 0, 0, 0, "Open Short - Volatility and Trend", magic_number);
if (ticket > 0)
{
total_positions++;
position_direction--;
}
}
else if (ma_upward && !macd_upward && !rsi_upward && in_bollinger)
{
int ticket = Sell(_Symbol, volume, Bid, slippage, 0, 0, 0, "Open Short - Mean Reversion", magic_number);
if (ticket > 0)
{
total_positions++;
position_direction--;
}
}
else if (!ma_upward && !macd_upward && !rsi_upward && in_bollinger)
{
int ticket = Buy(_Symbol, volume, Ask, slippage, 0, 0, 0, "Open Long - Mean Reversion", magic_number);
if (ticket > 0)
{
total_positions++;
position_direction++;
}
}
}
else
{
// 根据条件选择平仓
for (int i = 0;
// 判断是否在布林通道内
bool InBollinger(double price, double bollinger_upper, double bollinger_lower)
{
return (price < bollinger_upper) && (price > bollinger_lower);
}
// 交易函数
void Trade()
{
// 获取当前价格
double price = MarketInfo(_Symbol, MODE_BID);
// 计算均线、MACD、RSI、布林带、ATR等指标
double ma = iMA(_Symbol, _Period, ma_period, 0, ma_method, PRICE_CLOSE, 0);
double macd = iMACD(_Symbol, _Period, macd_fast_period, macd_slow_period, macd_signal_period, PRICE_CLOSE, MODE_MAIN, 0);
double rsi = iRSI(_Symbol, _Period, rsi_period, PRICE_CLOSE, 0);
double bollinger_upper = iBands(_Symbol, _Period, bollinger_period, bollinger_deviation, 0, PRICE_CLOSE, MODE_UPPER, 0);
double bollinger_lower = iBands(_Symbol, _Period, bollinger_period, bollinger_deviation, 0, PRICE_CLOSE, MODE_LOWER, 0);
double atr = iATR(_Symbol, _Period, atr_period, 0);
// 判断价格趋势
bool price_upward = price > ma;
bool price_downward = price < ma;
// 判断MACD指标趋势
bool macd_upward = macd > 0;
bool macd_downward = macd < 0;
// 判断RSI指标趋势
bool rsi_upward = rsi > rsi_buy_level;
bool rsi_downward = rsi < rsi_sell_level;
// 判断是否在布林带内
bool in_bollinger = InBollinger(price, bollinger_upper, bollinger_lower);
// 判断是否在波动性策略执行时间范围内
bool in_volatility_time = (Hour() >= volatility_start_hour) && (Hour() <= volatility_end_hour);
// 判断是否在均值回归策略执行时间范围内
bool in_mean_reversion_time = (Hour() >= mean_reversion_start_hour) && (Hour() <= mean_reversion_end_hour);
// 计算持仓数量
int total_positions = PositionsTotal();
int position_direction = 0;
for (int i = 0; i < total_positions; i++)
{
// 获取订单信息
ulong ticket = PositionGetTicket(i);
double lots = PositionGetDouble(POSITION_VOLUME);
double open_price = PositionGetDouble(POSITION_PRICE_OPEN);
// 判断订单持仓方向
if (PositionGetInteger(POSITION_TYPE) == POSITION_TYPE_BUY)
{
position_direction++;
}
else
{
position_direction--;
}
// 计算当前订单浮动盈亏
double profit = PositionGetDouble(POSITION_PROFIT);
// 判断是否平掉订单
if (profit > take_profit)
{
CloseOrder(ticket, lots, Bid, slippage);
}
else if (profit < stop_loss)
{
CloseOrder(ticket, lots, Ask, slippage);
}
}
// 执行交易
编译器报错如下:
'}' - unexpected end of program 343 1 我删除或增加括号也还是报错
'{' - unbalanced parentheses 44 1 我删除或增加括号也还是报错