Seems trivial, maybe I misunderstood : with a dot like all methods of objects.
if (arrCQ[iQ].match(id1,id2)) {
...
}
Thank you - at the first glance it seems to be perfect the . instead of :: removes the compiler error - but (sorry) after further google search I found:
". for accessing object member variables and methods via object instance"
and
"-> for accessing object member variables and methods via pointer to object"
so trying to use -> as it s an array of pointer to instances ( CQ* &arrCQ[] ) causes as well a compiler error.
I am not that far to test my example but this raises the question behaves OOP of MQL5 different than C++ (from stackoverflow)?
1.=> -> for accessing object member variables and methods via pointer to object Foo *foo = new Foo(); foo->member_var = 10; foo->member_func(); 2.=> . for accessing object member variables and methods via object instance Foo foo; foo.member_var = 10; foo.member_func(); 3.=> :: for accessing static variables and methods of a class/struct or namespace. It can also be used to access variables and functions from another scope (actually class, struct, namespace are scopes in that case)
Why does -> not work?
Wouldn't I get an error if at runtime the . is used for a pointer?
Any thanks so far from an OOP newbee!
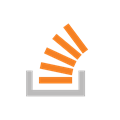
- stackoverflow.com
MQL is not C++. It does not have -> operator. It uses . both for pointers and references.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi,
the problem seems to be easy but I am stuck.
I want to have 2 classes CQ and CI.
CI in its constructor searches within an array of pointers (ArrCQ[]) to existing instances of CQ whether there is one that matches.
For this "match-check" CQ has a method match(..) but how can I call from within the CI-constructor the CQ method match?
This try causes a compiler error: 'match' - function not defined.
In addition to that if within the loop no match have been found a new instance of CQ should be created and the pointer should be inserted into the (increased) array. So I need to call as CQs contructor with the array and th two IDs.