https://www.mql5.com/en/forum/91432/page2#comment_2666762
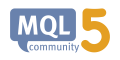
!!! Helping for free by coding of simple mql4(5) task.
- www.mql5.com
You can write tasks here. Screenshots with examples of what do you want are obligated...
This indicator works perfectly when attached to a chart.
However, I got a
"Zero Divide"
error when using it in Expert Advisor.
How do I work around this line to get it to work in EA?
_PipsRatio = (_CurRangeHigh - _CurRangeLow) / (_SubRangeHigh - _SubRangeLow)
Thank you!