Im working on this indicator, it draws swing points..I just need a more efficient way to perfom this task..my code appears messy to me...some times it misses a point.
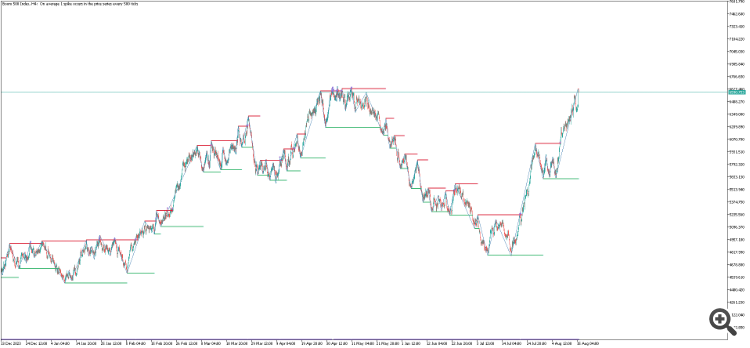
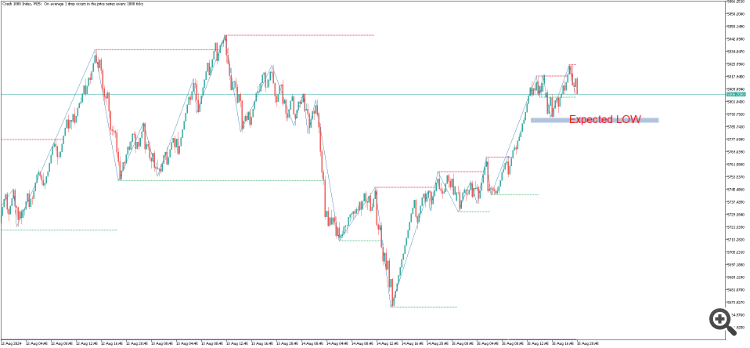
- For Advanced Users - Getting Started
- Moving (swapping) arrays
- Tracking bar formation

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register